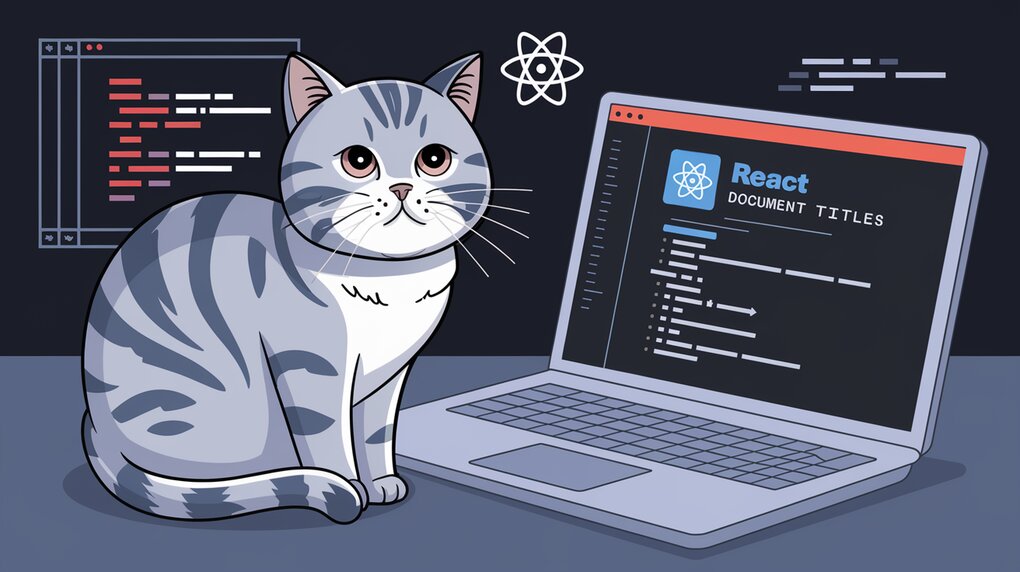
Exploring the React Document Title Library: A Declarative Approach to Document Titles
Exploring the React Document Title Library: A Declarative Approach to Document Titles
In the world of web development, managing document titles can be a crucial aspect of creating a seamless user experience. Titles are essential for various purposes, including search engine optimization (SEO), browser title bars, bookmarks, and more. React, being a popular JavaScript library for building web applications, provides several ways to manage document titles. One such method is through the react-document-title
library, which offers a declarative approach to specifying document.title
in a single-page app.
Introduction
The react-document-title
library allows developers to specify document titles in a declarative manner, making it easy to manage titles across their application. This library can be used on both the client-side and server-side, ensuring that titles are consistent and up-to-date regardless of the environment. With react-document-title
, developers can define titles at various levels within their application, from app-wide to page-specific, making it flexible and adaptable to different use cases.
Features
The react-document-title
library offers several key features that make it a powerful tool for managing document titles:
- Declarative: The library provides a declarative way to specify document titles, allowing developers to define titles in a straightforward manner.
- No DOM Emission: Unlike other libraries,
react-document-title
does not emit any DOM elements, ensuring that it does not interfere with the application’s rendering process. - Parent’s Props and State: The library can use the parent’s
props
andstate
, making it easy to integrate with other components. - Supports Arbitrary Levels of Nesting: Titles can be defined at various levels within the application, allowing for app-wide and page-specific titles.
- Works with Isomorphic Apps: The library is compatible with isomorphic applications, ensuring that titles are consistent across both client-side and server-side rendering.
Installation
To start using react-document-title
, you need to install it via npm or yarn:
npm install --save react-document-title
Basic Usage
The basic usage of react-document-title
involves wrapping your application components with the DocumentTitle
component and specifying the title as a prop. Here is a simple example:
import React from 'react';
import { DocumentTitle } from 'react-document-title';
function App() {
return (
<DocumentTitle title='My Web App'>
<SomeRouter />
</DocumentTitle>
);
}
In this example, the DocumentTitle
component is wrapped around the SomeRouter
component, and the title
prop is set to 'My Web App'
. This will set the document title to 'My Web App'
when the App
component is rendered.
Advanced Usage
For more complex scenarios, you can use the DocumentTitle
component within other components and update the title dynamically. Here is an example of how to use DocumentTitle
within a class component:
import React, { Component } from 'react';
import { DocumentTitle } from 'react-document-title';
class NewArticlePage extends Component {
constructor(props) {
super(props);
this.state = { title: 'Untitled' };
}
render() {
return (
<DocumentTitle title={this.state.title}>
<div>
<h1>New Article</h1>
<input
value={this.state.title}
onChange={(e) => this.setState({ title: e.target.value })}
/>
</div>
</DocumentTitle>
);
}
}
In this example, the DocumentTitle
component is wrapped around the NewArticlePage
component, and the title
prop is set to the value of the title
state variable. The input field updates the state, which in turn updates the document title.
Server-Side Rendering
When using react-document-title
on the server-side, you need to call the DocumentTitle.rewind()
method after rendering components to string to retrieve the title given to the innermost DocumentTitle
. This ensures that the title is embedded correctly into the HTML page template. Here is an example:
import { renderToString } from 'react-dom/server';
import { DocumentTitle } from 'react-document-title';
const markup = renderToString(<App />);
const title = DocumentTitle.rewind();
Conclusion
The react-document-title
library provides a powerful and flexible way to manage document titles in React applications. With its declarative approach, support for arbitrary levels of nesting, and compatibility with isomorphic apps, it is an essential tool for developers looking to enhance their application’s user experience. Whether you are building a simple single-page app or a complex isomorphic application, react-document-title
can help you manage document titles with ease.