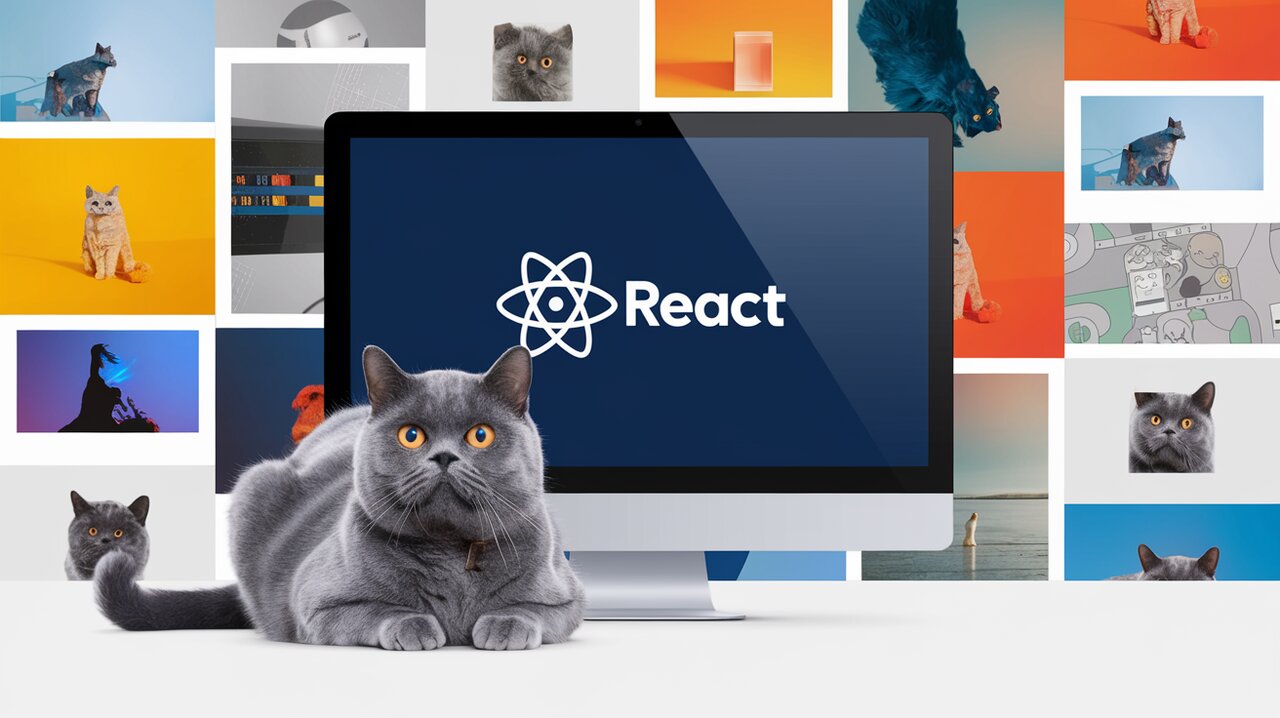
Unleash Photo Gallery Magic with React Photo Album
React Photo Album is a powerful library that allows developers to create responsive and visually appealing photo galleries with ease. Whether you’re building a personal portfolio, an e-commerce site, or a social media platform, this library offers a range of features to showcase your images in style. Let’s dive into the world of React Photo Album and explore how it can elevate your web development projects.
Features That Make React Photo Album Shine
React Photo Album comes packed with features that set it apart from other photo gallery libraries:
- Multiple Layout Options: Choose from rows, columns, or masonry layouts to best suit your design needs.
- Responsive Design: Automatically adjusts to different screen sizes for a seamless user experience.
- Server-Side Rendering (SSR) Support: Ensures your gallery looks great even before JavaScript loads.
- Customizable Components: Tailor the gallery to your specific requirements with custom render functions.
- TypeScript Support: Enjoy the benefits of type checking for a more robust development experience.
Getting Started with React Photo Album
To begin your journey with React Photo Album, you’ll first need to install the package. Open your terminal and run:
npm install react-photo-album
Once installed, you can import the component you need based on your desired layout:
import { RowsPhotoAlbum } from "react-photo-album";
import "react-photo-album/rows.css";
Creating Your First Photo Gallery
Let’s create a simple photo gallery using the rows layout:
import React from "react";
import { RowsPhotoAlbum } from "react-photo-album";
import "react-photo-album/rows.css";
const photos = [
{ src: "/image1.jpg", width: 800, height: 600 },
{ src: "/image2.jpg", width: 1600, height: 900 },
// Add more photos here
];
const Gallery = () => {
return <RowsPhotoAlbum photos={photos} />;
};
export default Gallery;
This basic setup will create a responsive gallery with your photos arranged in rows. The photos
array contains objects with the source URL, width, and height of each image.
Advanced Usage: Customizing Your Gallery
React Photo Album offers extensive customization options. Let’s explore some advanced features:
Responsive Columns
For a columns layout that adjusts based on screen width:
import { ColumnsPhotoAlbum } from "react-photo-album";
const Gallery = () => {
return (
<ColumnsPhotoAlbum
photos={photos}
columns={(containerWidth) => {
if (containerWidth < 400) return 1;
if (containerWidth < 800) return 2;
return 3;
}}
/>
);
};
Adding Click Handlers
Implement interactivity by adding click handlers to your photos:
const Gallery = () => {
const handleClick = ({ index }) => {
console.log(`Photo ${index} clicked!`);
// Add your logic here, e.g., opening a lightbox
};
return <RowsPhotoAlbum photos={photos} onClick={handleClick} />;
};
Custom Rendering
For complete control over how your photos are displayed, use custom render functions:
const Gallery = () => {
return (
<RowsPhotoAlbum
photos={photos}
render={{
photo: ({ photo, wrapperStyle, imageProps }) => (
<div style={wrapperStyle}>
<img {...imageProps} alt={photo.alt} />
<div className="photo-overlay">{photo.title}</div>
</div>
),
}}
/>
);
};
Optimizing for Performance
React Photo Album is built with performance in mind. Here are some tips to ensure your gallery runs smoothly:
-
Use Responsive Images: Provide multiple image sizes in the
srcSet
property to allow browsers to choose the most appropriate size. -
Implement Lazy Loading: React Photo Album supports lazy loading out of the box. Ensure you’re not loading all images at once for larger galleries.
-
Utilize Breakpoints: Set breakpoints to reduce unnecessary layout calculations during window resizing.
<RowsPhotoAlbum photos={photos} breakpoints={[300, 600, 1200]} />
Server-Side Rendering Considerations
When implementing Server-Side Rendering, you might encounter challenges with layout calculations. React Photo Album provides solutions:
-
Default Container Width: Specify a
defaultContainerWidth
for consistent initial rendering. -
Skeleton Component: Use a skeleton component while the actual gallery loads:
<RowsPhotoAlbum
photos={photos}
skeleton={<div style={{ width: "100%", height: "600px" }} />}
/>
- SSR Component: For the most advanced SSR support, use the experimental
UnstableSSR
component:
import { UnstableSSR as SSR } from "react-photo-album/ssr";
<SSR breakpoints={[300, 600, 900, 1200]}>
<RowsPhotoAlbum photos={photos} />
</SSR>
Conclusion
React Photo Album offers a powerful and flexible solution for creating beautiful photo galleries in your React applications. With its range of layouts, responsive design, and extensive customization options, you can create galleries that not only look great but also perform well across different devices.
As you continue to explore React Photo Album, you might find it helpful to check out related articles on ReactLibs.dev. For instance, the article on React Image Gallery offers insights into another popular image gallery library, which could provide additional perspectives on implementing visual components in React. Additionally, for those interested in optimizing image loading, the React Lazy Load Image Component Guide could be a valuable resource to complement your use of React Photo Album.
By leveraging the power of React Photo Album and staying informed about complementary libraries and techniques, you’ll be well-equipped to create stunning, performant photo galleries that enhance the visual appeal of your React applications.