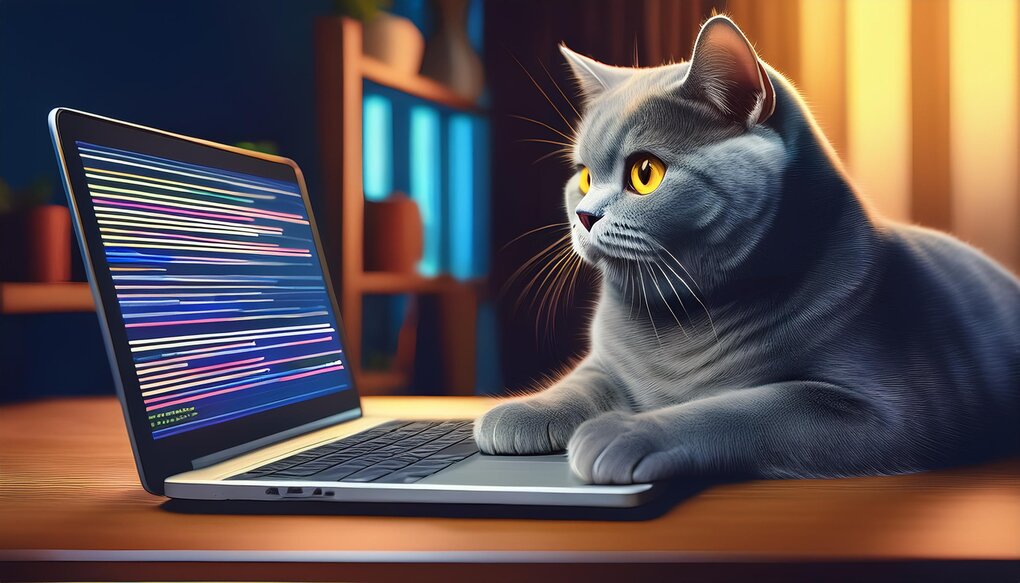
React Content Loader: Create Stunning Loading Skeletons with Ease
React Content Loader is a powerful library that allows you to create beautiful, customizable loading skeletons for your React applications. These skeletons provide a smooth, animated placeholder while your content is loading, improving the perceived performance and user experience of your app.
Installation
To get started with react-content-loader, you’ll need to install it in your project. You can do this using npm or yarn:
# Using npm
npm install react-content-loader
# Using yarn
yarn add react-content-loader
For React Native projects, you’ll also need to install react-native-svg
:
# Using npm
npm install react-content-loader react-native-svg
# Using yarn
yarn add react-content-loader react-native-svg
Basic Usage
React Content Loader comes with several preset loaders that you can use out of the box. Here’s a simple example using the default loader:
import React from 'react';
import ContentLoader from 'react-content-loader';
const MyLoader: React.FC = () => (
<ContentLoader>
{/* Add SVG shapes here */}
</ContentLoader>
);
export default MyLoader;
You can also use pre-built loaders like Facebook or Instagram style:
import React from 'react';
import ContentLoader, { Facebook, Instagram } from 'react-content-loader';
const MyFacebookLoader: React.FC = () => <Facebook />;
const MyInstagramLoader: React.FC = () => <Instagram />;
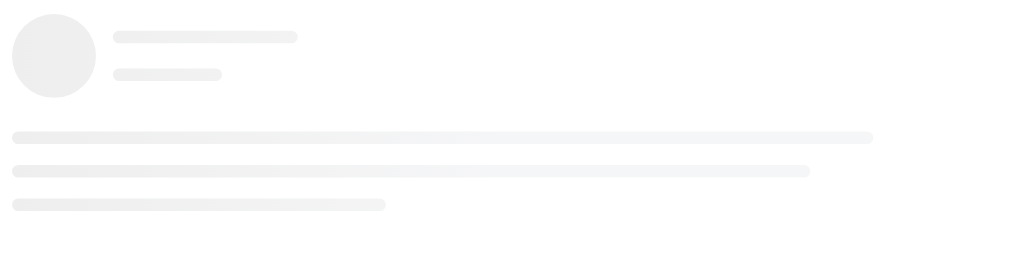
Advanced Usage
For more control over your loading skeleton, you can create custom loaders by adding SVG shapes:
import React from 'react';
import ContentLoader from 'react-content-loader';
const MyCustomLoader: React.FC = () => (
<ContentLoader
speed={2}
width={400}
height={160}
viewBox="0 0 400 160"
backgroundColor="#f3f3f3"
foregroundColor="#ecebeb"
>
<rect x="48" y="8" rx="3" ry="3" width="88" height="6" />
<rect x="48" y="26" rx="3" ry="3" width="52" height="6" />
<rect x="0" y="56" rx="3" ry="3" width="410" height="6" />
<rect x="0" y="72" rx="3" ry="3" width="380" height="6" />
<rect x="0" y="88" rx="3" ry="3" width="178" height="6" />
<circle cx="20" cy="20" r="20" />
</ContentLoader>
);
export default MyCustomLoader;
You can customize various aspects of the loader, such as speed, colors, and dimensions. Here’s an example with some custom properties:
import React from 'react';
import ContentLoader from 'react-content-loader';
const MyResponsiveLoader: React.FC = () => (
<ContentLoader
speed={1}
width={300}
height={200}
viewBox="0 0 300 200"
backgroundColor="#f5f5f5"
foregroundColor="#dbdbdb"
style={{ width: '100%' }}
>
<rect x="0" y="0" rx="5" ry="5" width="70" height="70" />
<rect x="80" y="17" rx="4" ry="4" width="220" height="13" />
<rect x="80" y="40" rx="3" ry="3" width="170" height="10" />
</ContentLoader>
);
export default MyResponsiveLoader;
Conclusion
React Content Loader is a versatile library that can significantly improve the loading experience in your React applications. By using pre-built loaders or creating custom ones, you can provide users with smooth, animated placeholders that make your app feel faster and more responsive.
Remember to experiment with different shapes, sizes, and animations to find the perfect loading skeleton for your specific use case. With a little creativity, you can create loading states that not only serve a functional purpose but also enhance the overall design of your application.