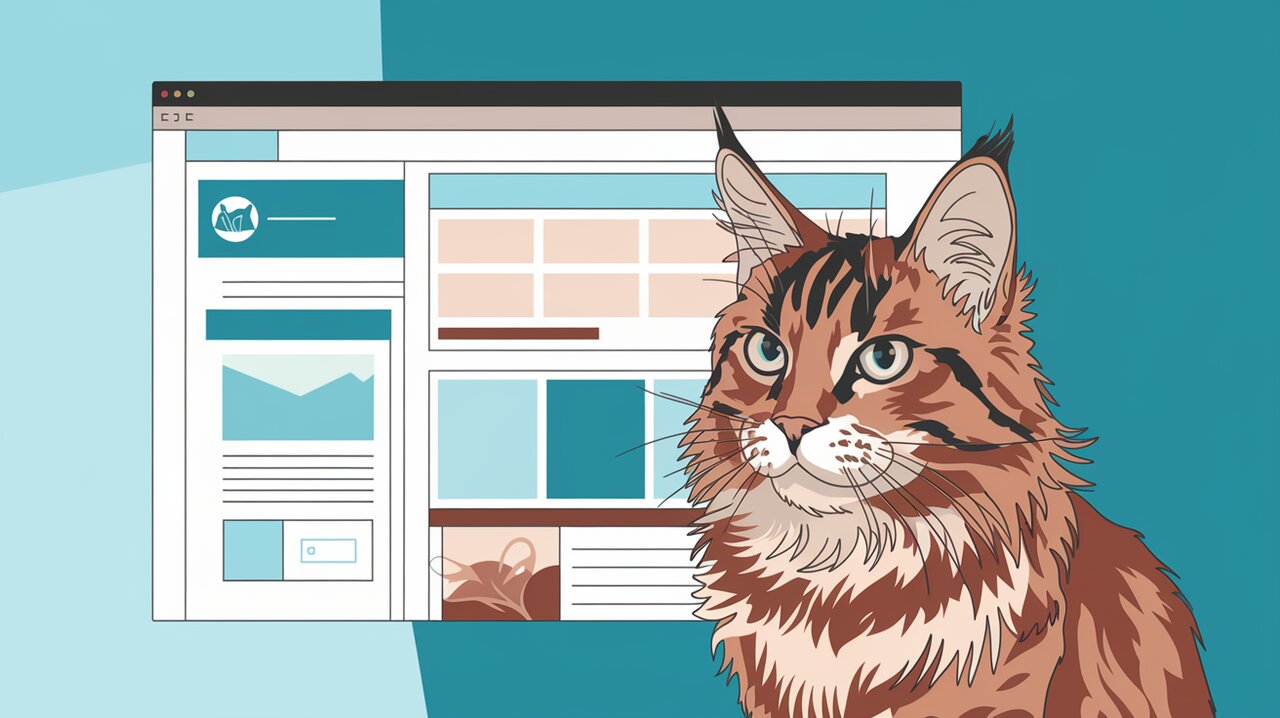
React-Headroom: Elevate Your Header Game with Smart Scrolling
React Headroom is a powerful React component that brings intelligent scrolling behavior to your website’s header. It allows you to create headers that hide when users scroll down and reappear when they scroll up, optimizing screen space and enhancing user experience. This library is particularly useful for single-page applications or long-scrolling websites where maintaining easy access to navigation is crucial.
Features
- Automatic header visibility management based on scroll direction
- Customizable pinning and unpinning behavior
- Support for custom styling and animations
- Lightweight and easy to integrate into existing React projects
- No external dependencies
Installation
You can install react-headroom using npm or yarn. Here are the commands for both package managers:
Using npm:
npm install react-headroom
Using yarn:
yarn add react-headroom
Basic Usage
Simple Implementation
To get started with react-headroom, you can wrap your header content with the Headroom
component. Here’s a basic example:
import React from 'react';
import Headroom from 'react-headroom';
const Header: React.FC = () => {
return (
<Headroom>
<header>
<h1>My Website</h1>
<nav>
<ul>
<li><a href="/">Home</a></li>
<li><a href="/about">About</a></li>
<li><a href="/contact">Contact</a></li>
</ul>
</nav>
</header>
</Headroom>
);
};
export default Header;
In this example, the Headroom
component wraps the entire header content. By default, it will hide the header when the user scrolls down and show it when they scroll up.
Customizing Behavior
React Headroom allows you to customize its behavior through props. Here’s an example with some common customizations:
import React from 'react';
import Headroom from 'react-headroom';
const Header: React.FC = () => {
return (
<Headroom
upTolerance={10}
downTolerance={10}
pinStart={300}
>
<header>
<h1>My Website</h1>
<nav>{/* Navigation items */}</nav>
</header>
</Headroom>
);
};
export default Header;
In this example, we’ve added three props:
upTolerance
: Sets the scroll tolerance in pixels when scrolling up before the header is pinned.downTolerance
: Sets the scroll tolerance in pixels when scrolling down before the header is unpinned.pinStart
: Specifies the distance from the top of the page where the header should start pinning.
These props allow you to fine-tune when the header appears and disappears based on the user’s scrolling behavior.
Advanced Usage
Styling and Animations
React Headroom applies CSS classes to the header based on its state, allowing you to create custom animations and styles. Here’s an example of how you can use these classes:
import React from 'react';
import Headroom from 'react-headroom';
import './Header.css';
const Header: React.FC = () => {
return (
<Headroom className="custom-headroom">
<header>
<h1>My Website</h1>
<nav>{/* Navigation items */}</nav>
</header>
</Headroom>
);
};
export default Header;
And in your CSS file:
.custom-headroom {
transition: all 0.5s ease-in-out;
}
.headroom--pinned {
transform: translateY(0%);
}
.headroom--unpinned {
transform: translateY(-100%);
}
.headroom--unfixed {
position: relative;
}
This CSS will create a smooth transition effect when the header is pinned or unpinned.
Callback Functions
React Headroom provides callback props that allow you to execute code when the header’s state changes. Here’s an example:
import React from 'react';
import Headroom from 'react-headroom';
const Header: React.FC = () => {
const handlePin = () => {
console.log('Header was pinned');
// You can add any logic here, like changing state or triggering animations
};
const handleUnpin = () => {
console.log('Header was unpinned');
// You can add any logic here, like changing state or triggering animations
};
return (
<Headroom
onPin={handlePin}
onUnpin={handleUnpin}
>
<header>
<h1>My Website</h1>
<nav>{/* Navigation items */}</nav>
</header>
</Headroom>
);
};
export default Header;
These callbacks can be useful for triggering additional effects or state changes in your application based on the header’s visibility.
Disabling the Component
There might be situations where you want to temporarily disable the headroom behavior. You can do this using the disable
prop:
import React, { useState } from 'react';
import Headroom from 'react-headroom';
const Header: React.FC = () => {
const [isDisabled, setIsDisabled] = useState(false);
return (
<>
<Headroom disable={isDisabled}>
<header>
<h1>My Website</h1>
<nav>{/* Navigation items */}</nav>
</header>
</Headroom>
<button onClick={() => setIsDisabled(!isDisabled)}>
{isDisabled ? 'Enable' : 'Disable'} Headroom
</button>
</>
);
};
export default Header;
This example includes a button that toggles the headroom behavior on and off, which can be useful for certain user interactions or responsive design considerations.
Conclusion
React Headroom is a versatile and powerful library that can significantly enhance the user experience of your React applications. By intelligently managing header visibility based on scroll behavior, it helps create more screen space for content while maintaining easy access to navigation.
With its simple integration, customizable behavior, and support for advanced styling and animations, react-headroom is an excellent choice for developers looking to create modern, user-friendly interfaces. Whether you’re building a single-page application, a long-scrolling website, or any React-based project that could benefit from smart header management, react-headroom provides an elegant solution that’s easy to implement and customize to your specific needs.