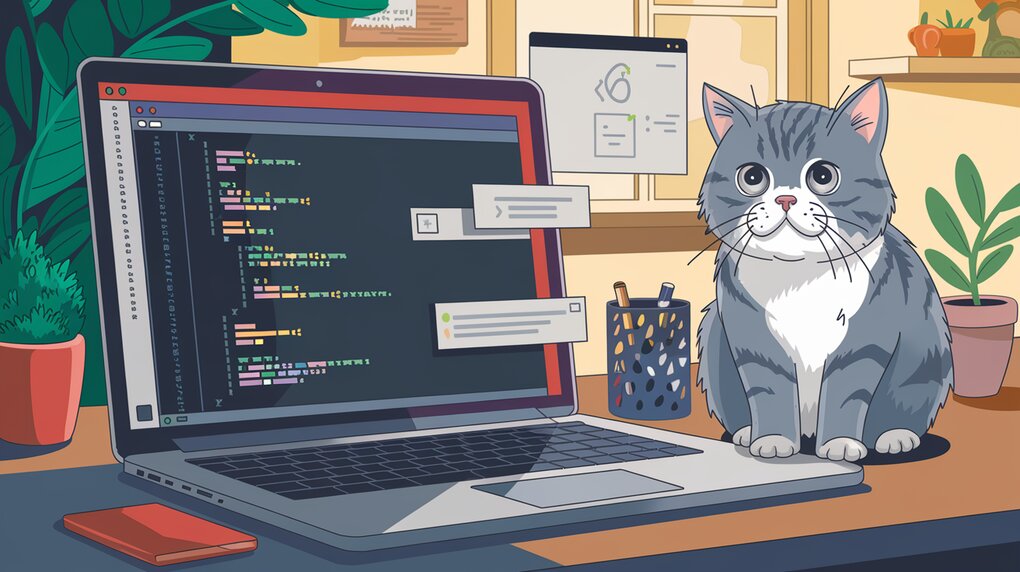
Unveiling the Secrets of react-hook-inview
Introduction
The react-hook-inview library is a handy tool for React developers who need to determine when elements are visible in the viewport. It leverages the Intersection Observer API to provide a simple hook that can be used for various purposes like lazy loading images, triggering animations, or loading data when an element comes into view.
Installation
To get started with react-hook-inview, you can install it using either npm or yarn:
npm install react-hook-inview
Or with yarn:
yarn add react-hook-inview
For older browsers that do not support the Intersection Observer API, consider installing a polyfill.
Basic Usage
The useInView
hook is the core of this library. Here’s a simple example to illustrate its usage:
import React from 'react';
import { useInView } from 'react-hook-inview';
const BasicComponent: React.FC = () => {
const [ref, isVisible] = useInView({
threshold: 1,
});
return <div ref={ref}>{isVisible ? 'Hello World!' : ''}</div>;
};
export default BasicComponent;
In this example, the isVisible
boolean determines whether the element is fully in the viewport, toggling the display of “Hello World!“.
Advanced Usage
For more advanced scenarios, useInView
offers additional features such as returning the IntersectionObserverEntry
and the observer itself. This allows for more complex interactions and optimizations:
import React from 'react';
import { useInView } from 'react-hook-inview';
const AdvancedComponent: React.FC = () => {
const [ref, isVisible, entry, observer] = useInView({
rootMargin: '0px 0px -50% 0px',
threshold: [0.25, 0.5, 0.75],
unobserveOnEnter: true,
});
React.useEffect(() => {
if (isVisible) {
console.log('Element is visible:', entry);
}
}, [isVisible, entry]);
return <div ref={ref}>{isVisible ? 'Advanced View!' : ''}</div>;
};
export default AdvancedComponent;
In this example, the element is observed with multiple thresholds, and the observer stops observing once the element is in view. The entry
provides detailed information about the intersection.
Conclusion
The react-hook-inview library simplifies the process of detecting element visibility in React applications. Whether you’re implementing lazy loading, animations, or other viewport-related features, this library provides a robust and flexible solution. By leveraging the Intersection Observer API, it ensures efficient performance across modern browsers.