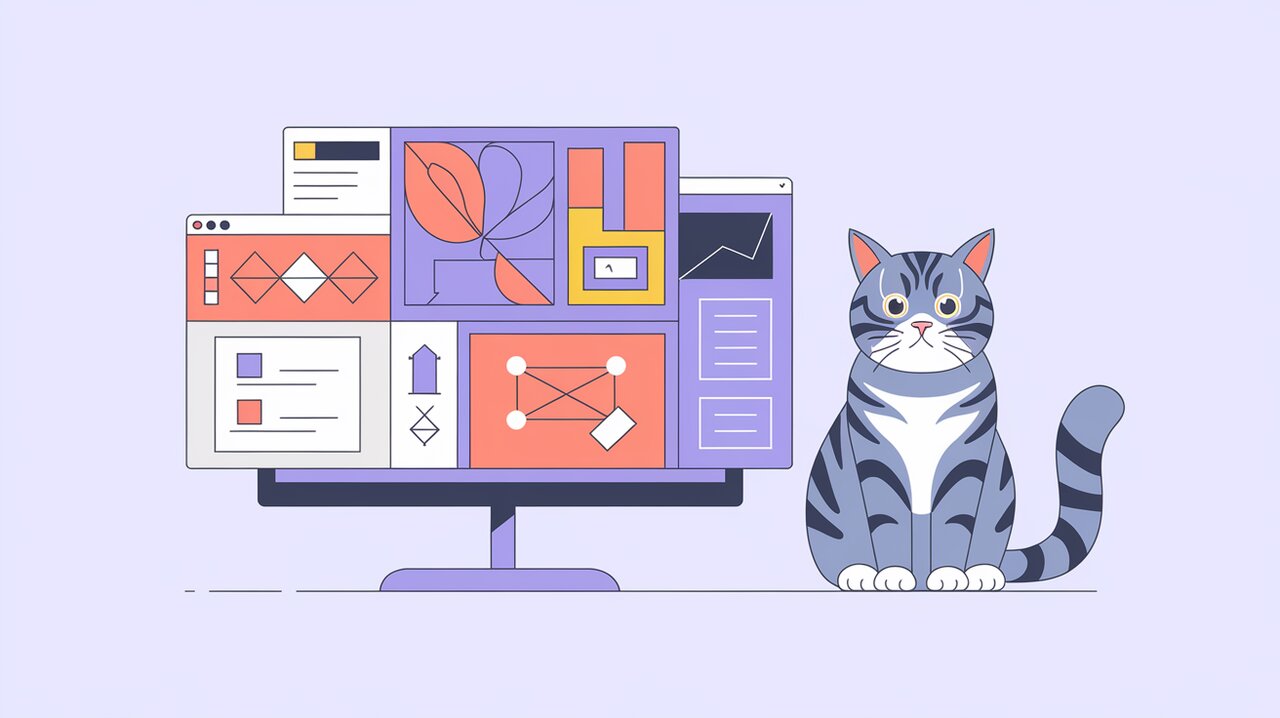
Subdivide is a powerful library for React that allows developers to create dynamic split-pane layouts effortlessly. This tool is designed for those who need a flexible interface that can be customized to fit specific workflows. With Subdivide, you can easily subdivide panes horizontally or vertically, resize them by dragging edges, and even merge them by dragging corners onto adjacent panes. This makes it ideal for applications where users need to define their own layout, such as dashboards or complex data visualization tools.
Key Features of Subdivide
- Infinite Subdivision: Panes can be divided infinitely, allowing for complex layout designs.
- Flexible Orientation: Supports both horizontal and vertical subdivisions.
- Interactive Resizing: Users can resize panes by dragging edges or corners.
- Component Integration: Easily assign any component to any pane.
Getting Started with Installation
To start using Subdivide, you need to install it via npm or yarn:
npm install subdivide
Or if you prefer yarn:
yarn add subdivide
After installation, you can use WebPack or Browserify to import the library into your project.
Basic Usage Examples
Setting Up a Simple Split-Pane Layout
To create a basic split-pane layout using Subdivide, you first need to import the component and use it within your React application:
import React from 'react';
import { Subdivide } from 'subdivide';
const MyLayout = () => (
<Subdivide>
<div>Pane 1</div>
<div>Pane 2</div>
</Subdivide>
);
export default MyLayout;
In this example, Subdivide
acts as a container that automatically manages the layout of its children components.
Connecting Subdivide with Redux
Subdivide integrates seamlessly with Redux for state management. Here’s how you can connect it:
import React from 'react';
import { createStore, combineReducers } from 'redux';
import { Provider, connect } from 'react-redux';
import { Subdivide, reducer as subdivideReducer } from 'subdivide';
const rootReducer = combineReducers({
subdivide: subdivideReducer,
});
const store = createStore(rootReducer);
const ConnectedSubdivide = connect(state => ({
subdivide: state.subdivide,
}))(Subdivide);
const App = () => (
<Provider store={store}>
<ConnectedSubdivide />
</Provider>
);
export default App;
Here, Subdivide
is connected to the Redux store, allowing you to manage its state alongside other application states.
Advanced Techniques
Customizing Pane Content
You can assign custom components to each pane in your layout:
import React from 'react';
import { Subdivide } from 'subdivide';
const CustomComponent = () => <div>Custom Content</div>;
const AdvancedLayout = () => (
<Subdivide>
<CustomComponent />
<div>Another Pane</div>
</Subdivide>
);
export default AdvancedLayout;
This flexibility allows you to integrate complex components into your layout seamlessly.
Dynamic Pane Management
For more interactive applications, you might want to dynamically add or remove panes based on user actions:
import React, { useState } from 'react';
import { Subdivide } from 'subdivide';
const DynamicLayout = () => {
const [panes, setPanes] = useState(['Pane 1', 'Pane 2']);
const addPane = () => {
setPanes([...panes, `Pane ${panes.length + 1}`]);
};
return (
<div>
<button onClick={addPane}>Add Pane</button>
<Subdivide>
{panes.map((paneContent) => (
<div key={paneContent}>{paneContent}</div>
))}
</Subdivide>
</div>
);
};
export default DynamicLayout;
This example demonstrates how you can manage panes dynamically by updating the state in response to user interactions.
Wrapping Up
The Subdivide library provides a robust solution for creating customizable split-pane layouts in React applications. Its ability to integrate with Redux and support dynamic content makes it an excellent choice for developers looking to build interactive and user-defined interfaces. Whether you’re developing a dashboard or a complex data visualization tool, Subdivide offers the flexibility and power needed to enhance your application’s user experience.