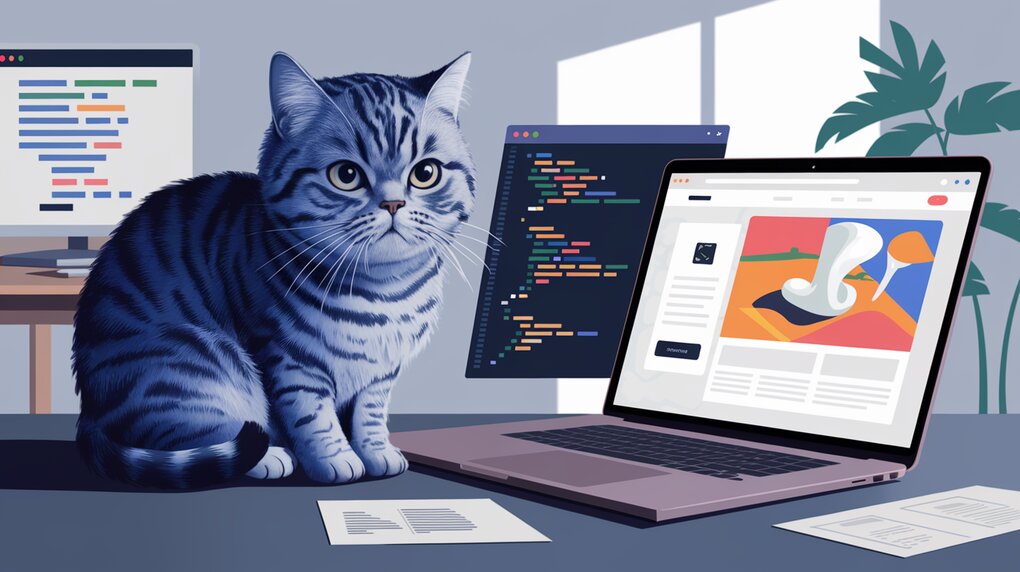
React Smooth is a powerful animation library for React that allows you to create smooth and interactive animations for your React components. In this article, we’ll explore the basics of using react-smooth, including installation, basic usage, and advanced scenarios.
Installation
To install react-smooth, you can use npm or yarn:
npm install --save react-smooth
yarn add react-smooth
Basic Usage
Let’s start with a simple animation example. Suppose we want to animate a div element to change its opacity from 0 to 1. We can use the Animate
component from react-smooth:
import React from 'react';
import { Animate } from 'react-smooth';
const App = () => {
return (
<Animate to={{ opacity: 1 }} from={{ opacity: 0 }} attributeName="opacity">
<div style={{ opacity: 0 }}>Hello World!</div>
</Animate>
);
};
In this example, we’re using the Animate
component to animate the div element. We’re setting the to
property to { opacity: 1 }
to specify the final opacity value, and the from
property to { opacity: 0 }
to specify the initial opacity value. The attributeName
property is set to "opacity"
to specify the style property we want to animate.
Advanced Usage
Now let’s look at some more advanced scenarios. Suppose we want to animate a div element to change its opacity and position over a period of time. We can use the steps
property to define the animation keyframes:
import React from 'react';
import { Animate } from 'react-smooth';
const steps = [
{
style: {
opacity: 0,
},
duration: 400,
},
{
style: {
opacity: 1,
transform: 'translate(0, 0)',
},
duration: 1000,
},
{
style: {
transform: 'translate(100px, 100px)',
},
duration: 1200,
},
];
const App = () => {
return (
<Animate steps={steps}>
<div>Hello World!</div>
</Animate>
);
};
In this example, we’re using the steps
property to define the animation keyframes. Each step object contains a style
property that specifies the style values for that step, and a duration
property that specifies the duration of that step.
Conclusion
In this article, we’ve explored the basics of using react-smooth, including installation, basic usage, and advanced scenarios. We’ve seen how to create simple animations using the Animate
component, and how to create more complex animations using the steps
property. With react-smooth, you can create smooth and interactive animations for your React components, and take your user experience to the next level.