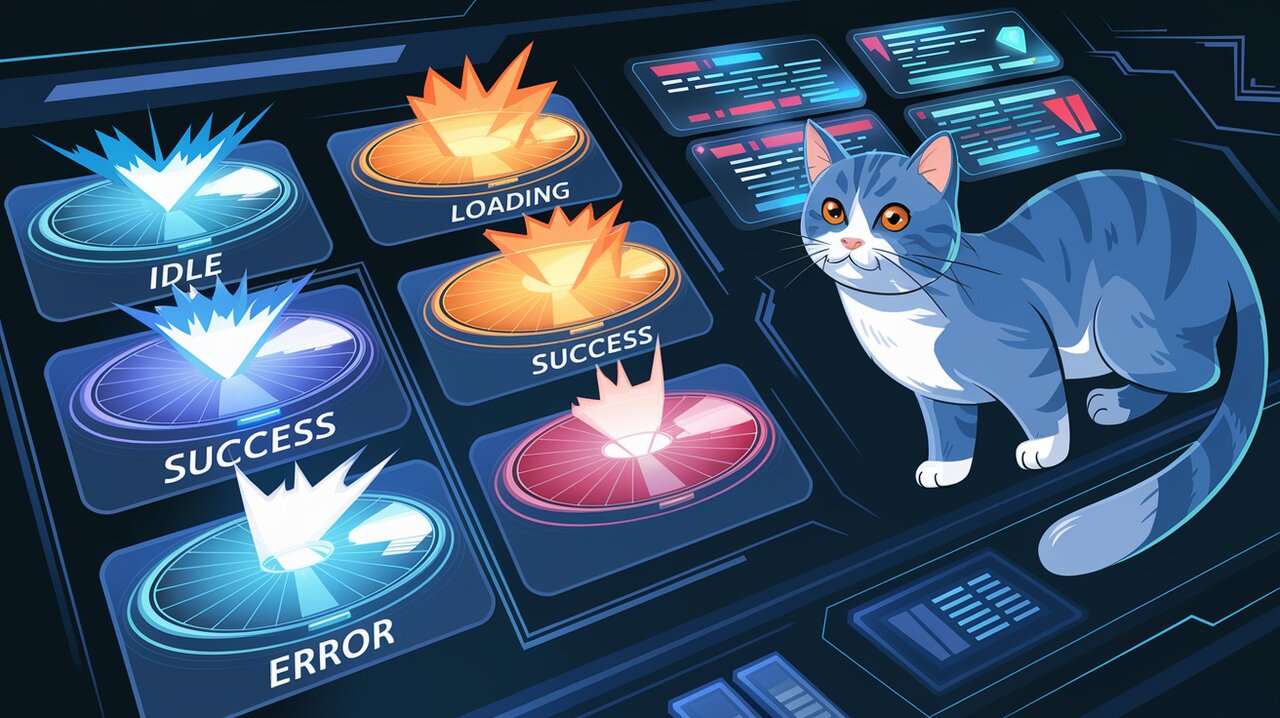
Supercharge Your React UI with Reactive Button: The 3D Animated Button Revolution
In the ever-evolving world of web development, creating engaging user interfaces is paramount. Enter Reactive Button, a game-changing React component that transforms mundane buttons into captivating, 3D animated elements. This library offers developers a powerful tool to enhance user experience without the need for complex UI frameworks.
Unveiling the Magic of Reactive Button
Reactive Button is not just another button component. It’s a feature-rich, highly customizable solution that brings life to your React applications. Here’s what makes it stand out:
- 3D animations that respond to user interactions
- Built-in progress indicator for visual feedback
- Support for custom icons and text
- Lightweight and zero-dependency design
- TypeScript support for enhanced development experience
- Easily customizable appearance and behavior
Getting Started with Reactive Button
Installation
To begin your journey with Reactive Button, you’ll need to install it in your React project. You can do this using npm or yarn:
npm install reactive-button
or
yarn add reactive-button
Basic Implementation
Let’s dive into a simple example to showcase the power of Reactive Button:
import React, { useState } from 'react';
import ReactiveButton from 'reactive-button';
const SubmitButton: React.FC = () => {
const [state, setState] = useState('idle');
const onClickHandler = () => {
setState('loading');
// Simulating an API call
setTimeout(() => {
setState('success');
}, 2000);
};
return (
<ReactiveButton
buttonState={state}
onClick={onClickHandler}
color="primary"
idleText="Submit"
loadingText="Processing..."
successText="Done!"
errorText="Error!"
/>
);
};
export default SubmitButton;
In this example, we create a submit button that changes its state and text based on user interaction. The button starts in an ‘idle’ state, transitions to ‘loading’ when clicked, and finally shows a ‘success’ message.
Customizing Your Reactive Button
Color Palette
Reactive Button comes with a variety of pre-defined color options to match your application’s design:
<ReactiveButton color="primary" />
<ReactiveButton color="secondary" />
<ReactiveButton color="teal" />
<ReactiveButton color="green" />
<ReactiveButton color="red" />
<ReactiveButton color="violet" />
<ReactiveButton color="blue" />
<ReactiveButton color="yellow" />
<ReactiveButton color="dark" />
<ReactiveButton color="light" />
Size Variations
Adapt your buttons to different contexts with four size options:
<ReactiveButton size="tiny" />
<ReactiveButton size="small" />
<ReactiveButton size="medium" />
<ReactiveButton size="large" />
Stylish Enhancements
Add some flair to your buttons with these style props:
<ReactiveButton outline />
<ReactiveButton rounded />
<ReactiveButton shadow />
Advanced Usage and Techniques
Integrating with Existing State Management
If your application already uses boolean states for loading indicators, you can easily integrate Reactive Button:
const [isLoading, setIsLoading] = useState(false);
return (
<ReactiveButton
buttonState={isLoading ? 'loading' : 'idle'}
onClick={() => setIsLoading(true)}
idleText="Start Process"
loadingText="Processing..."
/>
);
Incorporating Icons
Enhance your buttons with custom icons for a more intuitive UI:
import { FaPaperPlane } from 'react-icons/fa';
<ReactiveButton
idleText={
<span>
<FaPaperPlane /> Send
</span>
}
/>
Form Submission
For form submissions, set the type
prop to ‘submit’:
<form onSubmit={handleSubmit}>
<input type="text" name="username" />
<ReactiveButton type="submit" idleText="Submit Form" />
</form>
Conclusion
Reactive Button is a powerful tool in the React developer’s arsenal, offering a blend of aesthetics and functionality. By leveraging its 3D animations, state management, and customization options, you can create buttons that not only look great but also provide meaningful feedback to users.
As you continue to explore the capabilities of Reactive Button, you’ll find it to be an invaluable asset in creating more engaging and interactive React applications. Whether you’re building a simple contact form or a complex dashboard, Reactive Button has the flexibility to meet your needs and elevate your user interface.
Remember, great UIs are about more than just looks – they’re about creating intuitive, responsive experiences for your users. With Reactive Button, you’re well on your way to achieving just that.