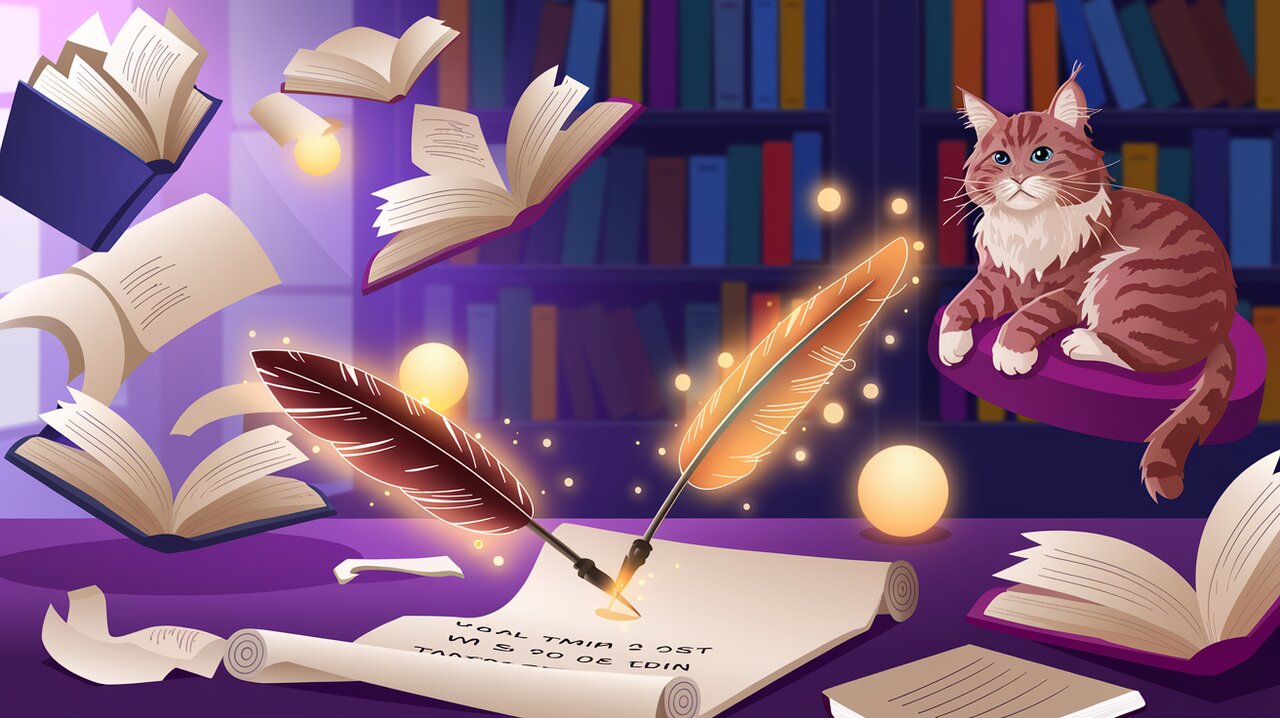
React Editor: Unleash Rich Text Wizardry in Your React Apps
Unleash the Power of Rich Text Editing
In the ever-evolving landscape of web development, creating engaging and interactive content is paramount. Enter react-editor, a powerful rich-text editing library that brings the magic of content creation to your React applications. With its seamless integration of TypeScript and React Hooks, react-editor offers a robust solution for developers seeking to implement advanced editing capabilities with ease and flexibility.
Spellbinding Features
react-editor comes packed with an array of enchanting features that will elevate your editing experience:
- Rich-text editing with full TypeScript and React Hooks integration
- Placeholder support via CSS for intuitive user guidance
- Essential methods like
focus()
,insertHTML(s)
, andinsertText(s)
- Automatic range saving, restoring, and end-of-content navigation
- Flexible value handling with
value/onChange
anddefaultValue/onChange
props - In-web image support and file drop handling
- Legacy support for projects using React versions prior to 16.8
Summoning the Editor
To bring the power of react-editor into your project, start by casting this simple spell in your terminal:
npm install react-editor
For those who prefer the yarn incantation:
yarn add react-editor
Crafting Your First Enchantment
Let’s dive into the basics of using react-editor in your React application. Here’s a simple example to get you started:
import React, { useState, useRef } from 'react';
import { Editor } from 'react-editor';
const MyEditor: React.FC = () => {
const [content, setContent] = useState('');
const editorRef = useRef<any>(null);
const handleFocus = () => {
if (editorRef.current) {
editorRef.current.focus();
}
};
const insertText = () => {
if (editorRef.current) {
editorRef.current.insertText('Hello, world!');
}
};
return (
<div>
<Editor
ref={editorRef}
placeholder="Start typing your magical story..."
value={content}
onChange={setContent}
/>
<button onClick={handleFocus}>Focus Editor</button>
<button onClick={insertText}>Insert Text</button>
</div>
);
};
export default MyEditor;
In this example, we create a basic editor component with focus and text insertion capabilities. The useRef
hook allows us to access the editor’s methods, while useState
manages the content state.
Advanced Incantations
Conjuring Images
react-editor supports in-web image handling, allowing users to seamlessly insert images into their content. Here’s how you can enable this feature:
import React from 'react';
import { Editor } from 'react-editor';
const ImageEditor: React.FC = () => {
const handleDrop = (e: React.DragEvent<HTMLDivElement>) => {
// Custom drop handling logic
console.log('File dropped:', e.dataTransfer.files);
};
return (
<Editor
allowInWebDrop={true}
onDrop={handleDrop}
placeholder="Drag and drop images here..."
/>
);
};
export default ImageEditor;
This setup allows users to drag and drop images directly into the editor. The onDrop
handler can be customized to process the dropped files as needed.
Enchanted HTML Processing
For more control over the content, you can use the processHTML
prop to transform HTML before it’s inserted into the editor:
import React from 'react';
import { Editor } from 'react-editor';
const ProcessingEditor: React.FC = () => {
const processHTML = (html: string): string => {
// Custom HTML processing logic
return html.replace(/<script>.*?<\/script>/g, ''); // Remove script tags
};
return (
<Editor
processHTML={processHTML}
placeholder="Enter your HTML content..."
/>
);
};
export default ProcessingEditor;
This example demonstrates how to remove potentially harmful <script>
tags from the inserted HTML, enhancing security.
Mastering the Arcane Arts
To truly harness the power of react-editor, let’s explore some advanced techniques:
Custom Styling
You can apply custom styles to your editor using the className
prop:
import React from 'react';
import { Editor } from 'react-editor';
import styles from './EditorStyles.module.css';
const StyledEditor: React.FC = () => {
return (
<Editor
className={styles.myCustomEditor}
placeholder="Type your stylish content here..."
/>
);
};
export default StyledEditor;
Controlled vs Uncontrolled Components
react-editor supports both controlled and uncontrolled component patterns. Here’s an example of each:
import React, { useState } from 'react';
import { Editor } from 'react-editor';
// Controlled Component
const ControlledEditor: React.FC = () => {
const [value, setValue] = useState('');
return (
<Editor
value={value}
onChange={setValue}
placeholder="Controlled editor..."
/>
);
};
// Uncontrolled Component
const UncontrolledEditor: React.FC = () => {
return (
<Editor
defaultValue="Initial content"
onChange={(newValue) => console.log('Content changed:', newValue)}
placeholder="Uncontrolled editor..."
/>
);
};
The controlled component allows for more precise state management, while the uncontrolled component offers simplicity for basic use cases.
Concluding the Spell
react-editor brings a touch of magic to the world of content creation in React applications. Its seamless integration of TypeScript and React Hooks, combined with a rich feature set, makes it an invaluable tool for developers looking to implement powerful editing capabilities. Whether you’re building a simple text input or a full-fledged content management system, react-editor provides the flexibility and functionality to bring your vision to life.
As you continue to explore the depths of this library, you’ll discover even more ways to enhance your users’ editing experience. Remember, the true power of react-editor lies not just in its features, but in how you wield them to create truly enchanting user interfaces.
For those seeking to further expand their React toolkit, you might also be interested in exploring other powerful libraries. Consider checking out articles on React DayPicker for advanced date selection or Yet Another React Lightbox for image galleries to complement your rich text editing capabilities.