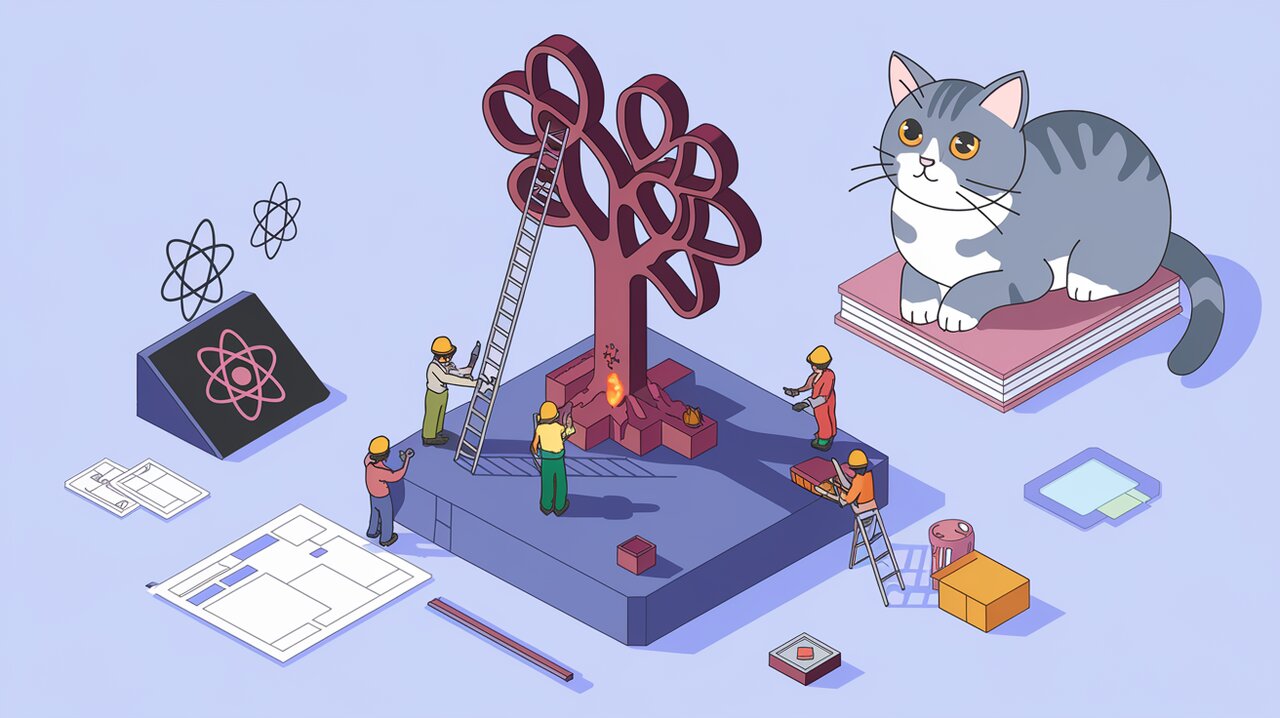
Redux has become an indispensable tool for managing state in complex JavaScript applications, particularly when combined with React. However, setting up a Redux project from scratch can be time-consuming and prone to configuration errors. Enter generator-redux-app, a powerful Yeoman generator that streamlines the process of scaffolding Redux applications, allowing developers to focus on building features rather than wrestling with boilerplate code.
Supercharging Your Redux Setup
generator-redux-app offers a suite of features that make it an invaluable tool for Redux developers:
- Instant project scaffolding based on best practices
- Integration with React Router for seamless navigation
- Jest configuration for robust testing out of the box
- Pre-configured Redux boilerplate extending the official counter example
- Optimized development workflow with hot reloading
Getting Started with generator-redux-app
Before we dive into using generator-redux-app, let’s ensure we have the necessary tools installed.
Installation
First, you’ll need to install Yeoman and generator-redux-app globally:
npm install -g yo generator-redux-app
For yarn enthusiasts:
yarn global add yo generator-redux-app
Scaffolding Your First Redux App
With the generator installed, creating a new Redux application is as simple as running:
mkdir my-redux-app && cd my-redux-app
yo redux-app
This command will scaffold a new Redux application in the current directory, complete with all the necessary boilerplate and configuration files.
Basic Usage: Exploring the Generated Structure
Let’s take a look at the core components of your newly generated Redux application.
Redux Store Setup
The generator creates a pre-configured Redux store in src/store/configureStore.ts
:
import { createStore, applyMiddleware, compose } from 'redux';
import thunk from 'redux-thunk';
import rootReducer from '../reducers';
const configureStore = (initialState = {}) => {
const middlewares = [thunk];
const enhancers = [];
const store = createStore(
rootReducer,
initialState,
compose(applyMiddleware(...middlewares), ...enhancers)
);
return store;
};
export default configureStore;
This setup includes Redux Thunk middleware for handling asynchronous actions and is ready for additional middleware and enhancers.
Root Reducer
The root reducer is generated in src/reducers/index.ts
:
import { combineReducers } from 'redux';
import counter from './counterReducer';
const rootReducer = combineReducers({
counter,
});
export default rootReducer;
Here, you can easily add more reducers as your application grows.
Advanced Usage: Customizing Your Redux Setup
generator-redux-app provides a solid foundation, but real-world applications often require more complex setups. Let’s explore some advanced customizations.
Adding Custom Middleware
To add custom middleware, you can modify the configureStore.ts
file:
import { createStore, applyMiddleware, compose } from 'redux';
import thunk from 'redux-thunk';
import logger from 'redux-logger';
import rootReducer from '../reducers';
const configureStore = (initialState = {}) => {
const middlewares = [thunk, logger];
const enhancers = [];
// Add Redux DevTools Extension support
if (process.env.NODE_ENV === 'development' && window.__REDUX_DEVTOOLS_EXTENSION__) {
enhancers.push(window.__REDUX_DEVTOOLS_EXTENSION__());
}
const store = createStore(
rootReducer,
initialState,
compose(applyMiddleware(...middlewares), ...enhancers)
);
return store;
};
export default configureStore;
This example adds Redux Logger for development and includes support for the Redux DevTools Extension.
Implementing Async Actions
generator-redux-app comes with Redux Thunk, making it easy to implement async actions:
import { Dispatch } from 'redux';
import { fetchUserData } from '../api/userService';
export const FETCH_USER_REQUEST = 'FETCH_USER_REQUEST';
export const FETCH_USER_SUCCESS = 'FETCH_USER_SUCCESS';
export const FETCH_USER_FAILURE = 'FETCH_USER_FAILURE';
export const fetchUser = (userId: string) => {
return async (dispatch: Dispatch) => {
dispatch({ type: FETCH_USER_REQUEST });
try {
const userData = await fetchUserData(userId);
dispatch({ type: FETCH_USER_SUCCESS, payload: userData });
} catch (error) {
dispatch({ type: FETCH_USER_FAILURE, error });
}
};
};
This action creator demonstrates how to handle asynchronous operations within the Redux ecosystem.
Conclusion
generator-redux-app is a powerful tool that significantly reduces the time and effort required to set up a Redux application. By providing a well-structured boilerplate and integrating essential libraries like React Router and Jest, it allows developers to focus on building features rather than configuration.
Whether you’re starting a new project or looking to standardize your Redux setup across multiple applications, generator-redux-app offers a robust and flexible foundation. As you become more familiar with the generated structure, you’ll find it easy to customize and extend to meet the specific needs of your projects.
By leveraging generator-redux-app, you’re not just saving time; you’re adopting a set of best practices that can lead to more maintainable and scalable Redux applications. Happy coding!