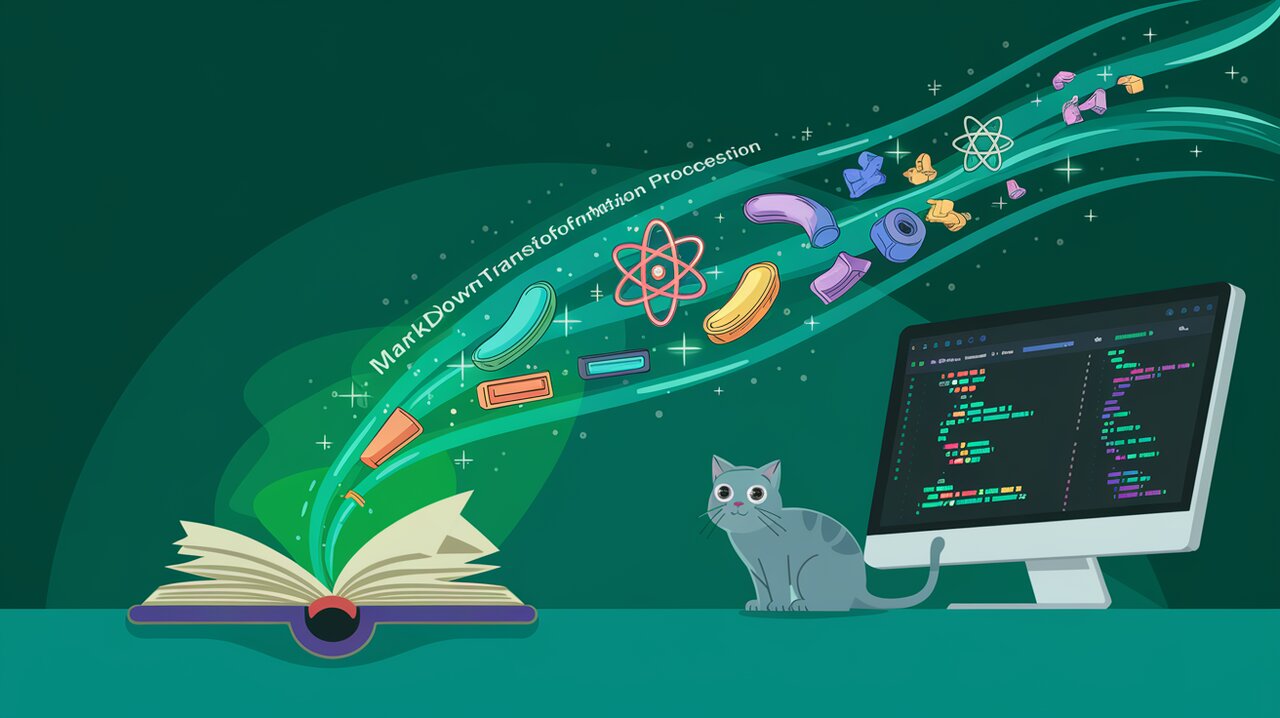
React-markdown is a powerful and flexible library that allows developers to seamlessly render Markdown content within React applications. This component-based approach to Markdown parsing offers a safe, customizable, and efficient solution for transforming plain text into rich, interactive JSX elements.
Features
React-markdown boasts several key features that make it stand out:
- Safety First: The library is designed to be secure by default, avoiding the use of
dangerouslySetInnerHTML
and protecting against XSS attacks. - Component Customization: Developers can easily override default HTML elements with custom React components, allowing for greater control over the rendered output.
- Plugin Support: React-markdown integrates well with various plugins, enabling extended functionality and syntax support.
- Compliance: The library offers 100% compliance with CommonMark specifications and can achieve full GitHub Flavored Markdown (GFM) support with additional plugins.
Installation
To get started with react-markdown, you can install it using npm or yarn:
npm install react-markdown
or
yarn add react-markdown
Basic Usage
Once installed, you can import and use the react-markdown component in your React application. Here’s a simple example:
import React from 'react';
import ReactMarkdown from 'react-markdown';
const App = () => {
const markdown = '# Hello, *world*!';
return <ReactMarkdown>{markdown}</ReactMarkdown>;
};
export default App;
This code will render a heading with “Hello, world!” where “world” is italicized.
Advanced Usage
Custom Components
One of the most powerful features of react-markdown is the ability to use custom components for rendering specific Markdown elements. Here’s an example of how to customize the rendering of headings:
import React from 'react';
import ReactMarkdown from 'react-markdown';
const CustomHeading = ({ level, children }) => {
const Tag = `h${level}`;
return <Tag style={{ color: 'blue' }}>{children}</Tag>;
};
const App = () => {
const markdown = '# Blue Heading\n\nNormal paragraph';
return (
<ReactMarkdown
components={{
h1: ({ node, ...props }) => <CustomHeading level={1} {...props} />,
h2: ({ node, ...props }) => <CustomHeading level={2} {...props} />,
}}
>
{markdown}
</ReactMarkdown>
);
};
export default App;
In this example, we’ve created a custom component for headings that applies a blue color to all heading levels.
Using Plugins
React-markdown supports various plugins to extend its functionality. For instance, you can use the remark-gfm
plugin to add support for GitHub Flavored Markdown features:
import React from 'react';
import ReactMarkdown from 'react-markdown';
import remarkGfm from 'remark-gfm';
const App = () => {
const markdown = 'Hello world ~strike~ ~~through~~';
return <ReactMarkdown remarkPlugins={[remarkGfm]}>{markdown}</ReactMarkdown>;
};
export default App;
This will render the strikethrough text correctly according to GFM syntax.
Syntax Highlighting
For code blocks, you can integrate syntax highlighting libraries like Prism.js. Here’s an example using the react-syntax-highlighter
package:
import React from 'react';
import ReactMarkdown from 'react-markdown';
import { Prism as SyntaxHighlighter } from 'react-syntax-highlighter';
import { dark } from 'react-syntax-highlighter/dist/esm/styles/prism';
const App = () => {
const markdown = '```javascript\nconst greeting = "Hello, world!";\nconsole.log(greeting);\n```';
return (
<ReactMarkdown
components={{
code({ node, inline, className, children, ...props }) {
const match = /language-(\w+)/.exec(className || '');
return !inline && match ? (
<SyntaxHighlighter
style={dark}
language={match[1]}
PreTag="div"
{...props}
>
{String(children).replace(/\n$/, '')}
</SyntaxHighlighter>
) : (
<code className={className} {...props}>
{children}
</code>
);
},
}}
>
{markdown}
</ReactMarkdown>
);
};
export default App;
This setup will apply syntax highlighting to code blocks in your Markdown content.
Conclusion
React-markdown provides a robust solution for rendering Markdown content in React applications. Its focus on safety, customization, and extensibility makes it an excellent choice for developers looking to integrate Markdown support into their projects. By leveraging custom components and plugins, you can create rich, interactive Markdown-based interfaces that seamlessly blend with your React application’s design and functionality.
As you explore react-markdown further, you’ll discover even more ways to tailor the Markdown rendering process to your specific needs, whether you’re building a simple blog or a complex documentation system. The library’s flexibility and performance make it a valuable tool in any React developer’s toolkit.