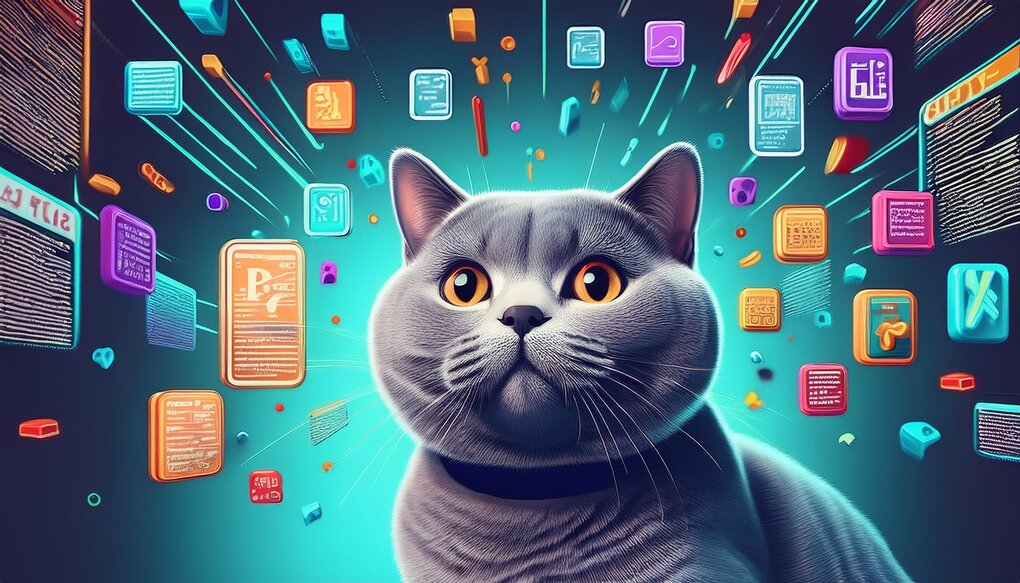
What is React-Move?
React-Move is a lightweight library designed for creating beautiful, data-driven animations in React applications. It offers fine-grained control over animations, allowing developers to specify delay, duration, and easing functions. React-Move is particularly useful for animating HTML, SVG, and React Native components, making it a versatile choice for developers looking to enhance their user interfaces with smooth transitions.
Installation
To get started with React-Move, you can install it using npm or yarn:
# Using npm
npm install react-move
# Using yarn
yarn add react-move
Basic Usage
React-Move provides two main components: NodeGroup and Animate. Let’s explore how to use these components with simple examples.
NodeGroup Example
The NodeGroup
component is used when you have an array of items that need to enter, update, or leave the DOM.
import React from 'react';
import { NodeGroup } from 'react-move';
const data = [
{ id: 1, value: 100 },
{ id: 2, value: 200 },
];
const MyComponent: React.FC = () => (
<NodeGroup
data={data}
keyAccessor={(d) => d.id.toString()}
start={() => ({ opacity: 0 })}
enter={() => ({ opacity: [1], timing: { duration: 500 } })}
update={() => ({ opacity: [1] })}
leave={() => ({ opacity: [0], timing: { duration: 500 } })}
>
{(nodes) => (
<div>
{nodes.map(({ key, data, state }) => (
<div key={key} style={{ opacity: state.opacity }}>
{data.value}
</div>
))}
</div>
)}
</NodeGroup>
);
export default MyComponent;
Animate Example
The Animate
component is used for animating a single item.
import React from 'react';
import { Animate } from 'react-move';
const MyAnimatedComponent: React.FC = () => (
<Animate
start={{ opacity: 0 }}
enter={{ opacity: [1], timing: { duration: 1000 } }}
update={{ opacity: [1] }}
leave={{ opacity: [0], timing: { duration: 1000 } }}
>
{({ opacity }) => (
<div style={{ opacity }}>
Hello, React-Move!
</div>
)}
</Animate>
);
export default MyAnimatedComponent;
Advanced Usage
React-Move allows for more complex animations by using custom interpolators and handling lifecycle events.
Understanding Interpolation
Interpolation is a crucial concept in React-Move animations. It’s the process of calculating intermediate values between two known points to create smooth transitions. For example, when animating a circle’s position from x=0 to x=100, interpolation calculates all the values in between (1, 2, 3, …, 98, 99) to create fluid movement. React-Move uses interpolation for various types of values, including numbers, colors, and SVG paths. By default, it handles numeric interpolation, but you can customize it for more complex transitions using additional libraries or custom functions.
Custom Interpolation
You can customize interpolation for attributes such as colors or transforms by using the interpolation
prop.
import React from 'react';
import { NodeGroup } from 'react-move';
import { interpolate, interpolateTransformSvg } from 'd3-interpolate';
const data = [
{ id: 1, color: '#ff0000' },
{ id: 2, color: '#00ff00' },
];
const MyAdvancedComponent: React.FC = () => (
<NodeGroup
data={data}
keyAccessor={(d) => d.id.toString()}
start={() => ({ color: '#0000ff' })}
enter={() => ({ color: ['#00ff00'], timing: { duration: 1500 } })}
update={() => ({ color: ['#ff0000'] })}
leave={() => ({ color: ['#0000ff'], timing: { duration: 1500 } })}
interpolation={(begValue, endValue, attr) => {
if (attr === 'color') {
return interpolate(begValue, endValue);
}
return interpolateTransformSvg(begValue, endValue);
}}
>
{(nodes) => (
<div>
{nodes.map(({ key, data, state }) => (
<div key={key} style={{ backgroundColor: state.color }}>
{data.color}
</div>
))}
</div>
)}
</NodeGroup>
);
export default MyAdvancedComponent;
Handling Events
React-Move supports animation lifecycle events such as start
, interrupt
, and end
.
import React from 'react';
import { NodeGroup } from 'react-move';
const data = [
{ id: 1, value: 100 },
{ id: 2, value: 200 },
];
const MyEventComponent: React.FC = () => (
<NodeGroup
data={data}
keyAccessor={(d) => d.id.toString()}
start={() => ({ opacity: 0 })}
enter={() => ({
opacity: [1],
timing: { duration: 500 },
events: {
start: () => console.log('Animation started'),
end: () => console.log('Animation ended'),
},
})}
update={() => ({ opacity: [1] })}
leave={() => ({ opacity: [0], timing: { duration: 500 } })}
>
{(nodes) => (
<div>
{nodes.map(({ key, data, state }) => (
<div key={key} style={{ opacity: state.opacity }}>
{data.value}
</div>
))}
</div>
)}
</NodeGroup>
);
export default MyEventComponent;
Conclusion
React-Move is a powerful library for creating animations in React applications. It offers flexibility and control over animations, making it a great choice for developers looking to enhance their user interfaces with smooth transitions. Whether you’re working with simple or complex animations, React-Move provides the tools you need to bring your React components to life.