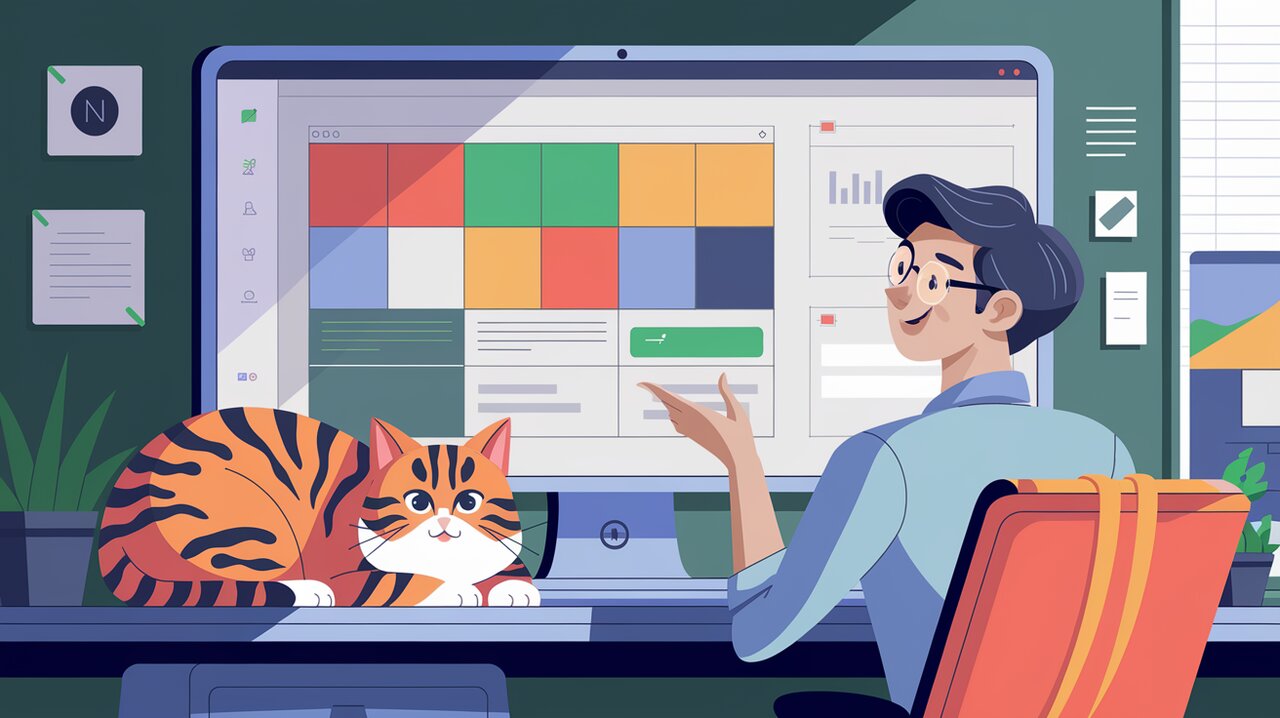
React Grid Layout: Crafting Fluid and Responsive Layouts with Ease
React Grid Layout is a powerful and flexible grid layout system designed specifically for React applications. It provides developers with the tools to create responsive, draggable, and resizable grid layouts, similar to popular systems like Packery or Gridster. However, React Grid Layout stands out by offering built-in support for responsive breakpoints and eliminating the need for jQuery.
Features
React Grid Layout comes packed with an impressive array of features that make it a go-to choice for developers looking to implement complex grid layouts:
- Responsive Design: Supports breakpoints for different screen sizes, allowing layouts to adapt seamlessly.
- Draggable Widgets: Users can easily rearrange grid items by dragging them to new positions.
- Resizable Widgets: Grid items can be resized, with optional minimum and maximum size constraints.
- Static Widgets: Ability to define non-draggable and non-resizable items within the grid.
- Flexible Packing: Options for horizontal, vertical, or no compacting of items.
- Bounds Checking: Ensures that dragging and resizing operations respect defined boundaries.
- Dynamic Updates: Widgets can be added or removed without rebuilding the entire grid.
- Layout Persistence: Layouts can be serialized and restored, perfect for saving user preferences.
- CSS Transforms: Utilizes CSS Transforms for smooth and efficient item positioning.
- Server-Side Rendering: Compatible with server-rendered applications.
Installation
To get started with React Grid Layout, you’ll need to install the package using npm or yarn. Open your terminal and run one of the following commands:
npm install react-grid-layout
or
yarn add react-grid-layout
After installation, make sure to include the necessary stylesheets in your application:
<link rel="stylesheet" href="/node_modules/react-grid-layout/css/styles.css">
<link rel="stylesheet" href="/node_modules/react-resizable/css/styles.css">
Basic Usage
Let’s dive into a simple example to demonstrate how to create a basic grid layout using React Grid Layout.
Creating a Simple Grid
Here’s how you can create a grid with three items, each with different properties:
import React from 'react';
import GridLayout from 'react-grid-layout';
const MyFirstGrid: React.FC = () => {
const layout = [
{ i: 'a', x: 0, y: 0, w: 1, h: 2, static: true },
{ i: 'b', x: 1, y: 0, w: 3, h: 2, minW: 2, maxW: 4 },
{ i: 'c', x: 4, y: 0, w: 1, h: 2 }
];
return (
<GridLayout className="layout" layout={layout} cols={12} rowHeight={30} width={1200}>
<div key="a">A</div>
<div key="b">B</div>
<div key="c">C</div>
</GridLayout>
);
};
export default MyFirstGrid;
In this example, we’ve created a grid with three items:
- Item ‘a’ is static and cannot be moved or resized.
- Item ‘b’ has a minimum width of 2 grid units and a maximum width of 4 grid units.
- Item ‘c’ is freely draggable and resizable.
Defining Layout Properties on Children
Alternatively, you can set layout properties directly on the child elements:
import React from 'react';
import GridLayout from 'react-grid-layout';
const MyFirstGrid: React.FC = () => {
return (
<GridLayout className="layout" cols={12} rowHeight={30} width={1200}>
<div key="a" data-grid={{x: 0, y: 0, w: 1, h: 2, static: true}}>A</div>
<div key="b" data-grid={{x: 1, y: 0, w: 3, h: 2, minW: 2, maxW: 4}}>B</div>
<div key="c" data-grid={{x: 4, y: 0, w: 1, h: 2}}>C</div>
</GridLayout>
);
};
export default MyFirstGrid;
This approach can be more convenient when working with dynamic content or when you prefer to keep layout information closer to the individual components.
Advanced Usage
React Grid Layout offers several advanced features that allow for more complex and interactive layouts. Let’s explore some of these capabilities.
Responsive Layouts
One of the most powerful features of React Grid Layout is its support for responsive layouts. You can define different layouts for various screen sizes:
import React from 'react';
import { Responsive, WidthProvider } from 'react-grid-layout';
const ResponsiveGridLayout = WidthProvider(Responsive);
const MyResponsiveGrid: React.FC = () => {
const layouts = {
lg: [
{ i: 'a', x: 0, y: 0, w: 1, h: 2, static: true },
{ i: 'b', x: 1, y: 0, w: 3, h: 2, minW: 2, maxW: 4 },
{ i: 'c', x: 4, y: 0, w: 1, h: 2 }
],
md: [
{ i: 'a', x: 0, y: 0, w: 1, h: 2, static: true },
{ i: 'b', x: 1, y: 0, w: 2, h: 2, minW: 2, maxW: 4 },
{ i: 'c', x: 3, y: 0, w: 1, h: 2 }
],
sm: [
{ i: 'a', x: 0, y: 0, w: 1, h: 2, static: true },
{ i: 'b', x: 1, y: 0, w: 1, h: 2, minW: 2, maxW: 4 },
{ i: 'c', x: 2, y: 0, w: 1, h: 2 }
]
};
return (
<ResponsiveGridLayout
className="layout"
layouts={layouts}
breakpoints={{ lg: 1200, md: 996, sm: 768, xs: 480, xxs: 0 }}
cols={{ lg: 12, md: 10, sm: 6, xs: 4, xxs: 2 }}
>
<div key="a">A</div>
<div key="b">B</div>
<div key="c">C</div>
</ResponsiveGridLayout>
);
};
export default MyResponsiveGrid;
This example defines different layouts for large (lg), medium (md), and small (sm) screen sizes. The WidthProvider
HOC automatically detects width changes and updates the layout accordingly.
Dynamic Add/Remove of Grid Items
React Grid Layout allows for the dynamic addition and removal of grid items. Here’s an example of how you can implement this:
import React, { useState } from 'react';
import GridLayout from 'react-grid-layout';
const DynamicGrid: React.FC = () => {
const [items, setItems] = useState([
{ i: 'a', x: 0, y: 0, w: 1, h: 2 },
{ i: 'b', x: 1, y: 0, w: 3, h: 2 },
{ i: 'c', x: 4, y: 0, w: 1, h: 2 }
]);
const addItem = () => {
const newItem = {
i: `n${items.length + 1}`,
x: (items.length * 2) % 12,
y: Infinity,
w: 2,
h: 2
};
setItems([...items, newItem]);
};
const removeItem = (itemId: string) => {
setItems(items.filter(item => item.i !== itemId));
};
return (
<div>
<button onClick={addItem}>Add Item</button>
<GridLayout
className="layout"
layout={items}
cols={12}
rowHeight={30}
width={1200}
>
{items.map(item => (
<div key={item.i}>
{item.i}
<span onClick={() => removeItem(item.i)} style={{ cursor: 'pointer', float: 'right' }}>x</span>
</div>
))}
</GridLayout>
</div>
);
};
export default DynamicGrid;
This example demonstrates how to add new items to the grid and remove existing ones dynamically.
Saving and Restoring Layouts
For applications that require layout persistence, React Grid Layout makes it easy to save and restore layouts:
import React, { useState, useEffect } from 'react';
import GridLayout from 'react-grid-layout';
const PersistentGrid: React.FC = () => {
const [layout, setLayout] = useState(() => {
const savedLayout = localStorage.getItem('myGridLayout');
return savedLayout ? JSON.parse(savedLayout) : [
{ i: 'a', x: 0, y: 0, w: 1, h: 2 },
{ i: 'b', x: 1, y: 0, w: 3, h: 2 },
{ i: 'c', x: 4, y: 0, w: 1, h: 2 }
];
});
useEffect(() => {
localStorage.setItem('myGridLayout', JSON.stringify(layout));
}, [layout]);
const onLayoutChange = (newLayout: Layout[]) => {
setLayout(newLayout);
};
return (
<GridLayout
className="layout"
layout={layout}
cols={12}
rowHeight={30}
width={1200}
onLayoutChange={onLayoutChange}
>
<div key="a">A</div>
<div key="b">B</div>
<div key="c">C</div>
</GridLayout>
);
};
export default PersistentGrid;
This example saves the layout to localStorage whenever it changes and restores it when the component mounts.
Conclusion
React Grid Layout is a powerful tool for creating flexible and responsive grid layouts in React applications. Its rich feature set, including draggable and resizable widgets, responsive breakpoints, and layout persistence, makes it an excellent choice for building complex user interfaces.
By leveraging React Grid Layout, developers can create dynamic, user-friendly layouts that adapt to different screen sizes and user interactions. Whether you’re building dashboards, content management systems, or any application that requires a flexible grid layout, React Grid Layout provides the tools you need to create polished and professional user interfaces.
As you continue to explore React Grid Layout, you’ll discover even more advanced features and use cases that can enhance your React applications. Happy coding!