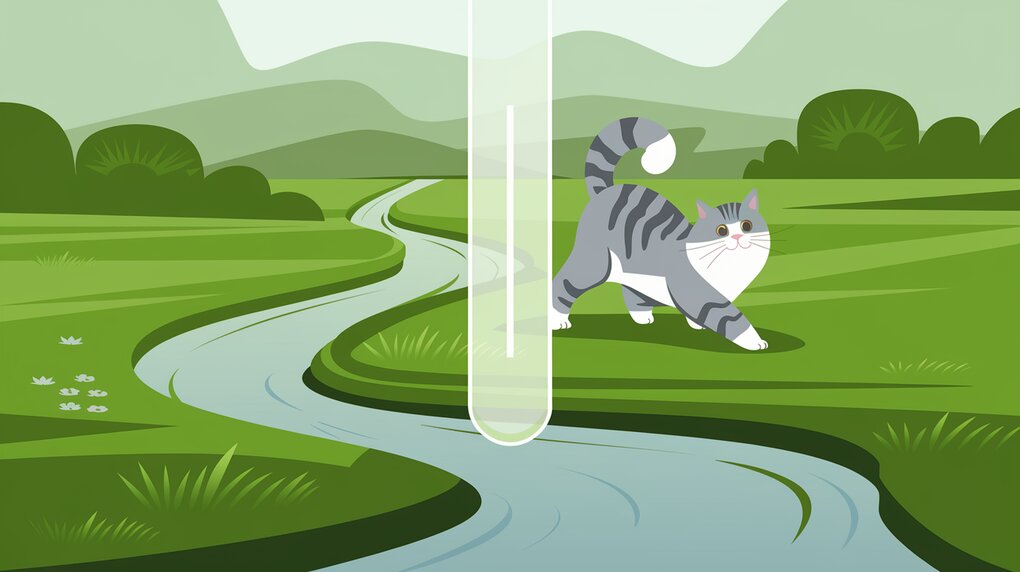
Custom Scrollbars with React: A Comprehensive Guide
Introduction
Custom scrollbars in React can significantly enhance the user experience by providing a tailored and interactive interface. The react-custom-scrollbars
library offers a comprehensive solution for creating custom scrollbars that are fully customizable, frictionless, and performant. In this article, we will explore the features, installation, basic usage, and advanced customization options of this library.
Features
The react-custom-scrollbars
library boasts several key features that make it a powerful tool for developers:
- Frictionless Native Browser Scrolling: The library ensures that scrolling is handled natively by the browser, providing a seamless and smooth experience.
- Native Scrollbars for Mobile Devices: The scrollbars are optimized for mobile devices, ensuring that they function correctly and efficiently on these platforms.
- Fully Customizable: The scrollbars can be customized extensively, allowing developers to tailor them to their specific needs.
- Auto Hide: The scrollbars can be automatically hidden when not in use, enhancing the overall user experience.
- Auto Height: The scrollbars can adjust their height dynamically based on the content, providing a clean and organized interface.
- Universal Rendering: The library supports rendering on both client and server sides, making it versatile for various application scenarios.
- High Performance: The library uses
requestAnimationFrame
for 60fps rendering, ensuring that the scrollbars update synchronously with the browser’s render flow. - No Extra Stylesheets: The library does not require additional stylesheets, allowing developers to style the scrollbars using their existing CSS.
Installation
To install the react-custom-scrollbars
library, you can use npm or yarn:
npm install react-custom-scrollbars --save
Alternatively, if you prefer a single-file UMD build, you can grab a pre-built version from unpkg:
npm install react-custom-scrollbars@4.2.1
Basic Usage
The basic usage of the react-custom-scrollbars
library is straightforward. Here is a simple example:
import { Scrollbars } from 'react-custom-scrollbars';
class App extends Component {
render() {
return (
<Scrollbars style={{ width: 500, height: 300 }}>
<p>Some great content...</p>
</Scrollbars>
);
}
}
In this example, we import the Scrollbars
component and use it to wrap a piece of content. The style
prop is used to set the width and height of the scrollbars.
Advanced Usage
The react-custom-scrollbars
library offers a wide range of props that can be used to customize the scrollbars. Here is an example of advanced usage:
import { Scrollbars } from 'react-custom-scrollbars';
class CustomScrollbars extends Component {
render() {
return (
<Scrollbars
onScroll={this.handleScroll}
onScrollFrame={this.handleScrollFrame}
onScrollStart={this.handleScrollStart}
onScrollStop={this.handleScrollStop}
onUpdate={this.handleUpdate}
renderView={this.renderView}
renderTrackHorizontal={this.renderTrackHorizontal}
renderTrackVertical={this.renderTrackVertical}
renderThumbHorizontal={this.renderThumbHorizontal}
renderThumbVertical={this.renderThumbVertical}
autoHide
autoHideTimeout={1000}
autoHideDuration={200}
autoHeight
autoHeightMin={0}
autoHeightMax={200}
thumbMinSize={30}
universal={true}
{...this.props}>
</Scrollbars>
);
}
}
In this example, we define a custom CustomScrollbars
component that uses various props to customize the scrollbars. These props include event handlers for scrolling, rendering functions for the scrollbar components, and options for auto hide, auto height, and thumb size.
Conclusion
The react-custom-scrollbars
library is a powerful tool for creating custom scrollbars in React. With its frictionless native scrolling, native support for mobile devices, and extensive customization options, it is an excellent choice for developers looking to enhance their applications with custom scrollbars. By following the basic and advanced usage examples provided in this article, you can start creating your own custom scrollbars and enhancing the user experience of your React applications.