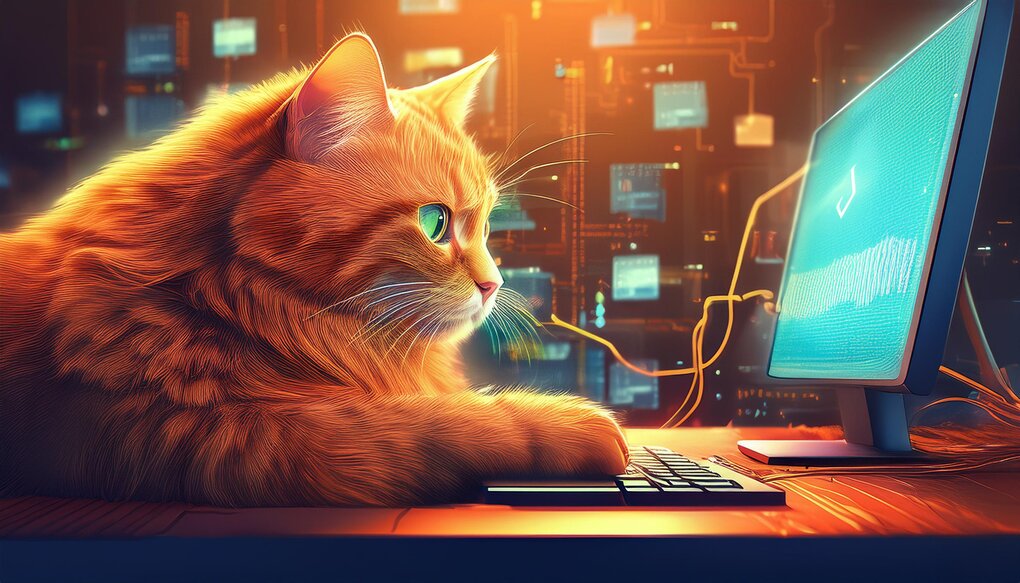
Installation
To get started with react-filepond
, you need to install it along with the core FilePond library. You can do this using either npm or yarn:
# Using npm
npm install react-filepond filepond --save
# Using yarn
yarn add react-filepond filepond
Basic Usage
After installation, you can start using the FilePond
component in your React application. Here’s a simple example to demonstrate its basic usage:
import React, { useState } from 'react';
import ReactDOM from 'react-dom';
import { FilePond } from 'react-filepond';
import 'filepond/dist/filepond.min.css';
function App() {
const [files, setFiles] = useState([]);
return (
<div className="App">
<FilePond
files={files}
onupdatefiles={setFiles}
allowMultiple={true}
maxFiles={3}
server="/api"
name="files"
labelIdle='Drag & Drop your files or <span class="filepond--label-action">Browse</span>'
/>
</div>
);
}
ReactDOM.render(<App />, document.getElementById('root'));
In this example, we import the necessary components and styles, and then define a simple App
component using React hooks. The FilePond
component is configured to allow multiple file uploads and is connected to a server endpoint.
What is FilePond?
FilePond is a versatile JavaScript library designed to simplify the process of uploading files in web applications. It provides a smooth, accessible, and visually appealing interface that supports a wide range of file types, including directories, files, blobs, local URLs, remote URLs, and Data URIs. The library is particularly known for its ability to handle asynchronous uploads, image optimization, and responsive design, making it a popular choice for developers looking to enhance user experience in file upload scenarios.
Core Features of FilePond
- Multiple Input Methods: Users can upload files via drag-and-drop, file selection from the filesystem, copy-pasting, or using the API.
- Asynchronous Uploads: Supports AJAX-based async uploading, with options to encode files as base64 data for form posts.
- Accessibility: Tested with assistive technologies like VoiceOver and JAWS, and is navigable by keyboard.
- Image Optimization: Automatically resizes images, crops them, and corrects EXIF orientation for optimal display.
- Responsive Design: Adapts to available screen space, ensuring functionality on both mobile and desktop devices.
Beyond Drag and Drop
While FilePond is often associated with its drag-and-drop functionality, it is not limited to this feature alone. FilePond can also serve as a standard file input, allowing users to click and select files from their filesystem. This flexibility makes it suitable for various use cases, whether you prefer a more traditional file selection method or a modern drag-and-drop interface.
Enhanced File Input
FilePond enhances the standard file input element by providing additional features such as:
- File Validation: Validate file types and sizes before upload using plugins like
filepond-plugin-file-validate-type
andfilepond-plugin-file-validate-size
. - Image Previews: Display previews of images before they are uploaded using plugins like
filepond-plugin-image-preview
. - Customizable Interface: Customize the look and feel of the file input to match your application’s design.
Advanced Usage
For more advanced use cases, you can customize react-filepond
with plugins and additional options. Below is an example that includes image preview and EXIF orientation correction:
import React, { useState } from 'react';
import ReactDOM from 'react-dom';
import { FilePond, registerPlugin } from 'react-filepond';
import 'filepond/dist/filepond.min.css';
import FilePondPluginImageExifOrientation from 'filepond-plugin-image-exif-orientation';
import FilePondPluginImagePreview from 'filepond-plugin-image-preview';
import 'filepond-plugin-image-preview/dist/filepond-plugin-image-preview.css';
registerPlugin(FilePondPluginImageExifOrientation, FilePondPluginImagePreview);
function App() {
const [files, setFiles] = useState([]);
return (
<div className="App">
<FilePond
files={files}
onupdatefiles={setFiles}
allowMultiple={true}
maxFiles={3}
server="/api"
name="files"
labelIdle='Drag & Drop your files or <span class="filepond--label-action">Browse</span>'
/>
</div>
);
}
ReactDOM.render(<App />, document.getElementById('root'));
In this setup, we register two plugins: FilePondPluginImageExifOrientation
and FilePondPluginImagePreview
. These plugins enhance the file upload experience by automatically correcting image orientation and providing a preview of the images.
Conclusion
The react-filepond
library is an excellent choice for developers looking to implement file uploads in React applications. It offers a wide range of features and customization options, making it suitable for both simple and complex use cases. By following this guide, you should be able to quickly set up and start using react-filepond
in your projects. Happy coding!