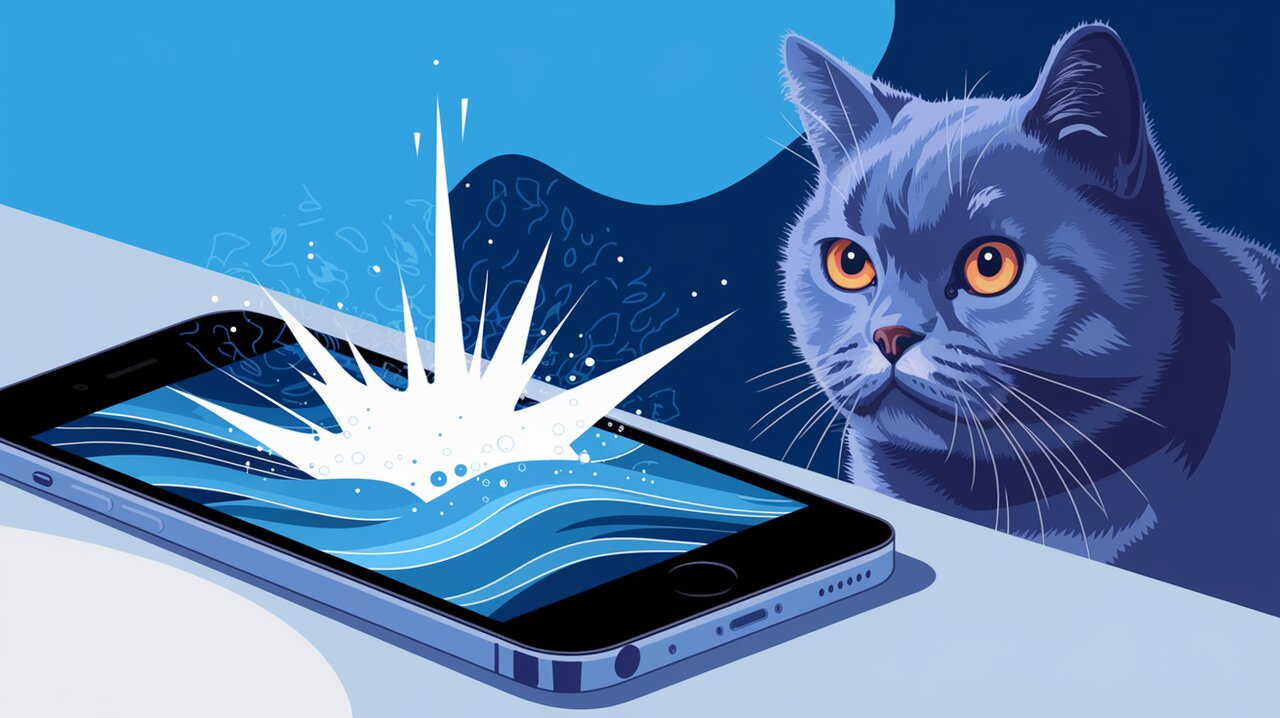
Splash into Action with react-native-bootsplash
React Native Bootsplash is a powerful library designed to enhance your app’s startup experience by providing a customizable splash screen. This initial screen not only gives users a polished first impression but also allows time for essential resources to load in the background. Whether you’re building for Android or iOS, react-native-bootsplash
simplifies the process of integrating an engaging launch screen into your React Native application.
Key Features
- Cross-Platform Support: Works seamlessly on both Android and iOS platforms.
- Customizable Design: Easily customize your splash screen’s appearance using logos and background colors.
- Efficient Asset Management: Automatically generates necessary assets for various screen sizes and resolutions.
Getting Started
To get started with react-native-bootsplash, you’ll need to install it in your React Native project. You can use either npm or yarn for this:
npm install --save react-native-bootsplash
# or
yarn add react-native-bootsplash
After installation, don’t forget to navigate to the ios
directory and run pod install
to ensure all dependencies are correctly linked.
Basic Usage
Setting Up Your Splash Screen
To create a basic splash screen, first generate the necessary assets using the CLI provided by react-native-bootsplash
. This command helps you create logos and background settings across different platforms:
npx react-native generate-bootsplash assets/logo.png --background=#FFFFFF --logo-width=100 --platforms=android,ios
Replace assets/logo.png
with the path to your logo file and adjust other parameters as needed.
Implementing in iOS
Edit your AppDelegate.mm
file to initialize the splash screen:
#import "AppDelegate.h"
#import "RNBootSplash.h" // Add this import
@implementation AppDelegate
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
[RNBootSplash initWithStoryboard:@"BootSplash" rootView:self.window.rootViewController.view];
return YES;
}
@end
Implementing in Android
Modify your MainActivity.java
or MainActivity.kt
file:
import android.os.Bundle;
import com.zoontek.rnbootsplash.RNBootSplash; // Add this import
public class MainActivity extends ReactActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
RNBootSplash.init(this); // Initialize the splash screen
super.onCreate(savedInstanceState);
}
}
Advanced Techniques
Custom Animations
For more advanced usage, you can create custom animations that transition from the splash screen to your main app view. Here’s an example using React Native’s Animated API:
import { useState } from 'react';
import { Animated } from 'react-native';
import BootSplash from 'react-native-bootsplash';
const App = () => {
const [opacity] = useState(new Animated.Value(1));
useEffect(() => {
const hideSplash = async () => {
await BootSplash.hide({ fade: true });
Animated.timing(opacity, {
toValue: 0,
duration: 500,
useNativeDriver: true,
}).start();
};
hideSplash();
}, []);
return (
<Animated.View style={{ flex: 1, opacity }}>
{/* Your app content */}
</Animated.View>
);
};
Integrating with React Navigation
If you’re using React Navigation, you can hide the splash screen once navigation is ready:
import { NavigationContainer } from '@react-navigation/native';
import BootSplash from 'react-native-bootsplash';
const App = () => (
<NavigationContainer onReady={() => BootSplash.hide()}>
{/* Navigation content */}
</NavigationContainer>
);
Wrapping Up
The react-native-bootsplash library offers a straightforward way to implement professional-looking splash screens in your React Native apps. By following these steps and examples, you can ensure that your users have a smooth and visually appealing experience right from the start. Whether you’re just getting started or looking to add complex animations, this library provides the tools you need to make it happen.