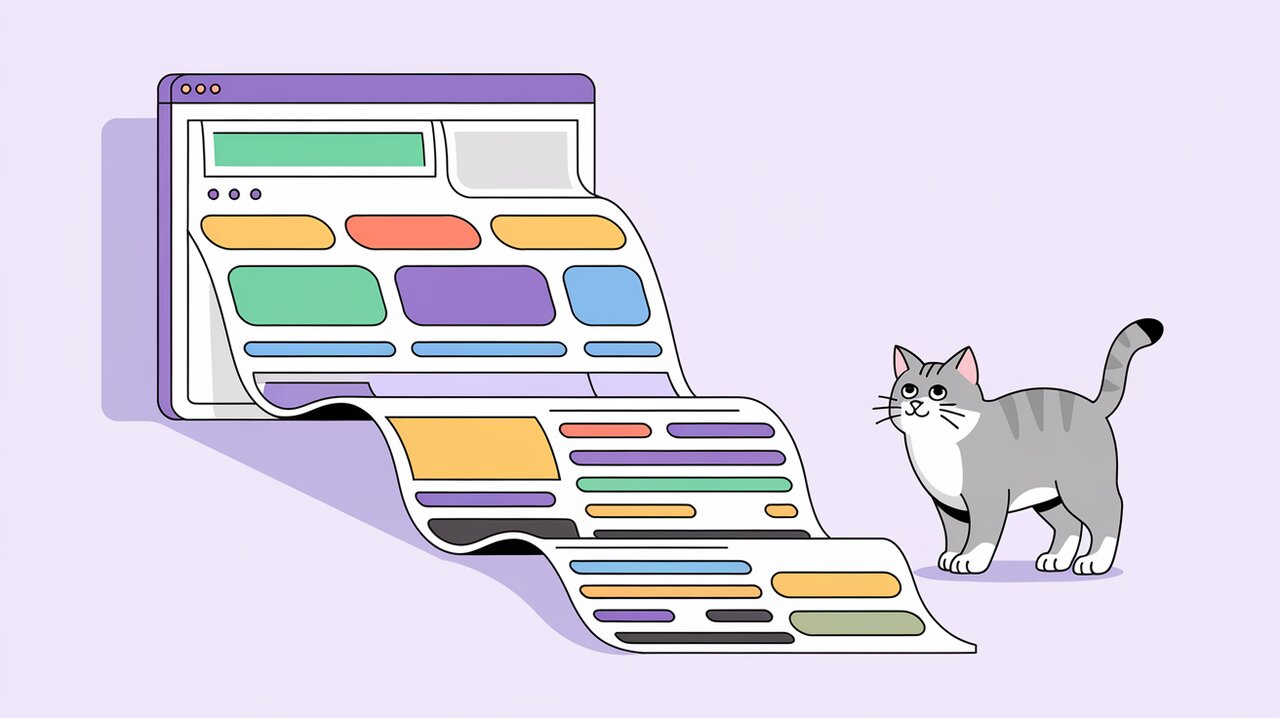
Mastering Infinite Scroll with React Waypoint
Revolutionizing User Experience with Infinite Scroll
In the world of web development, creating engaging and seamless user experiences is paramount. One technique that has gained significant popularity is infinite scrolling, which allows users to continuously load content as they scroll down a page. React Waypoint, a powerful library for React applications, makes implementing this feature a breeze.
What is React Waypoint?
React Waypoint is a component that allows you to execute a function whenever you scroll to an element. It works in all containers that can scroll, including the window itself. This versatility makes it an ideal choice for implementing features like lazy loading content, infinite scroll, scrollspies, or docking elements to the viewport on scroll.
Getting Started with React Waypoint
Installation
To begin using React Waypoint in your project, you’ll need to install it first. You can do this using npm or yarn:
npm install react-waypoint --save
or
yarn add react-waypoint
Basic Usage
Once installed, you can import and use the Waypoint component in your React application:
import { Waypoint } from 'react-waypoint';
function MyComponent() {
const handleWaypointEnter = () => {
console.log('Waypoint entered');
};
return (
<div>
<Waypoint onEnter={handleWaypointEnter} />
{/* Your content here */}
</div>
);
}
In this basic example, the onEnter
callback will be triggered when the Waypoint enters the viewport.
Implementing Infinite Scroll
Now, let’s dive into how we can use React Waypoint to create an infinite scroll feature.
Setting Up the State
First, we’ll need to set up some state to manage our data and pagination:
import React, { useState, useEffect } from 'react';
import { Waypoint } from 'react-waypoint';
function InfiniteScrollComponent() {
const [items, setItems] = useState([]);
const [page, setPage] = useState(1);
const [hasNextPage, setHasNextPage] = useState(true);
// ... rest of the component
}
Fetching Data
Next, we’ll create a function to fetch data from an API:
const fetchData = async () => {
if (!hasNextPage) return;
try {
const response = await fetch(`https://api.example.com/items?page=${page}`);
const newItems = await response.json();
setItems(prevItems => [...prevItems, ...newItems]);
setPage(prevPage => prevPage + 1);
setHasNextPage(newItems.length > 0);
} catch (error) {
console.error('Error fetching data:', error);
}
};
Rendering Items and Waypoint
Now, let’s render our items and add the Waypoint at the bottom:
return (
<div>
{items.map(item => (
<div key={item.id}>{item.name}</div>
))}
{hasNextPage && (
<Waypoint onEnter={fetchData} />
)}
</div>
);
Advanced Usage
React Waypoint offers several advanced features that can enhance your infinite scroll implementation:
Customizing Offsets
You can use the topOffset
and bottomOffset
props to adjust when the Waypoint triggers:
<Waypoint
onEnter={fetchData}
topOffset="100px"
bottomOffset="100px"
/>
This will trigger the Waypoint when it’s 100 pixels from entering or leaving the viewport.
Horizontal Scrolling
React Waypoint also supports horizontal scrolling. Simply add the horizontal
prop:
<Waypoint
onEnter={fetchData}
horizontal={true}
/>
Debugging
For troubleshooting, you can use the debug
prop to log helpful information to the console:
<Waypoint
onEnter={fetchData}
debug={true}
/>
Performance Considerations
While infinite scroll can greatly enhance user experience, it’s important to consider performance, especially when dealing with large datasets. Here are some tips:
-
Virtualization: For very long lists, consider using a virtualization library like
react-window
to render only visible items. -
Throttling: Implement throttling on your scroll event handlers to prevent excessive function calls.
-
Cleanup: Remember to clean up event listeners and cancel any ongoing fetch requests when the component unmounts.
Conclusion
React Waypoint provides a powerful and flexible solution for implementing infinite scroll in React applications. By leveraging its capabilities, developers can create smooth, efficient, and engaging user experiences that keep users immersed in content.
As you implement infinite scroll in your projects, remember to balance the user experience with performance considerations. With React Waypoint, you have a robust tool at your disposal to create seamless scrolling experiences that will delight your users and keep them engaged with your content.