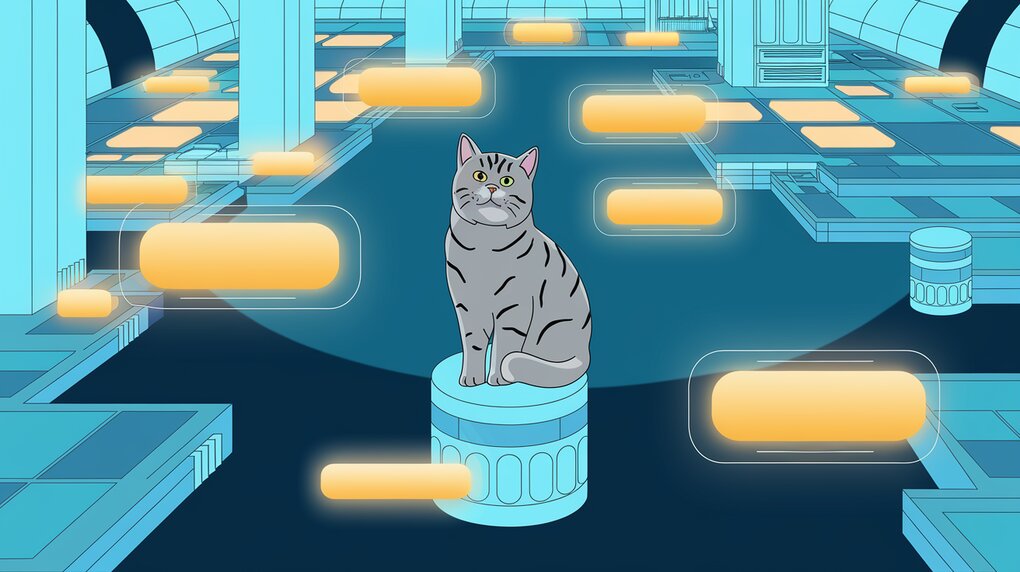
Introduction
React Placeholder is a powerful library that allows developers to enhance the user experience of their React applications by displaying placeholders while content is loading. This library is designed to replicate your page with nice placeholders, making it easier for users to understand that the content is still being loaded. You can use placeholders from the default set or create your own custom placeholders to fit your application’s needs.
Features
React Placeholder offers several key features that make it a valuable addition to your React projects:
- Dynamic Placeholders: Easily display placeholders while content is loading or if the source fails.
- Customization: Use built-in placeholders or create your own custom placeholders to fit your application’s design.
- Animation: Comes with a default pulse animation to better convey the loading state.
- Delay Control: Control the delay before displaying the placeholder to avoid brief flashes on faster connections.
- Styling: Style placeholders using CSS classes or inline styles.
Installation
To install React Placeholder, you can use npm or yarn:
npm install --save react-placeholder
yarn add react-placeholder
Basic Usage
Here is a simple example of how to use React Placeholder:
import ReactPlaceholder from 'react-placeholder';
import "react-placeholder/lib/reactPlaceholder.css";
function MyComponent() {
const [ready, setReady] = useState(false);
return (
<div>
<ReactPlaceholder type='media' rows={7} ready={ready}>
<MyComponent />
</ReactPlaceholder>
</div>
);
}
In this example, we import the ReactPlaceholder
component and use it to display a placeholder while the content is loading. The type
prop specifies the type of placeholder to display, and the rows
prop sets the number of rows. The ready
prop is used to control when the placeholder should be displayed.
Advanced Usage
Here is an example of how to use custom placeholders:
import ReactPlaceholder from 'react-placeholder';
import "react-placeholder/lib/reactPlaceholder.css";
function MyComponent() {
const [ready, setReady] = useState(false);
return (
<div>
<ReactPlaceholder ready={ready} customPlaceholder={<MyCustomPlaceholder />}>
<MyComponent />
</ReactPlaceholder>
</div>
);
}
function MyCustomPlaceholder() {
return (
<div className='my-awesome-placeholder'>
<RectShape color='blue' style={{ width: 30, height: 80 }} />
<TextBlock rows={7} color='yellow' />
</div>
);
}
In this example, we create a custom placeholder component MyCustomPlaceholder
and pass it to the customPlaceholder
prop of ReactPlaceholder
. This allows us to display a custom placeholder instead of the default one.
Conclusion
React Placeholder is a versatile library that can significantly improve the user experience of your React applications. By using placeholders, you can provide a more engaging and informative experience for your users, making it clear that content is still being loaded. With its customization options and animation support, React Placeholder is a valuable tool for any React developer.