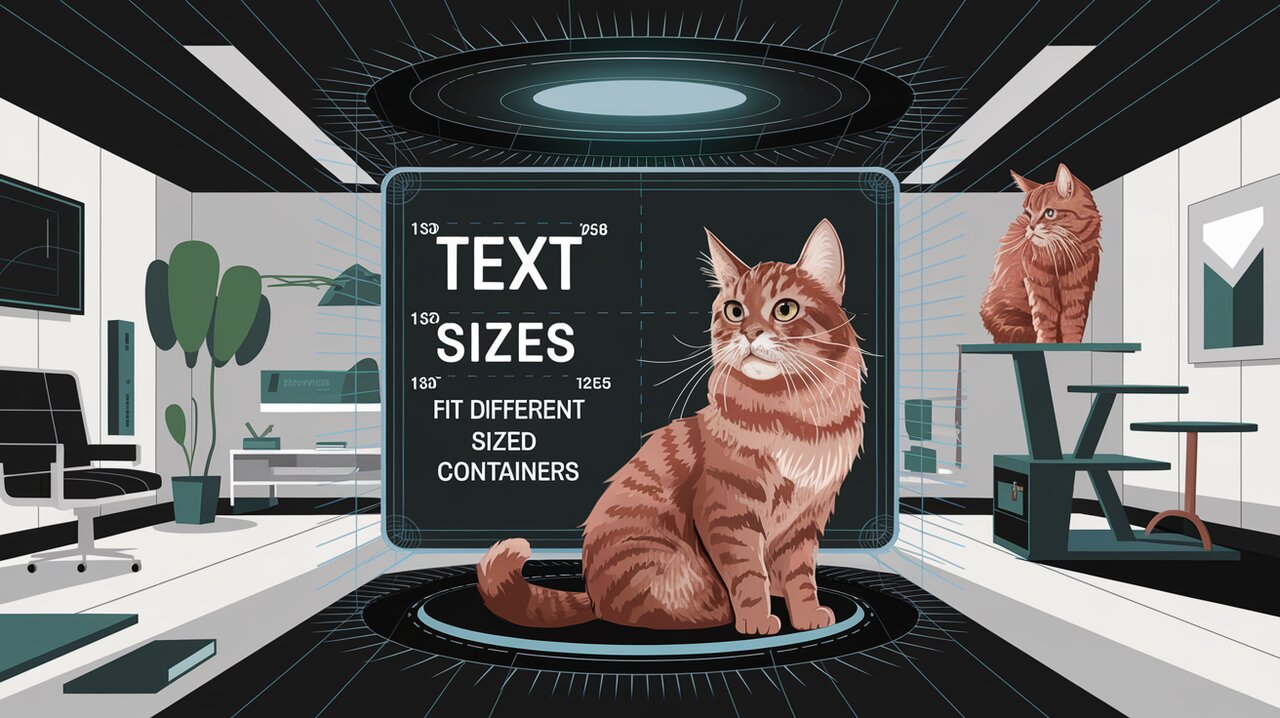
React-Textfit: Unleash the Magic of Responsive Text in React
React Textfit is a powerful library that brings responsive typography to your React applications with ease. By automatically adjusting font sizes to fit within specified containers, it eliminates the need for complex media queries or manual size adjustments. Let’s dive into how you can harness this tool to create sleek, responsive text components.
Features
React Textfit offers several key features that make it stand out:
- Efficient binary search algorithm for fast text fitting
- Support for both single-line and multi-line text
- Customizable minimum and maximum font sizes
- Compatibility with any CSS style configuration
- Responsive to window resizing
Installation
To get started with react-textfit
, you’ll need to install it in your React project. You can do this using npm or yarn:
npm install react-textfit --save
or
yarn add react-textfit
Basic Usage
Single-Line Text Fitting
For headlines or short phrases where you want the text to fit on a single line, you can use the ‘single’ mode:
import React from 'react';
import { Textfit } from 'react-textfit';
const SingleLineExample: React.FC = () => {
return (
<Textfit mode="single" max={48}>
This headline will fit perfectly!
</Textfit>
);
};
In this example, the text will adjust its size to fit the width of its container, with a maximum font size of 48 pixels.
Multi-Line Text Fitting
For longer text blocks or paragraphs, use the ‘multi’ mode:
import React from 'react';
import { Textfit } from 'react-textfit';
const MultiLineExample: React.FC = () => {
return (
<Textfit mode="multi" min={14} max={30}>
This paragraph will adjust its font size to fit within the container,
wrapping onto multiple lines as needed. It's perfect for responsive
design where you want to ensure readability across different screen sizes.
</Textfit>
);
};
This component will adjust the font size between 14 and 30 pixels to ensure the text fits within its container, allowing for multiple lines.
Advanced Usage
Respecting Container Height
When using the ‘single’ mode, you might want to ensure that the text respects both the width and height of its container. You can achieve this by setting forceSingleModeWidth
to false
:
import React from 'react';
import { Textfit } from 'react-textfit';
const HeightAwareExample: React.FC = () => {
return (
<div style={{ width: '300px', height: '100px', border: '1px solid black' }}>
<Textfit mode="single" forceSingleModeWidth={false}>
This text will respect both width and height!
</Textfit>
</div>
);
};
Custom Styling
React Textfit works seamlessly with custom styles. You can apply styles directly to the Textfit component:
import React from 'react';
import { Textfit } from 'react-textfit';
const StyledExample: React.FC = () => {
return (
<Textfit
mode="single"
max={40}
style={{
backgroundColor: '#f0f0f0',
padding: '10px',
borderRadius: '5px',
fontWeight: 'bold',
color: '#333'
}}
>
Stylish and responsive!
</Textfit>
);
};
Handling Resize Events
React Textfit automatically adjusts to window resize events, but you can control the throttle rate:
import React from 'react';
import { Textfit } from 'react-textfit';
const ThrottledExample: React.FC = () => {
return (
<Textfit mode="multi" throttle={100}>
This text will adjust its size, but not more than once every 100ms during resizing.
</Textfit>
);
};
Conclusion
React Textfit offers a powerful solution for creating responsive typography in React applications. By automatically adjusting font sizes to fit their containers, it simplifies the process of creating adaptable layouts. Whether you’re working on a complex dashboard or a simple landing page, react-textfit
can help ensure your text looks great on any device.
Remember to experiment with different modes, size limits, and styling options to achieve the perfect balance between aesthetics and readability in your responsive designs. Happy coding!