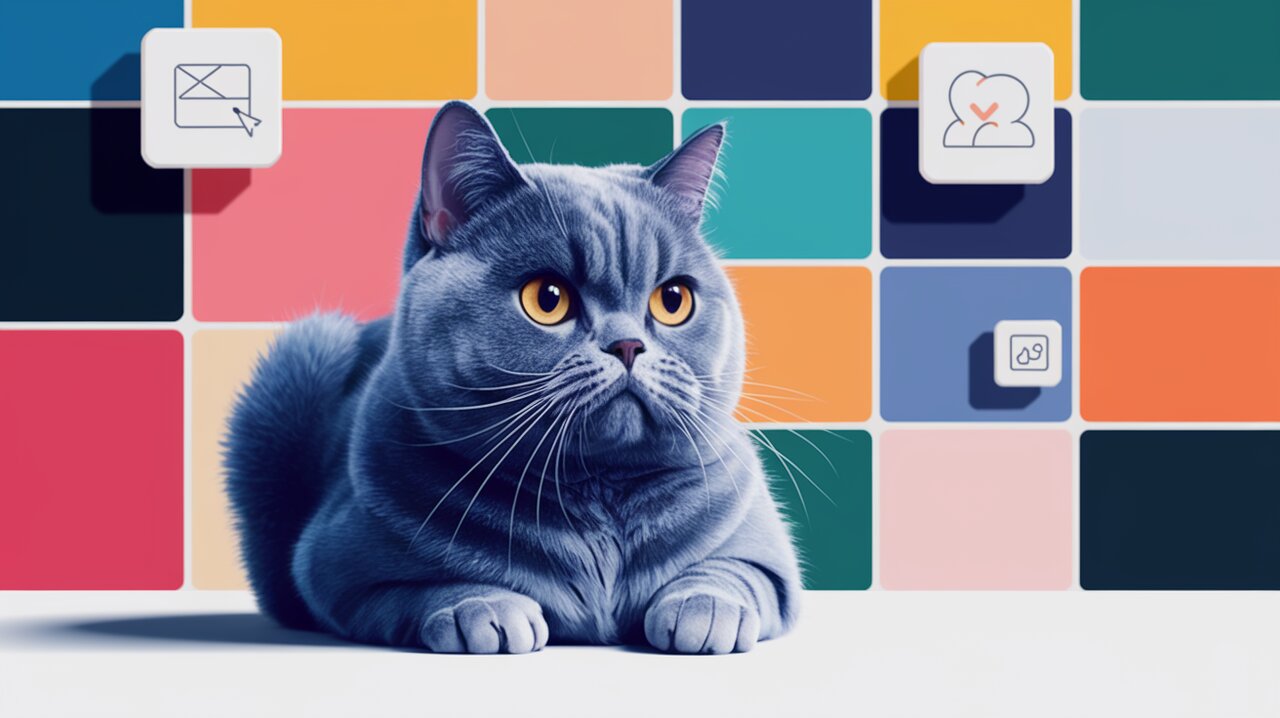
Simplify Image Management with React Upload Gallery
Revolutionize Your React Image Handling
In the world of web development, managing image galleries and file uploads can be a complex task. Enter React Upload Gallery, a powerful library that simplifies this process for React developers. This versatile tool allows you to create customizable image galleries, handle file uploads with ease, and provide a seamless user experience.
Key Features
React Upload Gallery comes packed with an array of features that make it stand out:
- Drag-and-drop functionality for intuitive image uploading
- Customizable UI components for gallery display
- Image sorting capabilities
- Flexible rule-setting for file uploads
- Support for custom HTTP requests
- Comprehensive event handling for various upload stages
Getting Started
To begin using React Upload Gallery in your project, you’ll need to install it first. Open your terminal and run one of the following commands:
npm install react-upload-gallery
or if you prefer yarn:
yarn add react-upload-gallery
Once installed, you can import the library and its styles into your React component:
import RUG from 'react-upload-gallery'
import 'react-upload-gallery/dist/style.css' // or use the SCSS version
Basic Usage
Let’s dive into a simple example to showcase the basic functionality of React Upload Gallery:
import React from 'react'
import RUG from 'react-upload-gallery'
import 'react-upload-gallery/dist/style.css'
const ImageGallery = () => {
return (
<RUG
action="/api/upload"
source={(response) => response.source}
/>
)
}
export default ImageGallery
In this example, we’ve created a basic image gallery component. The action
prop specifies the upload endpoint, while the source
prop defines how to extract the image source from the server response[1].
Customizing the Gallery
React Upload Gallery offers extensive customization options. Let’s explore some advanced usage scenarios:
Setting Upload Rules
You can define rules for uploaded images to ensure they meet specific criteria:
<RUG
rules={{
limit: 10,
size: 20,
width: { min: 1280, max: 1920 },
height: { min: 720, max: 1080 }
}}
accept={['jpg', 'jpeg']}
onWarning={(type, rules) => {
switch(type) {
case 'accept':
console.log(`Only ${rules.accept.join(', ')} allowed`)
break
case 'limit':
console.log(`Maximum ${rules.limit} images allowed`)
break
// ... handle other cases
}
}}
/>
This configuration sets limits on the number of images, file size, dimensions, and accepted file types[1].
Custom Request Handling
For more control over the upload process, you can implement a custom request handler:
<RUG
customRequest={({ uid, file, action, onProgress, onSuccess, onError }) => {
const formData = new FormData()
formData.append('file', file)
fetch(action, {
method: 'POST',
body: formData
})
.then(response => response.json())
.then(data => {
onSuccess(uid, data)
})
.catch(error => {
onError(uid, { status: 'error', response: error })
})
return {
abort: () => {
// Cancel the request if needed
}
}
}}
source={response => response.url}
/>
This example demonstrates how to implement a custom upload request using the Fetch API[1].
Advanced Components
React Upload Gallery provides several advanced components for further customization:
DragArea Component
The DragArea
component allows you to create a custom draggable area for images:
import RUG, { DragArea } from 'react-upload-gallery'
<RUG>
<DragArea>
{(image) => (
<div className="custom-image-container">
<img src={image.source} alt={image.name} />
<button onClick={image.remove}>Remove</button>
</div>
)}
</DragArea>
</RUG>
This setup creates a draggable area with custom image containers and removal buttons[1].
DropArea Component
The DropArea
component enables you to create a custom drop zone for file uploads:
<RUG
header={({ openDialogue }) => (
<DropArea>
{(isDrag) => (
<div style={{ background: isDrag ? '#f0f0f0' : '#fff' }}>
<button onClick={openDialogue}>Select Files</button>
<p>or drag and drop files here</p>
</div>
)}
</DropArea>
)}
/>
This example creates a header with a drop area that changes appearance when files are dragged over it[1].
Handling Gallery Events
React Upload Gallery provides various event handlers to manage different aspects of the gallery:
<RUG
onChange={(images) => {
console.log('Gallery updated:', images)
}}
onClick={(image) => {
console.log('Image clicked:', image)
}}
onConfirmDelete={(currentImage, images) => {
return window.confirm('Are you sure you want to delete this image?')
}}
onSortEnd={(images, { oldIndex, newIndex }) => {
console.log('Image order changed:', images)
}}
/>
These event handlers allow you to respond to changes in the gallery, handle image clicks, confirm deletions, and manage image reordering[1].
Conclusion
React Upload Gallery offers a comprehensive solution for managing image galleries and file uploads in React applications. Its flexibility, extensive customization options, and intuitive API make it an excellent choice for developers looking to implement robust image handling features.
By leveraging the power of React Upload Gallery, you can create visually appealing and highly functional image galleries that enhance user experience and streamline file management in your React projects.