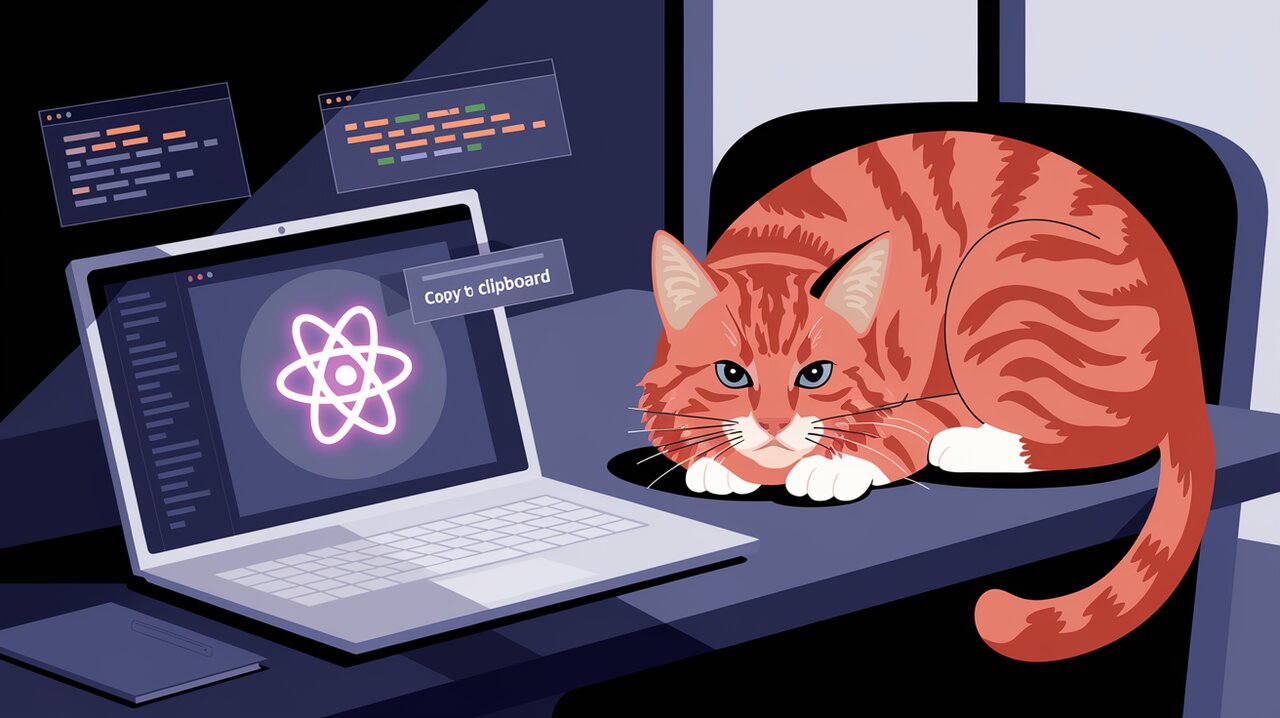
React applications often require the ability to copy text to the clipboard, enhancing user experience and facilitating easy sharing of content. The react-copy-to-clipboard library simplifies this process, providing a straightforward way to implement clipboard functionality in your React projects. Let’s dive into how you can leverage this powerful tool to add seamless copy operations to your applications.
Features
The react-copy-to-clipboard
library offers several key features that make it a valuable addition to any React developer’s toolkit:
- Simple integration with React components
- Cross-browser compatibility
- Customizable copy behavior
- Event callbacks for successful copy operations
- Support for copying text content of any length
Installation
To get started with react-copy-to-clipboard
, you’ll need to install it in your project. You can do this using npm or yarn.
Using npm:
npm install --save react-copy-to-clipboard
Using yarn:
yarn add react-copy-to-clipboard
Basic Usage
Once installed, you can start using the CopyToClipboard
component in your React application. Here’s a simple example of how to implement a copy-to-clipboard button:
import React, { useState } from 'react';
import { CopyToClipboard } from 'react-copy-to-clipboard';
const CopyButton: React.FC = () => {
const [copied, setCopied] = useState(false);
const textToCopy = "Hello, this is the text to be copied!";
return (
<div>
<input value={textToCopy} readOnly />
<CopyToClipboard text={textToCopy} onCopy={() => setCopied(true)}>
<button>{copied ? "Copied!" : "Copy to clipboard"}</button>
</CopyToClipboard>
</div>
);
};
export default CopyButton;
In this example, we create a simple component with an input field containing the text to be copied and a button that triggers the copy action. The CopyToClipboard
component wraps the button, providing it with the copy functionality.
Advanced Usage
Customizing Copy Behavior
The CopyToClipboard
component accepts an options
prop that allows you to customize its behavior. For instance, you can enable debug mode or set a custom message:
import React from 'react';
import { CopyToClipboard } from 'react-copy-to-clipboard';
const AdvancedCopyButton: React.FC = () => {
const textToCopy = "This is some advanced copy text";
return (
<CopyToClipboard
text={textToCopy}
onCopy={(text, result) => console.log(`Copied ${text}. Success: ${result}`)}
options={{
debug: true,
message: 'Press #{key} to copy'
}}
>
<button>Copy with custom options</button>
</CopyToClipboard>
);
};
export default AdvancedCopyButton;
This example enables debug mode and sets a custom message for browsers that don’t support programmatic copy.
Handling Copy Events
The onCopy
prop allows you to handle successful copy events. You can use this to provide user feedback or perform additional actions:
import React, { useState } from 'react';
import { CopyToClipboard } from 'react-copy-to-clipboard';
const CopyWithFeedback: React.FC = () => {
const [feedback, setFeedback] = useState('');
const textToCopy = "Copy me and see what happens!";
const handleCopy = (text: string, result: boolean) => {
if (result) {
setFeedback('Successfully copied!');
setTimeout(() => setFeedback(''), 2000);
} else {
setFeedback('Failed to copy. Please try again.');
}
};
return (
<div>
<CopyToClipboard text={textToCopy} onCopy={handleCopy}>
<button>Copy with feedback</button>
</CopyToClipboard>
{feedback && <p>{feedback}</p>}
</div>
);
};
export default CopyWithFeedback;
This component provides visual feedback to the user after a copy attempt, displaying a success or failure message.
Copying Dynamic Content
You can also use react-copy-to-clipboard with dynamic content, such as user input:
import React, { useState } from 'react';
import { CopyToClipboard } from 'react-copy-to-clipboard';
const DynamicCopy: React.FC = () => {
const [text, setText] = useState('');
return (
<div>
<input
value={text}
onChange={(e) => setText(e.target.value)}
placeholder="Enter text to copy"
/>
<CopyToClipboard text={text} onCopy={() => alert('Copied!')}>
<button>Copy dynamic text</button>
</CopyToClipboard>
</div>
);
};
export default DynamicCopy;
This example allows users to input their own text and copy it to the clipboard.
Conclusion
The react-copy-to-clipboard
library provides a simple yet powerful way to add clipboard functionality to your React applications. By leveraging its easy-to-use components and customizable options, you can enhance user experience and streamline content sharing in your projects. Whether you need basic copy functionality or more advanced features like custom feedback and dynamic content copying, this library has you covered. Implement these examples in your next React project and watch as your users enjoy the seamless copy-to-clipboard experience!