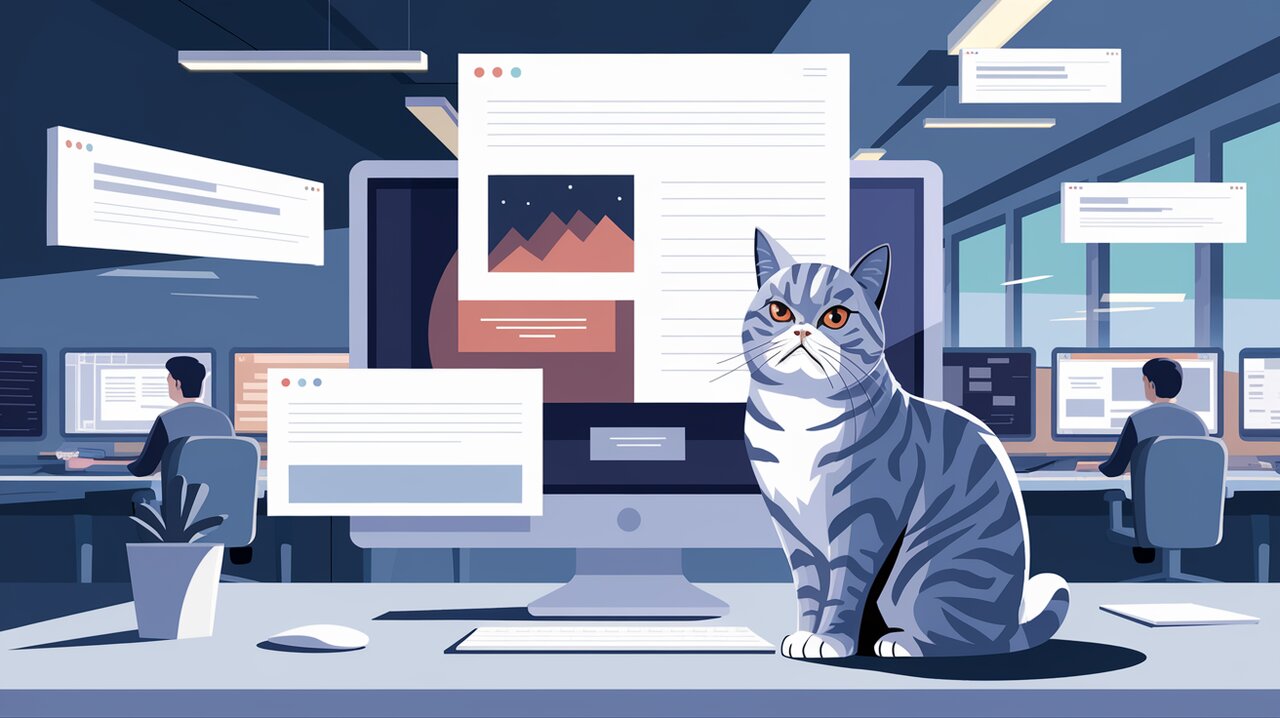
React Marquee Line is a powerful and flexible React component that brings the classic marquee effect into the modern web development era. This library allows developers to create smooth, customizable scrolling elements that can move horizontally or vertically, adding a dynamic touch to any React application.
Features
React Marquee Line comes packed with several features that make it stand out:
- Low rendering pressure: The component is optimized to minimize strain on the rendering engine.
- Flexible content: Supports both React elements and strings as scrolling items.
- Customizable speed: Easily adjust the scrolling speed to match your design needs.
- Bi-directional scrolling: Choose between horizontal and vertical scrolling directions.
Installation
To get started with React Marquee Line, you’ll need to install it in your React project. You can do this using either npm or yarn.
Using npm:
npm install react-marquee-line
Using yarn:
yarn add react-marquee-line
Basic Usage
Once installed, you can start using React Marquee Line in your components. Here’s a simple example of how to create a horizontal marquee:
import React from 'react';
import Marquee from 'react-marquee-line';
const App = () => {
const list = [
'First item',
'Second item',
'Third item',
];
return (
<Marquee list={list} />
);
};
export default App;
This code will create a horizontal marquee with three text items scrolling from right to left.
Advanced Usage
Customizing Direction
React Marquee Line allows you to change the scrolling direction. Here’s how you can create a vertical marquee:
import React from 'react';
import Marquee from 'react-marquee-line';
const VerticalMarquee = () => {
const list = [
'Scrolling up',
'Moving vertically',
'Fading in and out',
];
return (
<Marquee list={list} direction="vertical" />
);
};
export default VerticalMarquee;
Using React Elements
You’re not limited to just strings; you can also use React elements in your marquee:
import React from 'react';
import Marquee from 'react-marquee-line';
const MarqueeWithElements = () => {
const clickableItem = (
<span>
<a href="https://example.com">Click me</a> for more details
</span>
);
const list = [
'Plain text item',
clickableItem,
'Another text item',
];
return (
<Marquee list={list} />
);
};
export default MarqueeWithElements;
Adjusting Speed
For horizontal marquees, you can control the scrolling speed using the gear
prop:
import React from 'react';
import Marquee from 'react-marquee-line';
const FastMarquee = () => {
const list = ['Fast', 'Faster', 'Fastest'];
return (
<Marquee list={list} gear={2.5} />
);
};
export default FastMarquee;
The gear
prop accepts values of 1, 1.5, 2, or 2.5, with higher values resulting in faster scrolling.
Multi-line Vertical Marquee
For vertical marquees, you can specify how many lines to display at once:
import React from 'react';
import Marquee from 'react-marquee-line';
const MultiLineVerticalMarquee = () => {
const list = [
'Line 1',
'Line 2',
'Line 3',
'Line 4',
'Line 5',
];
return (
<Marquee list={list} direction="vertical" lines={3} />
);
};
export default MultiLineVerticalMarquee;
This will display three lines of text at a time, scrolling vertically.
Styling
React Marquee Line comes with basic styling, but you’ll likely want to customize it to match your application’s design. To do this, you can create a CSS file with your preferred styles and import it after the Marquee component.
Here’s an example of how you might style the marquee:
.react-marquee-line-container {
background-color: #f0f0f0;
padding: 10px;
border-radius: 5px;
}
.react-marquee-line-content {
font-family: Arial, sans-serif;
font-size: 16px;
color: #333;
}
Make sure to import this CSS file in your component file after importing the Marquee component.
Conclusion
React Marquee Line offers a simple yet powerful way to add scrolling text and elements to your React applications. With its customizable options for direction, speed, and content, you can create engaging user interfaces that capture attention and convey information dynamically.
As you integrate React Marquee Line into your projects, remember to consider performance implications, especially when using complex React elements or a large number of items in your marquee. Always test your implementation thoroughly to ensure smooth performance across different devices and browsers.
By leveraging the features of React Marquee Line, you can enhance your user interfaces with fluid, eye-catching animations that bring your content to life. Whether you’re building a news ticker, a product showcase, or simply want to add some movement to your static pages, React Marquee Line provides the tools you need to create compelling scrolling effects with ease.