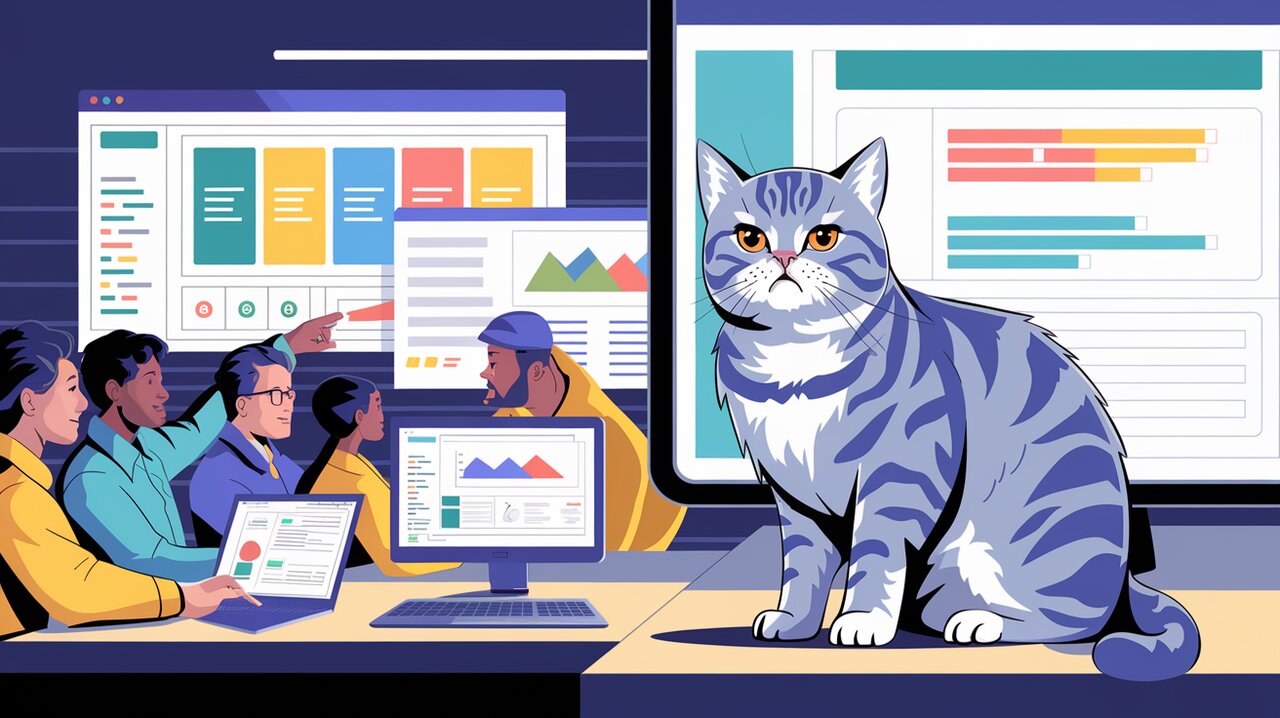
React Smart Data Table: Supercharge Your React Apps with Effortless Data Visualization
React Smart Data Table is a powerful and flexible component designed to simplify the process of displaying and managing data in React applications. With its “zero configuration” approach, this library allows developers to quickly implement feature-rich data tables without the need for extensive setup or customization.
Key Features
React Smart Data Table comes packed with an impressive array of features that cater to various data presentation needs:
- Automatic Column Generation: Creates human-readable column names based on object keys
- Sortable Columns: Allows users to sort data with support for custom sorting functions
- Filtering and Searching: Enables users to filter and search through data easily
- Search Highlighting: Highlights search terms in the results for better visibility
- Column Visibility Toggles: Gives users control over which columns to display
- Pagination: Automatically splits large datasets into manageable pages
- Server-Side Data Support: Handles remote data sources efficiently
- Row Click Handling: Provides control over row click events
- Smart Data Rendering: Automatically formats URLs, email addresses, boolean values, and image links
- Custom Column Overrides: Allows for specific column behavior customization
- Custom Components: Supports custom pagination components
- Column Order Control: Enables developers to specify the order and visibility of columns
Getting Started
Installation
To begin using React Smart Data Table in your project, you can install it via npm or yarn:
npm install react-smart-data-table
# or
yarn add react-smart-data-table
After installation, you may want to import the basic styling provided by the library. While this step is optional, it can help you get started quickly:
import 'react-smart-data-table/dist/react-smart-data-table.css'
Basic Usage
Let’s dive into a simple example to demonstrate how easy it is to use React Smart Data Table:
import React from 'react'
import SmartDataTable from 'react-smart-data-table'
const YourComponent = () => {
const data = [
{ id: 1, name: 'John Doe', email: 'john@example.com', age: 30 },
{ id: 2, name: 'Jane Smith', email: 'jane@example.com', age: 25 },
// ... more data
]
return (
<SmartDataTable
data={data}
name="test-table"
className="ui compact selectable table"
sortable
/>
)
}
In this example, we’re creating a basic table with sortable columns. The SmartDataTable
component automatically generates the table structure based on the provided data.
Customizing Column Headers
You can easily customize column headers using the headers
prop:
const headers = {
id: 'ID',
name: 'Full Name',
email: 'Email Address',
age: 'Age (years)',
}
<SmartDataTable
data={data}
headers={headers}
name="custom-header-table"
/>
This allows you to provide more descriptive or user-friendly column names.
Advanced Usage
Custom Sorting
React Smart Data Table allows you to implement custom sorting logic for specific columns:
const customSort = {
age: (a, b) => b - a, // Sort age in descending order
}
<SmartDataTable
data={data}
sortable
customSort={customSort}
name="custom-sort-table"
/>
Filtering and Searching
Enabling filtering and searching is straightforward:
<SmartDataTable
data={data}
filterValue="John"
searchable
name="searchable-table"
/>
This will display only rows containing “John” and allow users to perform further searches.
Custom Data Rendering
For columns that require special rendering, you can use the parseImg
and parseBool
props:
<SmartDataTable
data={data}
parseImg={{
imgWrapper: (url) => <img src={url} alt="User" className="user-avatar" />,
className: 'image-column',
}}
parseBool={{
yesWord: 'Active',
noWord: 'Inactive',
}}
name="custom-render-table"
/>
This example customizes the rendering of image URLs and boolean values.
Server-Side Data Fetching
React Smart Data Table supports server-side data fetching, which is crucial for handling large datasets:
const [data, setData] = useState([])
const [loading, setLoading] = useState(true)
useEffect(() => {
const fetchData = async () => {
setLoading(true)
const response = await fetch('https://api.example.com/data')
const result = await response.json()
setData(result)
setLoading(false)
}
fetchData()
}, [])
return (
<SmartDataTable
data={data}
loading={loading}
name="server-side-table"
/>
)
This setup allows the table to display a loading state while fetching data from a remote source.
Leveraging Context
React Smart Data Table provides a context that you can access in your custom components. This is particularly useful when creating custom empty table messages or loading indicators:
import { useSmartDataTableContext } from 'react-smart-data-table'
const CustomEmptyTable = () => {
const { columns, data } = useSmartDataTableContext()
return (
<div>
<p>No data available for {columns.length} columns.</p>
<p>Total rows: {data.length}</p>
</div>
)
}
<SmartDataTable
data={data}
emptyTable={CustomEmptyTable}
name="context-example-table"
/>
This example demonstrates how to use the context to create a custom component for when the table is empty.
Conclusion
React Smart Data Table offers a powerful, flexible, and easy-to-use solution for displaying data in React applications. Its “zero configuration” approach, combined with a rich set of features and customization options, makes it an excellent choice for developers looking to quickly implement sophisticated data tables.
By leveraging its automatic column generation, sorting capabilities, filtering and searching functions, and custom rendering options, you can create highly interactive and user-friendly data presentations with minimal effort. Whether you’re working with small local datasets or large server-side data sources, React Smart Data Table provides the tools you need to build efficient and responsive data-driven interfaces.
As you continue to explore the library’s capabilities, you’ll find that it can adapt to a wide range of use cases, from simple data displays to complex, interactive data management systems. With React Smart Data Table, you’re well-equipped to tackle any data presentation challenge in your React projects.