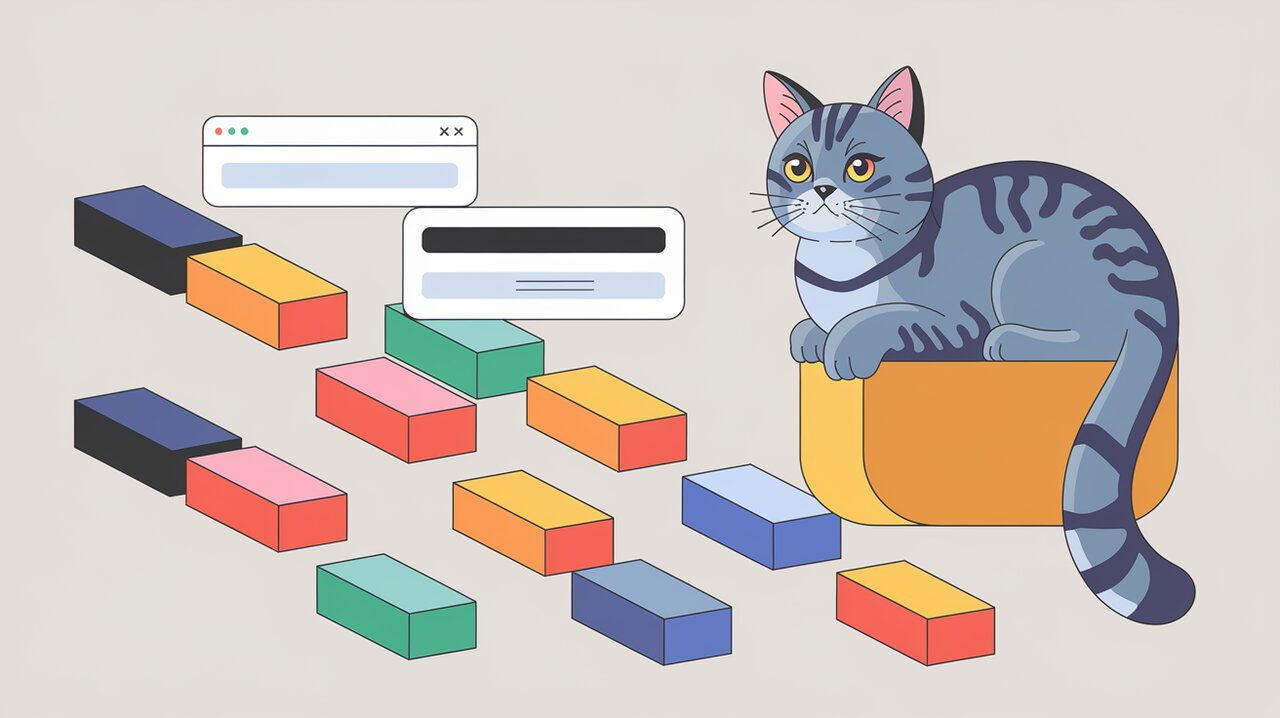
React DnD Multi Backend: Drag-and-Drop Magic Simplified
Introduction
Drag-and-drop interactions are integral to creating intuitive and interactive user experiences. react-dnd-multi-backend
takes this a step further by enabling seamless switching between multiple drag-and-drop backends, such as HTML5 and touch backends. This flexibility ensures your application performs optimally on both desktop and mobile devices.
Whether you’re building complex dashboards or simple to-do lists, react-dnd-multi-backend
empowers developers to handle diverse user interactions effortlessly.
Why Choose React DnD Multi Backend?
Here are some of the standout features of react-dnd-multi-backend
:
- Multi-Backend Support: Switch between HTML5 and touch backends dynamically based on user input.
- Customizable Pipelines: Create tailored backend pipelines for unique application needs.
- Preview Component: Simulate drag-and-drop visuals with ease, even on unsupported backends.
- Seamless Transitions: Built-in transitions ensure smooth backend switching without disrupting user interactions.
Getting Started
Installation
To get started, you need to install react-dnd-multi-backend
along with its dependencies:
Using npm:
npm install react-dnd react-dnd-html5-backend react-dnd-touch-backend react-dnd-multi-backend rdndmb-html5-to-touch
Using yarn:
yarn add react-dnd react-dnd-html5-backend react-dnd-touch-backend react-dnd-multi-backend rdndmb-html5-to-touch
Setting Up a Basic Pipeline
The library provides a default pipeline called HTML5toTouch
, which combines the HTML5 and touch backends. Let’s see how to integrate it into your application.
import React from 'react';
import { DndProvider } from 'react-dnd';
import { MultiBackend } from 'react-dnd-multi-backend';
import { HTML5toTouch } from 'rdndmb-html5-to-touch';
const App = () => {
return (
<DndProvider backend={MultiBackend} options={HTML5toTouch}>
<YourComponent />
</DndProvider>
);
};
export default App;
This setup ensures your application uses the HTML5 backend by default and switches to the touch backend when a touch event is detected.
Basic Usage
Implementing Drag Sources and Drop Targets
The core of any drag-and-drop functionality lies in defining draggable items (sources) and droppable areas (targets). Here’s an example:
Drag Source
import { useDrag } from 'react-dnd';
const DraggableItem = ({ id, name }: { id: string; name: string }) => {
const [{ isDragging }, drag] = useDrag(() => ({
type: 'ITEM',
item: { id },
collect: (monitor) => ({
isDragging: monitor.isDragging(),
}),
}));
return (
<div ref={drag} style={{ opacity: isDragging ? 0.5 : 1 }}>
{name}
</div>
);
};
Drop Target
import { useDrop } from 'react-dnd';
const DropZone = ({ onDrop }: { onDrop: (item: any) => void }) => {
const [{ isOver }, drop] = useDrop(() => ({
accept: 'ITEM',
drop: (item) => onDrop(item),
collect: (monitor) => ({
isOver: monitor.isOver(),
}),
}));
return (
<div ref={drop} style={{ backgroundColor: isOver ? '#f3f3f3' : '#ffffff', border: '1px dashed black' }}>
Drop here!
</div>
);
};
Combining Drag-and-Drop Components
Now, let’s combine these components in an application:
const App = () => {
const handleDrop = (item) => {
console.log('Dropped item:', item);
};
return (
<DndProvider backend={MultiBackend} options={HTML5toTouch}>
<DraggableItem id="1" name="Item One" />
<DropZone onDrop={handleDrop} />
</DndProvider>
);
};
Advanced Usage
Custom Pipelines
You can create custom pipelines to define your own backend transitions. Here’s an example:
import { HTML5Backend } from 'react-dnd-html5-backend';
import { TouchBackend } from 'react-dnd-touch-backend';
import { MouseTransition, TouchTransition } from 'react-dnd-multi-backend';
export const CustomPipeline = {
backends: [
{
id: 'html5',
backend: HTML5Backend,
transition: MouseTransition,
},
{
id: 'touch',
backend: TouchBackend,
options: { enableMouseEvents: true },
preview: true,
transition: TouchTransition,
},
],
};
Use this pipeline in your application:
<DndProvider options={CustomPipeline}>
<YourComponent />
</DndProvider>
Using Previews
The Preview
component allows you to render custom drag previews:
import { Preview } from 'react-dnd-preview';
const generatePreview = ({ itemType, item, style }) => (
<div style={{ ...style, backgroundColor: 'lightblue' }}>{itemType}: {item.id}</div>
);
const App = () => (
<DndProvider options={HTML5toTouch}>
<YourComponent />
<Preview generator={generatePreview} />
</DndProvider>
);
Multi-Drag and Multi-Drop Hooks
For more complex scenarios involving multiple backends, you can use useMultiDrag
and useMultiDrop
hooks to customize behavior per backend.
Example with useMultiDrag
import { useMultiDrag } from 'react-dnd-multi-backend';
const MultiDraggableItem = ({ color }: { color: string }) => {
const [[dragProps], { html5, touch }] = useMultiDrag({
type: 'card',
item: { color },
collect(monitor) {
return { isDragging: monitor.isDragging() };
},
});
return (
<div style={{ opacity: dragProps.isDragging ? 0.5 : 1 }}>
<div ref={html5[1]} style={{ backgroundColor: color }}>HTML5</div>
<div ref={touch[1]} style={{ backgroundColor: color }}>Touch</div>
</div>
);
};
Conclusion
With react-dnd-multi-backend
, building robust drag-and-drop interfaces that work seamlessly across devices has never been easier. Its ability to switch between multiple backends dynamically ensures that your applications deliver consistent experiences for all users.
Dive into the world of multi-backend drag-and-drop magic today! For more examples, check out related articles like “React Beautiful DnD Exploration” or “SortableJS React Drag-and-Drop Mastery”.