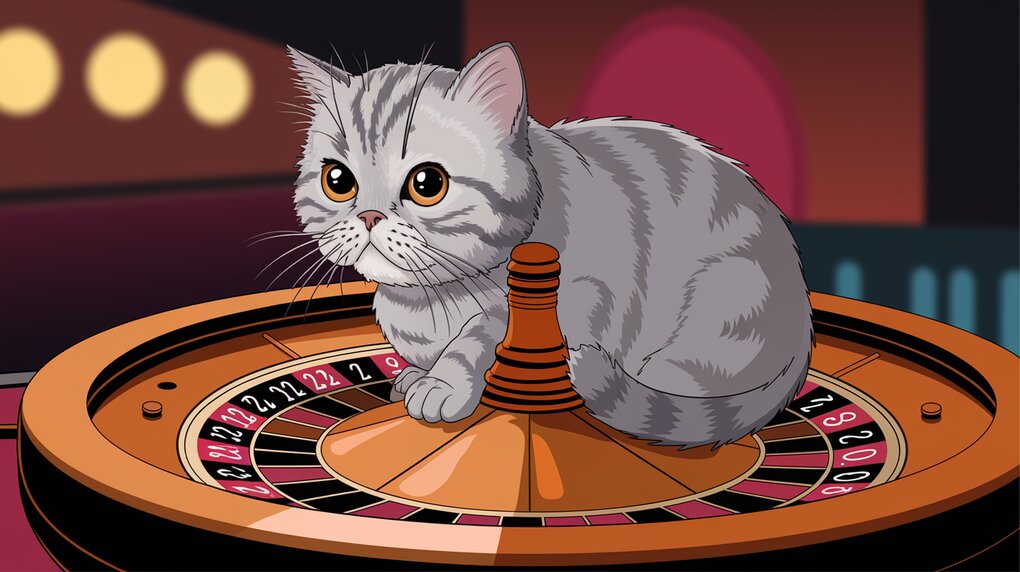
React Roulette Pro: A Comprehensive Guide for Developers
Introduction
React Roulette Pro is a robust and customizable library designed to create roulette wheels in React applications. This library offers a variety of features, including optimization, customization, and ease of use. In this guide, we will delve into the features, installation, and usage of React Roulette Pro, making it easier for developers to integrate and customize this library.
Features
React Roulette Pro boasts several impressive features that make it an excellent choice for developers:
- Optimization: The library is optimized for performance, ensuring that your roulette wheel runs smoothly and efficiently.
- Customization: React Roulette Pro allows for extensive customization, enabling you to tailor the appearance and behavior of your roulette wheel to fit your specific needs.
- Design Plugins Architecture: The library supports a design plugins architecture, allowing you to create and use custom design plugins to enhance the look and feel of your roulette wheel.
- Two Types of Roulette: React Roulette Pro supports both horizontal and vertical roulette types, providing flexibility in how you display your roulette wheel.
- Powerful and Lightweight: The library is powerful yet lightweight, making it easy to integrate into your React application without adding significant bulk.
- Ease of Use: React Roulette Pro is designed to be easy to use, with a straightforward API and minimal setup required.
- NextJS, GatsbyJS, and RemixJS Support: The library is compatible with popular React frameworks like NextJS, GatsbyJS, and RemixJS, making it versatile and adaptable.
Installation
To install React Roulette Pro, you can use npm or yarn:
# Via npm:
npm i react-roulette-pro
# Via yarn:
yarn add react-roulette-pro
Basic Usage
Here is a basic example of how to use React Roulette Pro in your React application:
import { useState } from 'react';
import RoulettePro from 'react-roulette-pro';
import 'react-roulette-pro/dist/index.css';
const prizes = [
{
image: 'https://i.ibb.co/6Z6Xm9d/good-1.png',
},
{
image: 'https://i.ibb.co/T1M05LR/good-2.png',
},
{
image: 'https://i.ibb.co/Qbm8cNL/good-3.png',
},
{
image: 'https://i.ibb.co/5Tpfs6W/good-4.png',
},
{
image: 'https://i.ibb.co/64k8D1c/good-5.png',
},
];
const winPrizeIndex = 0;
const reproductionArray = (array = [], length = 0) => [
...Array(length)
.fill('_')
.map(() => array[Math.floor(Math.random() * array.length)]),
];
const reproducedPrizeList = [
...prizes,
...reproductionArray(prizes, prizes.length * 3),
...prizes,
...reproductionArray(prizes, prizes.length),
];
const generateId = () =>
`${Date.now().toString(36)}-${Math.random().toString(36).substring(2)}`;
const prizeList = reproducedPrizeList.map((prize) => ({
...prize,
id: typeof crypto.randomUUID === 'function' ? crypto.randomUUID() : generateId(),
}));
const App = () => {
const [start, setStart] = useState(false);
const prizeIndex = prizes.length * 4 + winPrizeIndex;
const handleStart = () => {
setStart((prevState) => !prevState);
};
const handlePrizeDefined = () => {
console.log('🥳 Prize defined 🥳');
};
return (
<>
<RoulettePro
prizes={prizeList}
prizeIndex={prizeIndex}
start={start}
onPrizeDefined={handlePrizeDefined}
/>
<button onClick={handleStart}>Start</button>
</>
);
};
export default App;
Advanced Usage
Customizing the Roulette
React Roulette Pro allows for extensive customization. Here are some examples of how you can customize the roulette:
- Passing a
prizeItemRenderFunction
: You can pass a function to render the prize items yourself.
<RoulettePro
prizes={prizeList}
prizeIndex={prizeIndex}
start={start}
onPrizeDefined={handlePrizeDefined}
prizeItemRenderFunction={(item) => (
<div>
<img src={item.image} alt={item.image} />
<p>{item.text}</p>
</div>
)}
/>
- Using a Design Plugin: You can use a design plugin to customize the appearance of your roulette wheel.
import { gracefulLinesPlugin } from 'rrp-graceful-lines-plugin';
<RoulettePro
prizes={prizeList}
prizeIndex={prizeIndex}
start={start}
onPrizeDefined={handlePrizeDefined}
designPlugin={gracefulLinesPlugin}
/>
- Passing Custom Classes: You can pass custom classes to style your roulette wheel.
<RoulettePro
prizes={prizeList}
prizeIndex={prizeIndex}
start={start}
onPrizeDefined={handlePrizeDefined}
classes={{
wrapper: 'custom-wrapper',
prizeListWrapper: 'custom-prize-list-wrapper',
prizeItem: 'custom-prize-item',
}}
/>
Using React Roulette Pro with SSR
React Roulette Pro can be used with Server-Side Rendering (SSR) without issues. Here is an example of how to use it with NextJS:
import dynamic from 'next/dynamic';
const RoulettePro = dynamic(() => import('react-roulette-pro'), {
ssr: false,
});
const App = () => {
// Your code here
return (
<>
<RoulettePro
prizes={prizeList}
prizeIndex={prizeIndex}
start={start}
onPrizeDefined={handlePrizeDefined}
/>
<button onClick={handleStart}>Start</button>
</>
);
};
export default App;
Conclusion
React Roulette Pro is a powerful and customizable library for creating roulette wheels in React applications. With its optimization, customization, and ease of use, it is an excellent choice for developers looking to add a roulette wheel to their applications. By following the examples provided in this guide, you can easily integrate and customize React Roulette Pro to fit your specific needs.