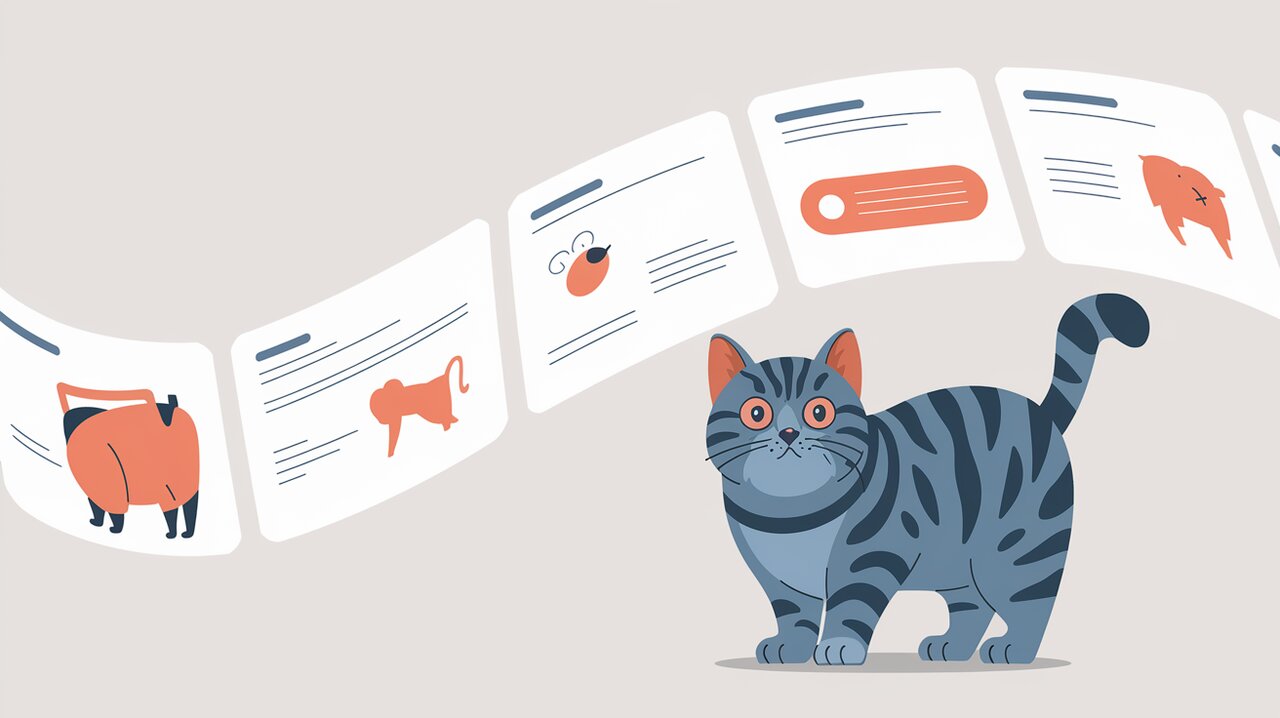
Animating with Ease in React
The react-web-animation library is a powerful tool designed for React developers looking to implement complex animations using the Web Animations API. This library offers a declarative approach, making it easier to control animations directly within your React components without relying on third-party frameworks or CSS.
Key Features of React-Web-Animation
- Declarative Animation Components: Use
<Animated.div>
and other elements to easily apply animations. - Control Over Animations: Play, pause, stop, or reverse animations with simple controls.
- Parallel and Sequential Animations: Manage multiple animations simultaneously or in sequence using
<AnimationGroup>
and<AnimationSequence>
.
Getting Started with Installation
To start using react-web-animation
, ensure you have its peer dependencies installed:
npm install react react-dom prop-types
Then, add react-web-animation
to your project:
npm install react-web-animation
Include the Web Animations API polyfill in your application for broader browser support:
<script src="https://cdnjs.cloudflare.com/ajax/libs/web-animations/2.2.1/web-animations-next.min.js"></script>
Bringing Animations to Life
Basic Animation Setup
Creating simple animations is straightforward with react-web-animation
. Here’s how you can animate an element:
import React from 'react';
import { Animated } from 'react-web-animation';
const BasicAnimation: React.FC = () => {
const keyframes = [
{ transform: 'scale(1)', opacity: 1, offset: 0 },
{ transform: 'scale(0.5)', opacity: 0.5, offset: 0.3 },
{ transform: 'scale(0.667)', opacity: 0.667, offset: 0.7875 },
{ transform: 'scale(0.6)', opacity: 0.6, offset: 1 }
];
const timing = {
duration: 2500,
easing: 'ease-in-out',
delay: 0,
iterations: Infinity,
direction: 'alternate',
fill: 'forwards'
};
return (
<Animated.div keyframes={keyframes} timing={timing}>
Web Animations API Rocks!
</Animated.div>
);
};
export default BasicAnimation;
In this example, we define keyframes for scaling and fading effects and apply them to an <Animated.div>
component.
Advanced Animation Techniques
For more complex scenarios, such as coordinating multiple animations:
import React from 'react';
import { AnimationGroup } from 'react-web-animation';
const AdvancedAnimations: React.FC = () => {
const animations = [
{ keyframes: [{ transform: 'translateX(0px)' }, { transform: 'translateX(100px)' }], timing: { duration: 1000 } },
{ keyframes: [{ transform: 'rotate(0deg)' }, { transform: 'rotate(360deg)' }], timing: { duration: 1000 } }
];
return (
<AnimationGroup animations={animations}>
<div>First Animation</div>
<div>Second Animation</div>
</AnimationGroup>
);
};
export default AdvancedAnimations;
This setup uses <AnimationGroup>
to run multiple animations in parallel.
Wrapping Up
The react-web-animation library provides an intuitive way to integrate sophisticated animations into your React applications by leveraging the native Web Animations API. Whether you’re animating single elements or complex sequences, this library simplifies the process while offering robust control over animation behaviors.
For more insights into animation libraries in React, explore similar articles like Animating with Framer Motion and Animating Text Transitions.