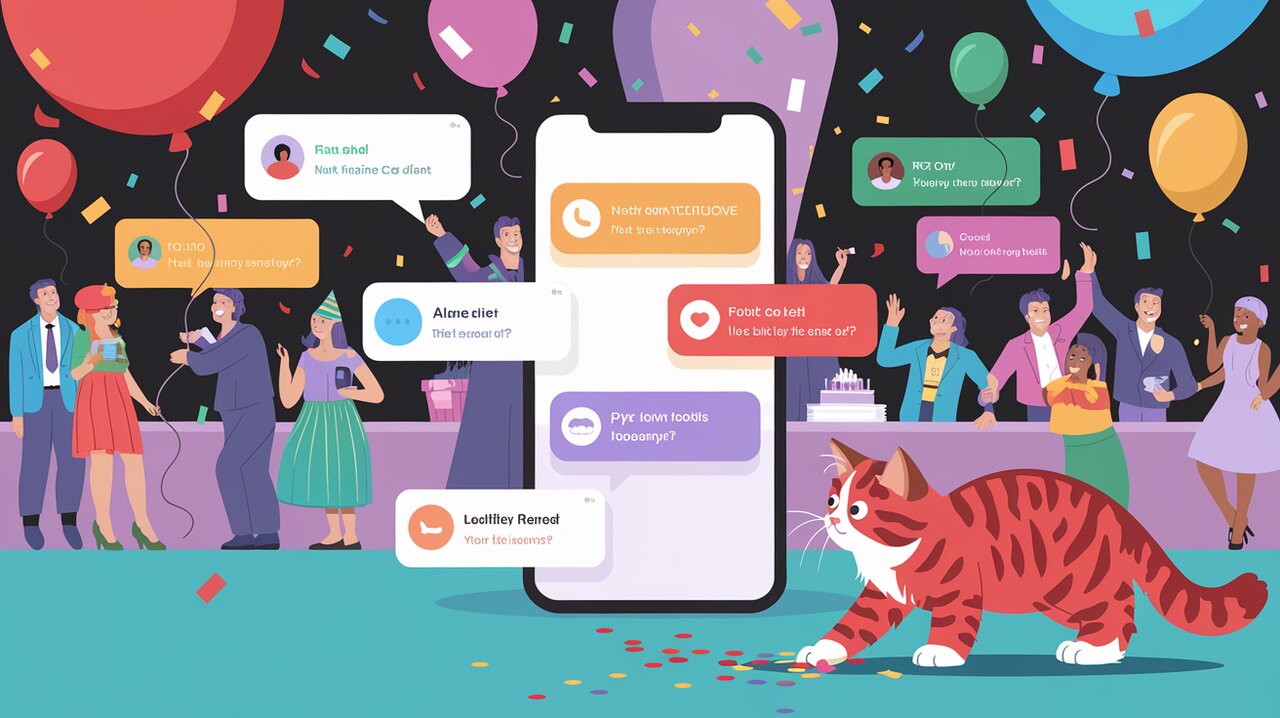
Reapop is a simple yet powerful notification system for React applications that allows developers to create and manage notifications with ease. Whether you’re building a complex web application or a simple React project, Reapop provides a flexible and customizable solution for keeping your users informed and engaged.
Notification Extravaganza: Key Features
Reapop comes packed with a variety of features that make it stand out:
- Multiple notification positions
- Customizable themes
- Support for images and buttons in notifications
- Automatic dismissal with customizable timers
- Responsive design for small screens
- Easy integration with Redux (optional)
Setting Up the Party: Installation
To get started with Reapop, you’ll need to install it in your React project. You can do this using npm or yarn:
npm install reapop --save
or
yarn add reapop
Basic Usage: Sending Your First Notification
Let’s dive into how you can use Reapop to create notifications in your React application. We’ll start with the basic setup and then move on to more advanced features.
Configuring Reapop
First, you’ll want to set up some default properties for your notifications. This is done using the setUpNotifications
function:
import { setUpNotifications } from 'reapop'
setUpNotifications({
defaultProps: {
position: 'top-right',
dismissible: true
}
})
This configuration sets all notifications to appear in the top-right corner of the screen and makes them dismissible by default.
Adding the Notification System
Next, you need to add the NotificationsSystem
component to your app. This component should be placed at the root of your application to avoid positioning conflicts:
import React from 'react'
import NotificationsSystem, { atalhoTheme, useNotifications } from 'reapop'
const App = () => {
const { notifications, dismissNotification } = useNotifications()
return (
<div>
<NotificationsSystem
notifications={notifications}
dismissNotification={(id) => dismissNotification(id)}
theme={atalhoTheme}
/>
{/* Your other components */}
</div>
)
}
In this example, we’re using the atalhoTheme
, which is one of the built-in themes provided by Reapop. You can also create your own custom theme if desired.
Creating Notifications
Now that we have our system set up, let’s create a notification:
import React, { useEffect } from 'react'
import { useNotifications } from 'reapop'
const WelcomeComponent = () => {
const { notify } = useNotifications()
useEffect(() => {
notify('Welcome to our app!', 'success')
}, [])
return <div>Welcome page content</div>
}
This will display a success notification with the message “Welcome to our app!” when the component mounts.
Advanced Notification Techniques
Reapop offers more advanced features for creating rich, interactive notifications. Let’s explore some of these capabilities.
Custom Notification Styles
You can customize the appearance of your notifications by passing additional properties:
notify({
title: 'File Upload',
message: 'Your file is being uploaded...',
status: 'loading',
dismissible: false,
dismissAfter: 0,
image: 'path/to/upload-icon.png'
})
This creates a non-dismissible notification with a title, custom message, loading status, and an image.
Adding Buttons to Notifications
Reapop allows you to add interactive buttons to your notifications:
notify({
title: 'Friend Request',
message: 'John Doe wants to be your friend',
status: 'info',
buttons: [
{
name: 'Accept',
primary: true,
onClick: () => handleAcceptFriend()
},
{
name: 'Decline',
onClick: () => handleDeclineFriend()
}
]
})
This notification includes two buttons that trigger different actions when clicked.
Updating Existing Notifications
You can update an existing notification by using its ID:
const { payload: notification } = notify('Uploading file...', 'loading')
// Later, update the notification
setTimeout(() => {
notification.status = 'success'
notification.message = 'File uploaded successfully!'
notify(notification)
}, 3000)
This example shows how to update a notification’s status and message after a certain operation completes.
Theming Your Notification Fiesta
Reapop comes with three built-in themes: atalho, wybo, and bootstrap. You can easily switch between these themes or create your own custom theme.
Using a Built-in Theme
To use a built-in theme, simply import it and pass it to the NotificationsSystem
component:
import { wyboTheme } from 'reapop'
<NotificationsSystem
// ... other props
theme={wyboTheme}
/>
Creating a Custom Theme
For more control over the appearance of your notifications, you can create a custom theme:
import { Theme, baseTheme } from 'reapop'
const customTheme: Theme = {
...baseTheme,
notification: (notification) => ({
backgroundColor: notification.status === 'success' ? '#4CAF50' : '#F44336',
color: '#FFFFFF',
// Add more custom styles here
}),
// Customize other elements like buttons, icons, etc.
}
Then use your custom theme in the NotificationsSystem
component:
<NotificationsSystem
// ... other props
theme={customTheme}
/>
Wrapping Up the Notification Party
Reapop provides a powerful and flexible system for adding notifications to your React applications. With its easy setup, customizable themes, and advanced features like buttons and updates, you can create a rich notification experience that enhances user interaction and keeps them informed about important events in your app.
Whether you’re using it with Redux or as a standalone solution with React hooks, Reapop’s simplicity and extensibility make it an excellent choice for managing notifications in your projects. So go ahead, throw a notification fiesta in your React app, and watch your user engagement soar!
For more advanced usage and detailed API documentation, you can refer to the official Reapop documentation. Happy notifying!