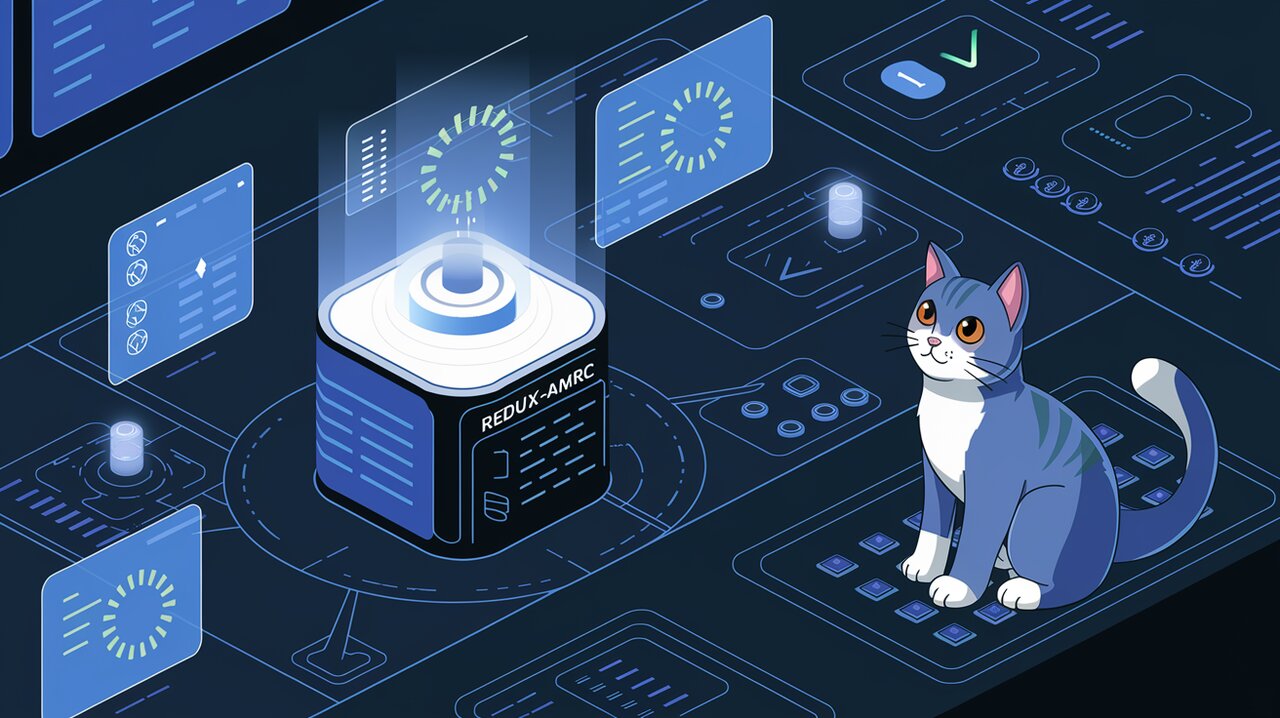
Taming Async Actions with Redux-AMRC: Your Middleware Superhero
Redux has revolutionized state management in React applications, but handling asynchronous actions can still be a challenge. Enter Redux Async Middleware and Reducer Creator (redux-amrc), a powerful library that simplifies the process of dispatching async actions and managing their states. By reducing boilerplate and automating common async patterns, redux-amrc allows developers to focus on what matters most: building great applications.
Simplifying Async Workflows
At its core, redux-amrc provides two main features: a middleware for handling async actions and a reducer creator for managing async states. These tools work together to streamline your Redux workflow, making it easier to handle complex asynchronous operations without cluttering your codebase.
Key Features of redux-amrc
- Automatic Action Creation: Say goodbye to manually writing async action objects. Redux-amrc generates them for you.
- Built-in Async State Management: Get access to multiple async states like loading, loaded, and error without extra code.
- Reducer Automation: No need to write reducers for handling async action objects manually.
- Performance Optimization: Includes options to prevent unnecessary data loading, improving application performance.
- Customizable: Allows integration with custom reducers for fine-grained control over state updates.
Getting Started with redux-amrc
Let’s dive into how you can integrate redux-amrc into your React Redux project.
Installation
First, install the package using npm or yarn:
npm install redux-amrc --save
# or
yarn add redux-amrc
Basic Configuration
To set up redux-amrc, you need to configure your Redux store and reducers.
Configuring the Store
Add the asyncMiddleware
to your Redux middleware list:
import { createStore, applyMiddleware } from 'redux';
import { asyncMiddleware } from 'redux-amrc';
import rootReducer from './reducers';
const store = createStore(
rootReducer,
applyMiddleware(asyncMiddleware)
);
Setting Up the Reducer
Use the reducerCreator
to handle async states in your root reducer:
import { combineReducers } from 'redux';
import { reducerCreator } from 'redux-amrc';
const rootReducer = combineReducers({
async: reducerCreator()
});
export default rootReducer;
Creating Async Actions
Now, let’s create some async actions using redux-amrc:
import { ASYNC } from 'redux-amrc';
function fetchUserData(userId: string) {
return {
[ASYNC]: {
key: 'userData',
promise: () => fetch(`/api/users/${userId}`).then(res => res.json())
}
};
}
This action creator will automatically generate LOAD
, LOAD_SUCCESS
, and LOAD_FAIL
actions for you.
Advanced Usage: Optimizing Performance
Redux-amrc offers advanced features to optimize your application’s performance. Let’s explore some of these capabilities.
Preventing Redundant Data Fetching
Use the once
option to avoid reloading data that’s already in the state:
function loadUserProfile(userId: string) {
return {
[ASYNC]: {
key: `userProfile_${userId}`,
promise: () => fetch(`/api/users/${userId}/profile`).then(res => res.json()),
once: true
}
};
}
This ensures that the user profile is only fetched once, even if the action is dispatched multiple times.
Custom Reducers for Fine-Grained Control
You can integrate custom reducers with redux-amrc for more control over state updates:
import { reducerCreator } from 'redux-amrc';
const TOGGLE_FAVORITE = 'TOGGLE_FAVORITE';
function userProfileReducer(state = {}, action) {
switch (action.type) {
case TOGGLE_FAVORITE:
return { ...state, isFavorite: !state.isFavorite };
default:
return state;
}
}
const asyncReducer = reducerCreator({
userProfile: userProfileReducer
});
Now you can dispatch custom actions that will be handled by your reducer while still benefiting from redux-amrc’s async handling.
Leveraging Async States in Components
Redux-amrc automatically generates various async states that you can use in your components:
import React from 'react';
import { useSelector } from 'react-redux';
function UserProfile({ userId }) {
const userProfile = useSelector(state => state.async[`userProfile_${userId}`]);
const { loading, loaded, error } = useSelector(state => state.async.loadState[`userProfile_${userId}`]);
if (loading) return <div>Loading...</div>;
if (error) return <div>Error: {error}</div>;
if (!loaded) return null;
return (
<div>
<h1>{userProfile.name}</h1>
<p>{userProfile.bio}</p>
</div>
);
}
This component seamlessly integrates with the async states managed by redux-amrc, providing a smooth user experience.
Conclusion: Streamlining Redux Async Operations
Redux-amrc offers a powerful solution for managing async actions in Redux applications. By automating action creation, reducing boilerplate, and providing built-in async state management, it allows developers to focus on building features rather than wrestling with async logic. Whether you’re working on a small project or a large-scale application, redux-amrc can significantly simplify your Redux workflow and improve your development experience.
As you continue to explore redux-amrc, you’ll discover even more ways to optimize your async operations and create more efficient, maintainable Redux applications. Happy coding!