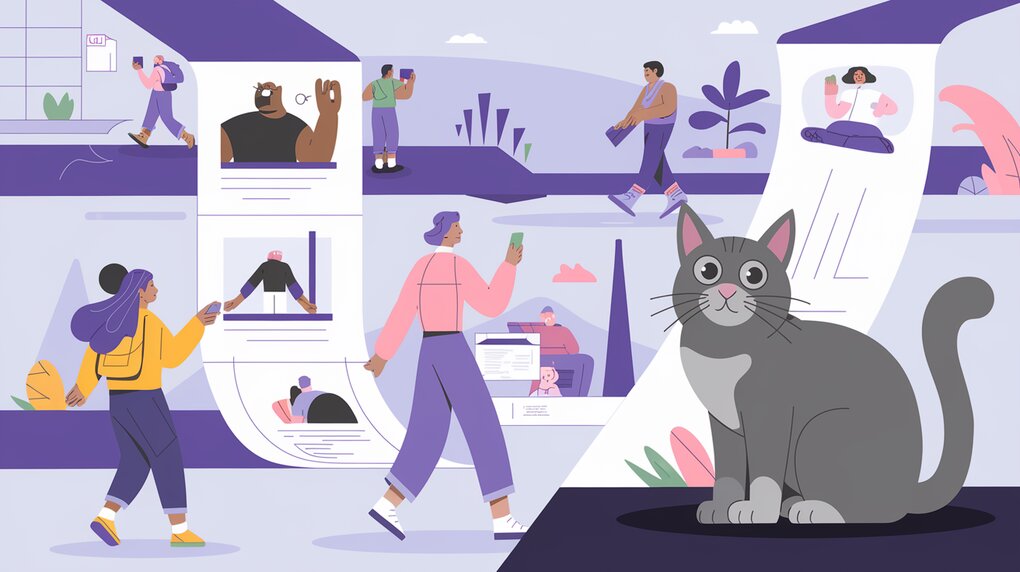
Effortless Image Loading with React-Lazy-Load-Image-Component
In the world of web development, optimizing image loading is crucial for enhancing performance and user experience. The react-lazy-load-image-component library offers a simple yet effective solution for lazy loading images in React applications. By deferring the loading of images until they are in the viewport, you can significantly improve page load times and reduce bandwidth usage.
Features
- Lazy Loading: Automatically loads images only when they enter the viewport.
- Placeholder Support: Displays a placeholder while the image is loading.
- Customizable: Offers various options to customize the loading behavior and appearance.
Installation
To get started with the react-lazy-load-image-component, you can install it using npm or yarn:
npm install react-lazy-load-image-component
or
yarn add react-lazy-load-image-component
Basic Usage with Code Examples
Using the library is straightforward. Here’s a simple example to demonstrate how you can implement lazy loading for an image:
import React from 'react';
import { LazyLoadImage } from 'react-lazy-load-image-component';
import 'react-lazy-load-image-component/src/effects/blur.css';
const MyComponent: React.FC = () => (
<div>
<LazyLoadImage
alt="A beautiful landscape"
height={400}
src="path/to/image.jpg"
width={600}
effect="blur"
/>
</div>
);
export default MyComponent;
In this example, the image will only load when it is about to enter the viewport, with a blur effect applied during loading.
Advanced Usage with Code Examples
For more advanced scenarios, you can customize the behavior and appearance of the lazy-loaded images. Here’s how you can use placeholders and handle events:
import React from 'react';
import { LazyLoadImage } from 'react-lazy-load-image-component';
const AdvancedComponent: React.FC = () => (
<div>
<LazyLoadImage
alt="A scenic view"
height={400}
src="path/to/another-image.jpg"
width={600}
placeholderSrc="path/to/placeholder.jpg"
onError={(e) => console.error('Image failed to load', e)}
afterLoad={() => console.log('Image loaded successfully')}
/>
</div>
);
export default AdvancedComponent;
In this advanced example, a placeholder image is displayed while the main image loads. Additionally, event handlers are used to handle loading errors and log successful loads.
Conclusion
The react-lazy-load-image-component library is a powerful tool for optimizing image loading in React applications. By implementing lazy loading, you can enhance the performance and user experience of your web applications. Whether you’re dealing with simple image galleries or complex media-heavy sites, this library provides the flexibility and ease of use needed to manage image loading efficiently.