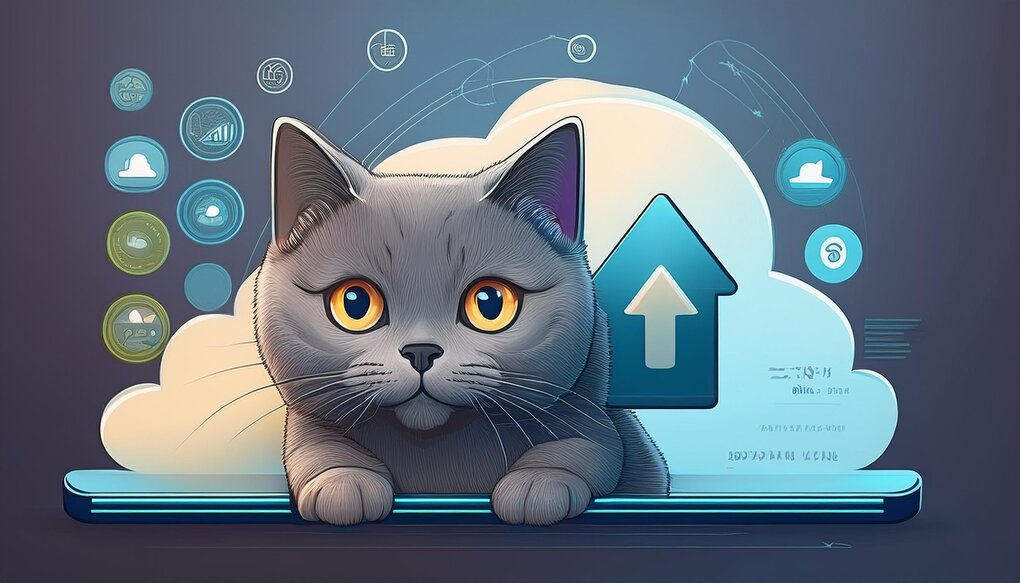
Introduction
The react-dropzone library is a powerful tool for developers looking to implement drag-and-drop file upload functionality in their React applications. It simplifies the process of allowing users to drag files from their file explorer and drop them into your application for upload. This library is especially useful in scenarios where user-friendly file upload interfaces are needed, such as in job application portals, content management systems, or any web app that requires file handling.
Installation
To get started with react-dropzone, you need to install it via npm or yarn. Here’s how you can do it:
# Using npm
npm install react-dropzone
# Using yarn
yarn add react-dropzone
Basic Usage with Code Examples
To create a simple drag-and-drop file upload interface, you can use the useDropzone
hook provided by the library. Here’s a basic example:
import React, { useCallback } from 'react';
import { useDropzone } from 'react-dropzone';
const MyDropzone: React.FC = () => {
const onDrop = useCallback((acceptedFiles: File[]) => {
console.log(acceptedFiles);
}, []);
const { getRootProps, getInputProps, isDragActive } = useDropzone({ onDrop });
return (
<div {...getRootProps()} style={{ border: '2px dashed #cccccc', padding: '20px', textAlign: 'center' }}>
<input {...getInputProps()} />
{isDragActive ? (
<p>Drop the files here ...</p>
) : (
<p>Drag 'n' drop some files here, or click to select files</p>
)}
</div>
);
};
export default MyDropzone;
This example sets up a basic drag-and-drop zone where users can drop files or click to open a file dialog. The onDrop
function logs the accepted files to the console.
Advanced Usage with Code Examples
For more advanced use cases, you might want to handle file previews, restrict file types, or manage multiple files. Here’s how you can extend the basic functionality:
Handling File Previews
import React, { useCallback, useState } from 'react';
import { useDropzone } from 'react-dropzone';
const MyDropzoneWithPreview: React.FC = () => {
const [files, setFiles] = useState<File[]>([]);
const onDrop = useCallback((acceptedFiles: File[]) => {
setFiles(acceptedFiles.map(file => Object.assign(file, {
preview: URL.createObjectURL(file)
})));
}, []);
const { getRootProps, getInputProps } = useDropzone({ onDrop });
return (
<div {...getRootProps()} style={{ border: '2px dashed #cccccc', padding: '20px', textAlign: 'center' }}>
<input {...getInputProps()} />
<p>Drag 'n' drop some files here, or click to select files</p>
<div>
{files.map(file => (
<div key={file.name}>
<img src={file.preview} alt={file.name} width={100} />
<p>{file.name}</p>
</div>
))}
</div>
</div>
);
};
export default MyDropzoneWithPreview;
Restricting File Types
To restrict file uploads to certain types, you can use the accept
option:
const { getRootProps, getInputProps } = useDropzone({
onDrop,
accept: 'image/*'
});
Managing Multiple Files
You can also limit the number of files using the maxFiles
option:
const { getRootProps, getInputProps } = useDropzone({
onDrop,
maxFiles: 3
});
Conclusion
The react-dropzone library is a versatile and easy-to-use tool for implementing drag-and-drop file uploads in React applications. With its simple API and extensive customization options, you can create intuitive and efficient file upload interfaces tailored to your application’s needs. Whether you’re building a simple file uploader or a complex system with file previews and type restrictions, react-dropzone has you covered. Happy coding!