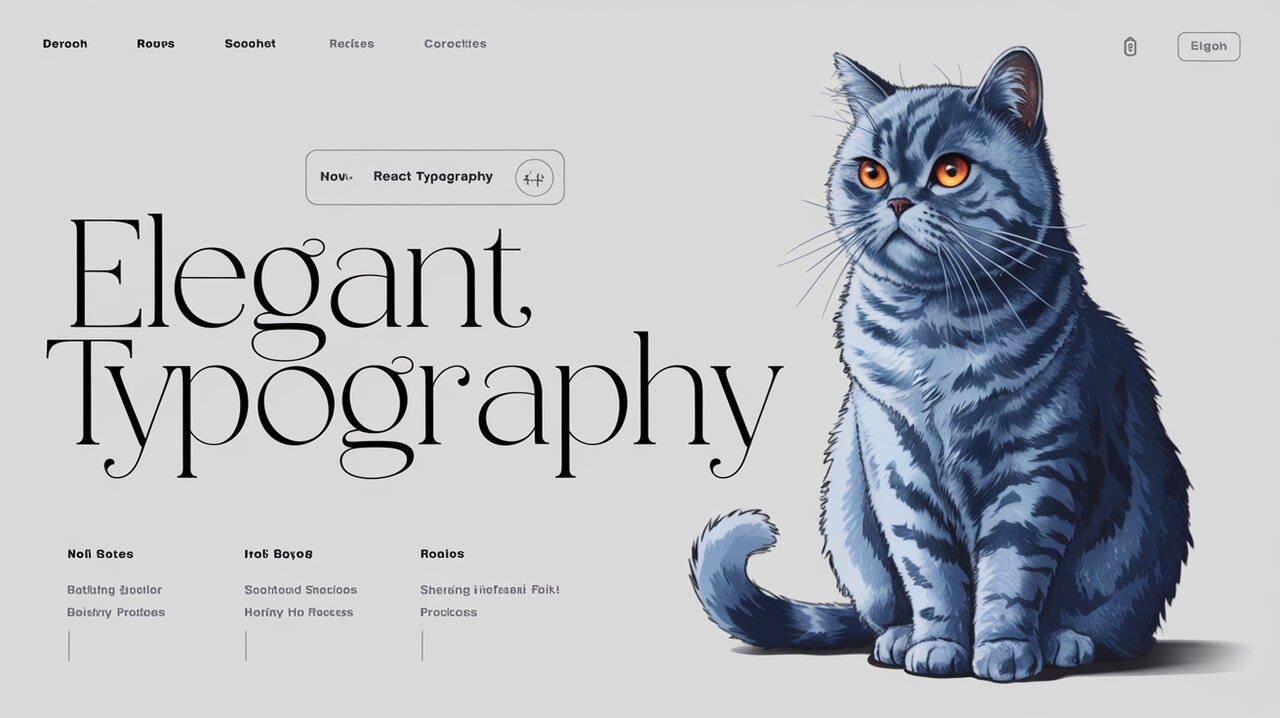
React Typography: Crafting Beautiful Web Designs with Ease
Typography plays a crucial role in web design, influencing readability and aesthetic appeal. The react-typography
library simplifies the process of creating beautiful, consistent typographic designs in React applications. Built on top of Typography.js, it provides a high-level API for managing fonts, sizes, line heights, and more, making it ideal for both beginners and seasoned developers.
Key Features of react-typography
- Effortless Styling: Automatically generates CSS for block and inline elements based on your configuration.
- Theme Support: Includes pre-built themes that can be customized or shared across projects.
- Plugins: Extend functionality with plugins like
typography-plugin-code
for specialized styles. - React Integration: Provides helper components (
TypographyStyle
,GoogleFont
) for seamless integration with React.
Getting Started
Installation
You can install the library using either npm or yarn:
npm install react-typography typography
or
yarn add react-typography typography
Setting Up
To start using react-typography
, you need to configure a typography instance. Here’s a simple example:
import Typography from 'typography';
const typography = new Typography({
baseFontSize: '18px',
baseLineHeight: 1.45,
headerFontFamily: ['Avenir Next', 'Helvetica Neue', 'Segoe UI', 'Helvetica', 'Arial', 'sans-serif'],
bodyFontFamily: ['Georgia', 'serif'],
});
export default typography;
Basic Usage
Injecting Styles
The easiest way to apply styles is by injecting them directly into your application:
import typography from './typography';
typography.injectStyles();
This method works well for client-side rendering but might not be ideal for server-side rendering.
Using Helper Components
For server-rendered React apps, use the helper components:
import { TypographyStyle, GoogleFont } from 'react-typography';
import typography from './typography';
function App() {
return (
<html>
<head>
<TypographyStyle typography={typography} />
<GoogleFont typography={typography} />
</head>
<body>
<h1>Welcome to React Typography</h1>
<p>Crafting beautiful text has never been easier!</p>
</body>
</html>
);
}
Advanced Customization
Using Themes
Themes are pre-configured settings that you can import and customize. For example:
import Typography from 'typography';
import funstonTheme from 'typography-theme-funston';
const typography = new Typography(funstonTheme);
export default typography;
You can also modify themes to suit your needs:
funstonTheme.baseFontSize = '20px'; // Adjust font size
const typography = new Typography(funstonTheme);
Overriding Styles
For more control, use the overrideThemeStyles
method to tweak specific elements:
funstonTheme.overrideThemeStyles = ({ rhythm }) => ({
'h1,h2': {
marginBottom: rhythm(1),
color: '#333',
},
});
const typography = new Typography(funstonTheme);
Adding Plugins
Extend functionality by adding plugins like typography-plugin-code
:
import CodePlugin from 'typography-plugin-code';
funstonTheme.plugins = [new CodePlugin()];
const typography = new Typography(funstonTheme);
Conclusion
The react-typography library empowers developers to create stunning typographic designs effortlessly. With its robust API, theme support, and seamless React integration, it’s a must-have tool for any web project. Whether you’re building a blog or a corporate website, react-typography
ensures your text looks as good as your code.
For more insights into styling libraries in React, check out articles like “React Headroom: Elevate Your Header Game” or “React Helmet Async SEO Wizardry”.