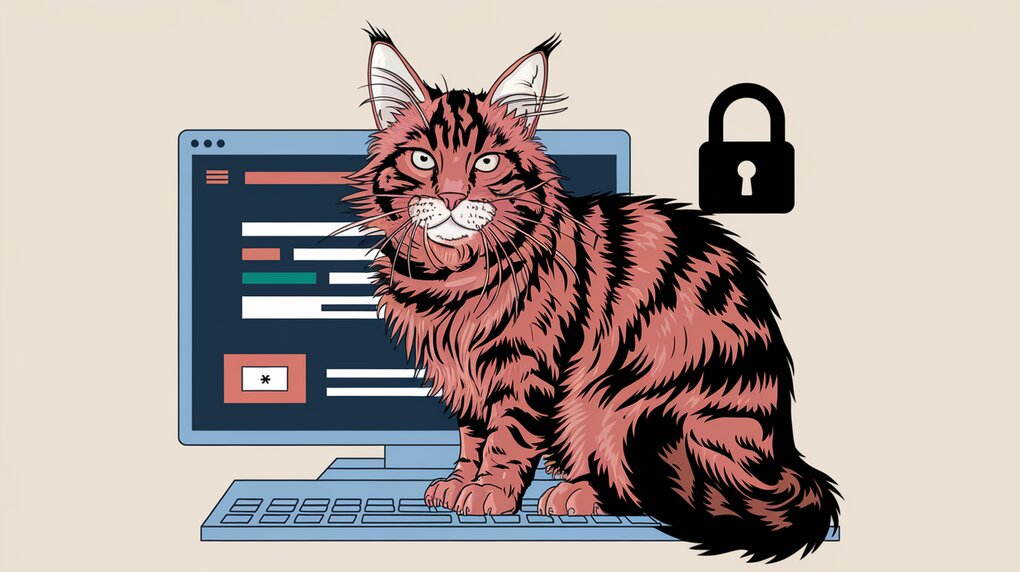
Mastering React Focus Lock: A Comprehensive Guide
Introduction
React Focus Lock is a versatile library that helps developers manage focus behavior within their React applications. This feature is crucial for creating accessible and user-friendly interfaces, particularly in scenarios where the user’s focus needs to be controlled or restricted to specific elements. In this guide, we will explore the key features, installation, basic and advanced usage, and conclude with a comprehensive overview of React Focus Lock.
Features
React Focus Lock offers several key features that make it an essential tool for developers:
- Browser-Friendly Focus Lock: The library ensures that focus is managed correctly across different browsers.
- Matching All Use Cases: It supports various use cases, including modal dialogs and focused tasks.
- Trusted by Best UI Frameworks: React Focus Lock is trusted by top UI frameworks such as Atlassian AtlasKit, ReachUI, SmoothUI, and Storybook.
- Portals Support: It allows rendering components outside the normal document flow while still maintaining focus.
- Scattered Locks: You can set up different isolated locks and switch between them using the
group
prop. - Controllable Isolation Level: You can control the isolation level of the focus lock.
- Variable Size Bundle: The library uses a sidecar to trim the UI part down to 1.5kb.
Installation
To install React Focus Lock, you can use npm or yarn:
npm install react-focus-lock
yarn add react-focus-lock
Basic Usage
Here is a simple example of how to use React Focus Lock to lock focus within a component:
import React from 'react';
import { FocusLock } from 'react-focus-lock';
const MyComponent = () => (
<FocusLock>
<div tabIndex={-1}>
<input type="text" />
<button>Click me</button>
</div>
</FocusLock>
);
In this example, the FocusLock
component wraps the input and button elements, ensuring that focus remains within this component.
Advanced Usage
React Focus Lock provides several advanced features that can be used to manage focus programmatically:
- Programmatic Focus Management: You can use the
useFocusInside
method to move focus inside a given node. - Focus Scattering: You can use the
shards
prop to manage focus across different nodes. - Focus Guards: You can use the
InFocusGuard
component to protect focus from being lost. - WhiteList: You can specify a function to determine which nodes can receive focus.
Here is an example of using shards
to manage focus across different nodes:
import React from 'react';
import { FocusLock } from 'react-focus-lock';
const MyComponent = () => (
<FocusLock shards={[ref1, ref2]}>
<div ref={ref1}>
<input type="text" />
</div>
<div ref={ref2}>
<button>Click me</button>
</div>
</FocusLock>
);
Conclusion
React Focus Lock is a powerful tool that simplifies the process of managing focus behavior within React applications. It provides a comprehensive set of features that help developers create accessible and user-friendly interfaces. By using React Focus Lock, developers can ensure that focus is managed correctly across different browsers and scenarios, enhancing the overall user experience.
License
React Focus Lock is licensed under the MIT License.