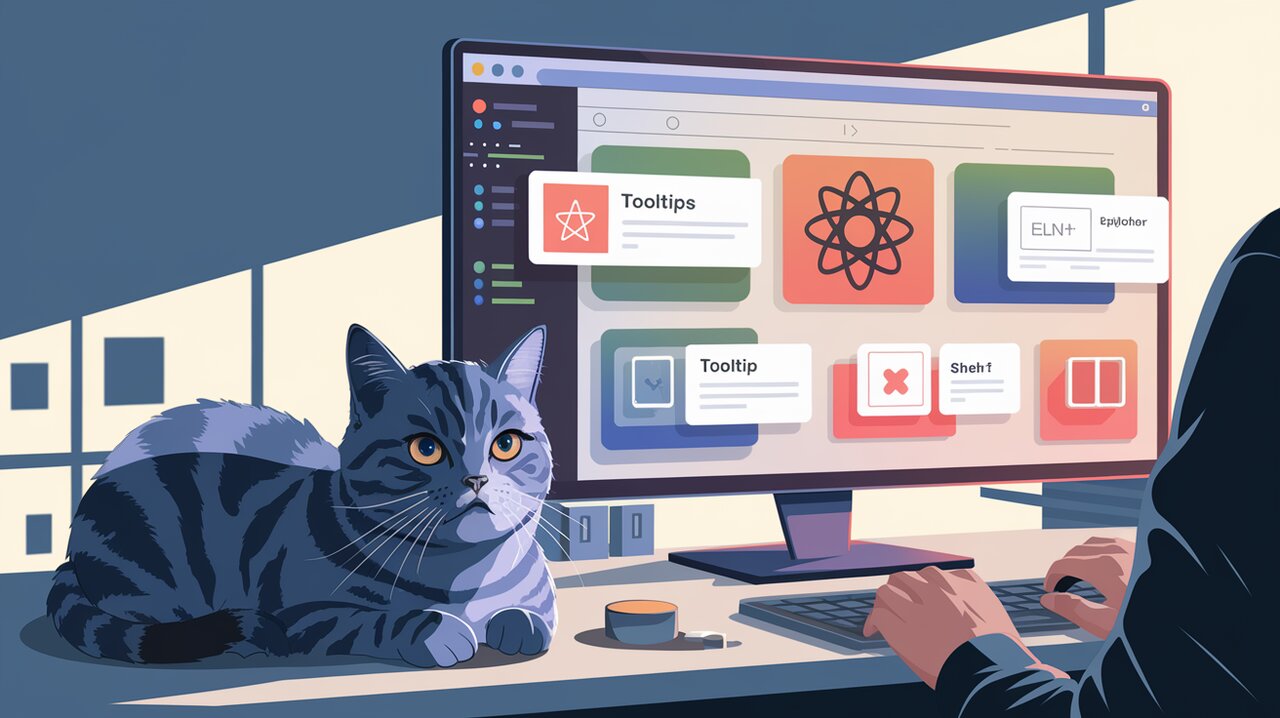
React Tippy: Elevate Your UI with Effortless Tooltips
React Tippy is a lightweight and powerful tooltip library designed specifically for React applications. Built on top of Tippy.js and powered by Popper.js, it provides developers with an easy-to-use solution for adding informative and interactive tooltips to their user interfaces. Whether you’re looking to enhance your UI with simple hover tooltips or create more complex interactive popovers, React Tippy offers a wide range of customization options to suit your needs.
Features
React Tippy comes packed with a variety of features that make it a versatile choice for developers:
- Easy Integration: Seamlessly integrates with React components
- Customizable Appearance: Offers various themes, sizes, and animations
- Flexible Positioning: Supports multiple tooltip positions and automatic repositioning
- Interactive Tooltips: Allows for creation of interactive popovers
- Performance Optimized: Lightweight and efficient, ensuring smooth user experience
- Accessibility: Supports keyboard navigation and screen readers
Installation
To get started with React Tippy, you’ll need to install it in your React project. You can do this using either npm or yarn.
Using npm:
npm install react-tippy
Using yarn:
yarn add react-tippy
After installation, you’ll need to import the CSS file in your main application file or component:
import 'react-tippy/dist/tippy.css';
Basic Usage
Let’s dive into some basic examples of how to use React Tippy in your React components.
Simple Tooltip
To create a simple tooltip, you can use the Tooltip
component provided by React Tippy:
import React from 'react';
import { Tooltip } from 'react-tippy';
const SimpleTooltip = () => {
return (
<Tooltip title="This is a simple tooltip">
<button>Hover me</button>
</Tooltip>
);
};
In this example, the tooltip will appear when the user hovers over the button. The title
prop specifies the content of the tooltip.
Custom Content Tooltip
React Tippy allows you to use custom content for your tooltips, including React components:
import React from 'react';
import { Tooltip } from 'react-tippy';
const CustomContentTooltip = () => {
const tooltipContent = (
<div>
<h3>Custom Tooltip</h3>
<p>This is a tooltip with custom content!</p>
</div>
);
return (
<Tooltip html={tooltipContent}>
<span>Hover for custom content</span>
</Tooltip>
);
};
Here, we use the html
prop to pass a React element as the tooltip content, allowing for more complex and styled tooltips.
Advanced Usage
React Tippy offers advanced features for more complex use cases and customizations.
Interactive Tooltips
You can create interactive tooltips that don’t close when hovered:
import React from 'react';
import { Tooltip } from 'react-tippy';
const InteractiveTooltip = () => {
return (
<Tooltip
interactive={true}
position="right"
trigger="click"
html={(
<div>
<h3>Interactive Tooltip</h3>
<button onClick={() => console.log('Button clicked!')}>
Click me!
</button>
</div>
)}
>
<button>Click for interactive tooltip</button>
</Tooltip>
);
};
This example creates a tooltip that opens on click, stays open when hovered, and contains an interactive button.
Controlled Tooltip
For more control over when the tooltip is shown or hidden, you can use the open
prop:
import React, { useState } from 'react';
import { Tooltip } from 'react-tippy';
const ControlledTooltip = () => {
const [isOpen, setIsOpen] = useState(false);
return (
<div>
<button onClick={() => setIsOpen(!isOpen)}>
Toggle Tooltip
</button>
<Tooltip
open={isOpen}
title="This is a controlled tooltip"
position="bottom"
>
<span>Controlled tooltip target</span>
</Tooltip>
</div>
);
};
This example demonstrates how to manually control the visibility of a tooltip using React state.
Using withTooltip HOC
React Tippy provides a higher-order component (HOC) for adding tooltip functionality to existing components:
import React from 'react';
import { withTooltip } from 'react-tippy';
const MyComponent = ({ children }) => (
<div>{children}</div>
);
const MyComponentWithTooltip = withTooltip(MyComponent, {
title: 'This is a tooltip added with HOC',
position: 'top',
});
const App = () => (
<MyComponentWithTooltip>
Hover over me for tooltip
</MyComponentWithTooltip>
);
This approach is useful when you want to add tooltip functionality to existing components without modifying their implementation.
Customization Options
React Tippy offers a wide range of customization options to tailor tooltips to your specific needs:
- Position: Control tooltip placement with options like
top
,bottom
,left
,right
, and their variations (e.g.,top-start
,bottom-end
). - Animations: Choose from various animation styles including
shift
,perspective
,fade
,scale
, andnone
. - Themes: Apply different visual themes such as
dark
,light
, ortransparent
. - Sizing: Adjust tooltip size with options like
small
,regular
, andbig
. - Timing: Customize show/hide delays and animation durations.
- Interactivity: Enable features like
followCursor
for mouse-following tooltips orsticky
for tooltips that stick to moving elements.
Here’s an example showcasing some of these customization options:
import React from 'react';
import { Tooltip } from 'react-tippy';
const CustomizedTooltip = () => {
return (
<Tooltip
title="Customized Tooltip"
position="right"
trigger="click"
animation="scale"
arrow={true}
theme="light"
duration={300}
distance={20}
>
<button>Click for custom tooltip</button>
</Tooltip>
);
};
This example creates a tooltip with a custom position, trigger, animation, theme, and other visual properties.
Conclusion
React Tippy provides a powerful and flexible solution for adding tooltips to your React applications. With its easy integration, extensive customization options, and support for advanced use cases, it’s an excellent choice for developers looking to enhance their UI with informative and interactive tooltips. Whether you’re building a simple website or a complex web application, React Tippy offers the tools you need to create engaging and user-friendly interfaces. By leveraging its features, you can significantly improve the user experience of your React projects, making information more accessible and your UI more intuitive.