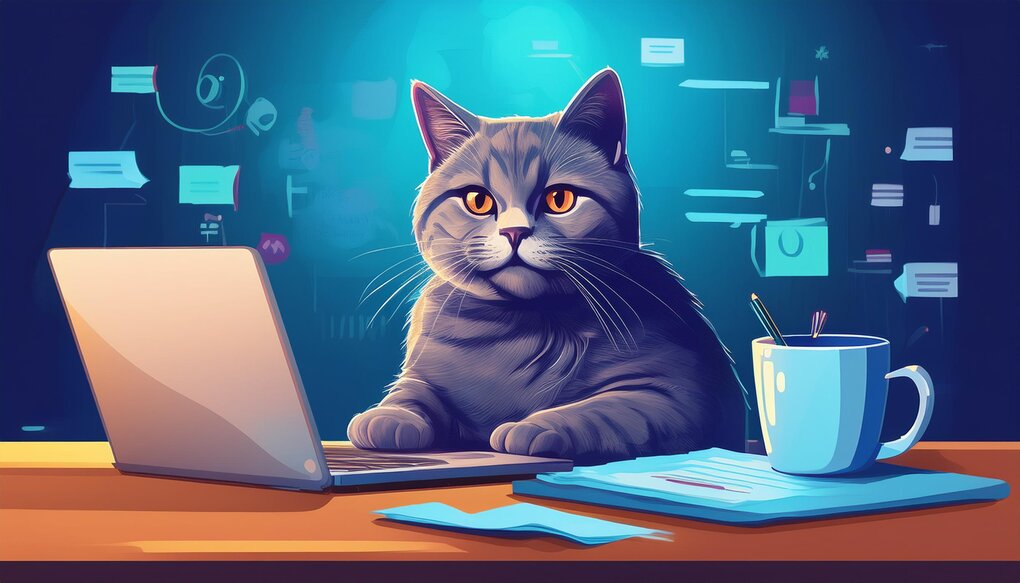
React Pro Sidebar is a powerful and lightweight library that allows developers to create responsive, collapsible, and customizable dropdown menus and unlimited nested submenus for web applications. It provides a set of pre-built components and APIs that can be used to manage the sidebar.
Installation
To get started, you need to install the react-pro-sidebar
library. You can do this using npm or yarn.
Using npm
npm install react-pro-sidebar
Using yarn
yarn add react-pro-sidebar
Basic Usage
Here is a simple example of how to use React Pro Sidebar:
import { Sidebar, Menu, MenuItem, SubMenu } from 'react-pro-sidebar';
function App() {
return (
<Sidebar>
<Menu>
<SubMenu label="Charts">
<MenuItem> Pie charts </MenuItem>
<MenuItem> Line charts </MenuItem>
</SubMenu>
<MenuItem> Documentation </MenuItem>
<MenuItem> Calendar </MenuItem>
</Menu>
</Sidebar>
);
}
export default App;
Advanced Usage
Using React Router
You can integrate React Router links into your sidebar using the component
prop:
import { Sidebar, Menu, MenuItem } from 'react-pro-sidebar';
import { Link } from 'react-router-dom';
function App() {
return (
<Sidebar>
<Menu
menuItemStyles={{
button: {
// the active class will be added automatically by react router
// so we can use it to style the active menu item
[`&.active`]: {
backgroundColor: '#13395e',
color: '#b6c8d9',
},
},
}}
>
<MenuItem component={<Link to="/documentation" />}> Documentation</MenuItem>
<MenuItem component={<Link to="/calendar" />}> Calendar</MenuItem>
<MenuItem component={<Link to="/e-commerce" />}> E-commerce</MenuItem>
</Menu>
</Sidebar>
);
}
export default App;
Customization
You can customize the styles of the sidebar and its components using the rootStyles
prop. For example:
import { Sidebar, Menu, MenuItem } from 'react-pro-sidebar';
function App() {
return (
<Sidebar
rootStyles={{
[`.${sidebarClasses.container}`]: {
backgroundColor: 'red',
},
}}
>
<Menu>
// ...
</Menu>
</Sidebar>
);
}
export default App;
Conclusion
React Pro Sidebar is a versatile library that allows you to create highly customizable and responsive sidebars for your React applications. With its ability to integrate with React Router and provide extensive customization options, it is an excellent choice for building navigation systems that adapt to different screen sizes and user needs.