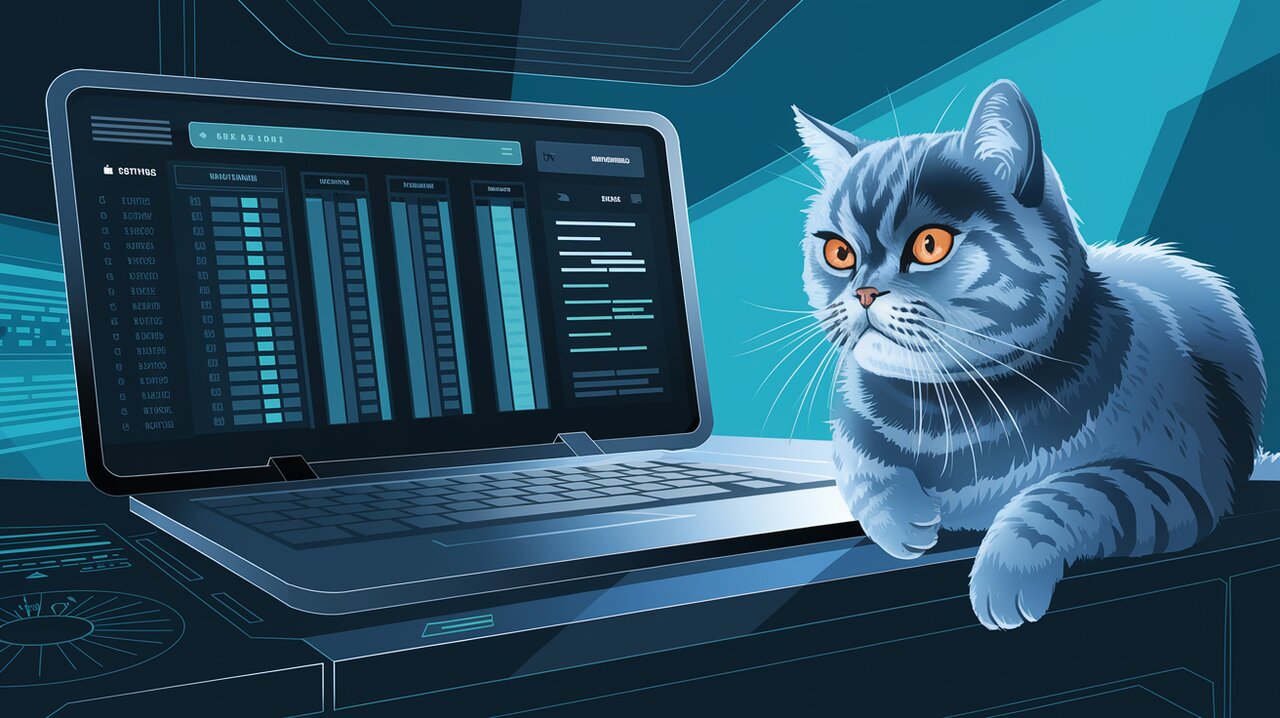
React Data Table Component: Unleashing Tabular Magic in React
Welcome to the world of React Data Table Component, a robust library that brings simplicity and flexibility to building data tables in React applications. Whether you’re developing dashboards or need interactive tables, this library offers essential features like sorting, pagination, and theming out of the box.
Key Features
- Declarative Configuration: Easily configure your tables using simple props.
- Built-in Sorting and Pagination: Enhance user experience with built-in functionalities.
- Selectable and Expandable Rows: Allow users to interact with rows for detailed information.
- Customizable Themes: Style your tables to match your application’s design.
- Responsive Design: Ensure your tables look great on any device.
Setting Up Your Environment
To get started with react-data-table-component
, you can install it via npm or yarn:
npm install react-data-table-component
or
yarn add react-data-table-component
Getting Started with Basic Usage
Creating a Simple Table
To create a basic table, you need to import the component and pass your data and columns as props:
import DataTable from 'react-data-table-component';
const columns = [
{ name: 'Title', selector: row => row.title },
{ name: 'Year', selector: row => row.year },
];
const data = [
{ id: 1, title: 'Inception', year: '2010' },
{ id: 2, title: 'Interstellar', year: '2014' },
];
<DataTable
columns={columns}
data={data}
/>
This code snippet demonstrates how easy it is to set up a table using react-data-table-component
. You define columns and data, then pass them to the DataTable
component.
Adding Pagination
Pagination is crucial for handling large datasets. Enable it by simply adding a pagination prop:
<DataTable
columns={columns}
data={data}
pagination
/>
With pagination enabled, your table will automatically handle page navigation for you.
Diving into Advanced Features
Customizing Themes
The library allows you to customize the appearance of your tables through themes:
import { createTheme } from 'react-data-table-component';
createTheme('solarized', {
text: {
primary: '#268bd2',
secondary: '#2aa198',
},
background: {
default: '#002b36',
},
});
<DataTable
columns={columns}
data={data}
theme="solarized"
/>
This example shows how you can create a custom theme named ‘solarized’ and apply it to your table.
Handling Row Expansion
For more interactive tables, you can enable row expansion:
const ExpandableComponent = ({ data }) => <div>{data.details}</div>;
<DataTable
columns={columns}
data={data}
expandableRows
expandableRowsComponent={ExpandableComponent}
/>
Row expansion allows users to view additional details about each entry without cluttering the main table view.
Wrapping Up
The React Data Table Component is an excellent choice for developers looking to implement feature-rich data tables in their React applications. With its ease of use and extensive customization options, it caters to both beginners and advanced users alike. For more insights into similar libraries, check out our articles on Syncfusion EJ2 React Charts and MUI X Data Grid.