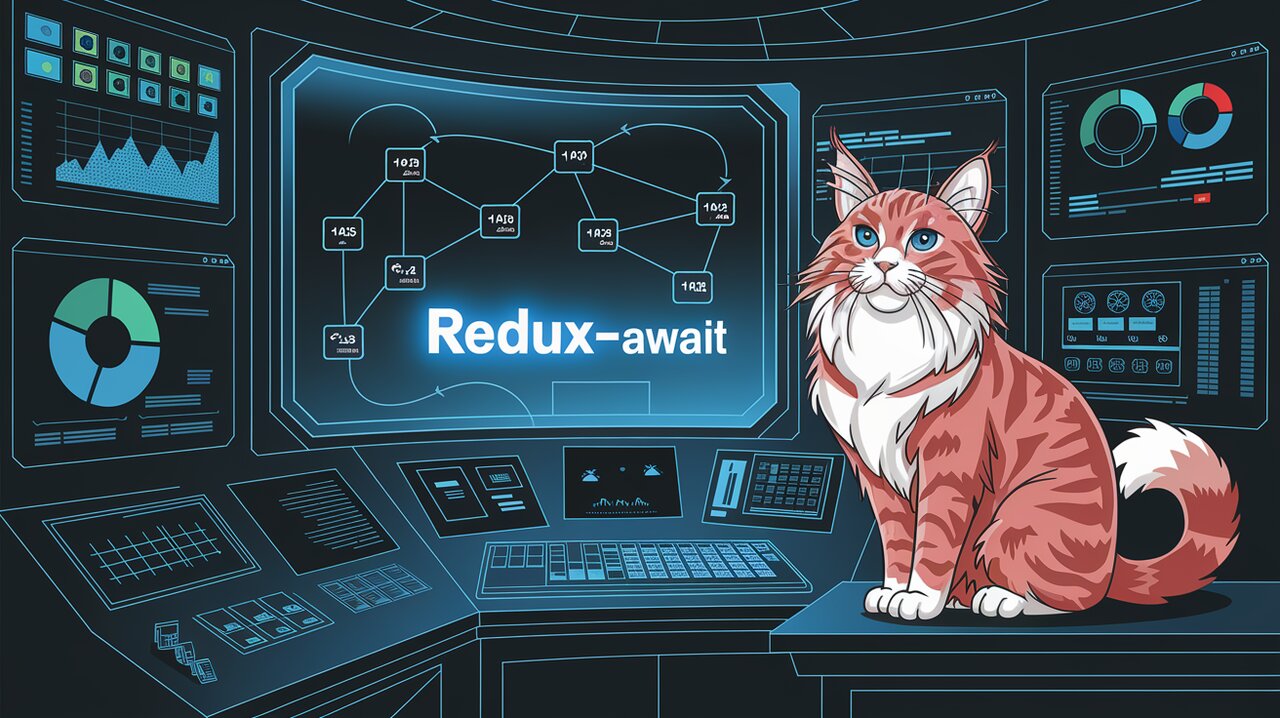
Redux-Await: Orchestrating Async Actions with Elegance
In the ever-evolving landscape of React development, managing asynchronous operations within Redux can often feel like conducting a complex symphony. Enter Redux-Await, a powerful library that promises to harmonize your async Redux actions with the grace of a seasoned conductor. Let’s dive into how this library can transform your React applications and simplify your state management.
The Overture: Understanding Redux-Await
Redux-Await is designed to tackle one of the most common challenges in Redux applications: handling asynchronous actions elegantly. By providing a middleware and reducer combination, it allows developers to incorporate promises directly into their action payloads, streamlining the process of tracking async statuses and errors.
Setting the Stage: Installation and Setup
Before we can start our async symphony, we need to set up our orchestra. Let’s begin by installing redux-await
:
npm install redux-await
# or
yarn add redux-await
With the library installed, it’s time to integrate it into your Redux setup:
Applying the Middleware
First, we need to apply the redux-await
middleware to our store:
import { createStore, applyMiddleware, combineReducers } from 'redux';
import { middleware as awaitMiddleware } from 'redux-await';
const createStoreWithMiddleware = applyMiddleware(awaitMiddleware)(createStore);
Incorporating the Reducer
Next, we’ll add the redux-await
reducer to our root reducer:
import { reducer as awaitReducer } from 'redux-await';
const rootReducer = combineReducers({
// Your other reducers
await: awaitReducer,
});
const store = createStoreWithMiddleware(rootReducer);
The First Movement: Basic Usage
With our setup complete, let’s explore how to use Redux-Await in action. The key is to include the AWAIT_MARKER
in your actions that contain promises:
import { AWAIT_MARKER } from 'redux-await';
const getTodos = () => ({
type: 'GET_TODOS',
AWAIT_MARKER,
payload: {
todos: api.getTodos(), // Returns a promise
},
});
In this example, api.getTodos()
is an asynchronous call that returns a promise. Redux-Await will automatically handle the promise lifecycle, updating the state with pending, success, or failure statuses.
The Second Movement: Connecting Components
To leverage the power of Redux-Await in your components, you’ll need to use its custom connect
function:
import { connect } from 'redux-await';
const TodoList = ({ todos, statuses, errors }) => {
if (statuses.todos === 'pending') {
return <div>Loading...</div>;
}
if (statuses.todos === 'failure') {
return <div>Error: {errors.todos.message}</div>;
}
return (
<ul>
{todos.map(todo => <li key={todo.id}>{todo.title}</li>)}
</ul>
);
};
export default connect(state => state.todos)(TodoList);
This connect
function automatically spreads the await
state onto your component’s props, giving you easy access to statuses and errors for each async action.
The Third Movement: Advanced Techniques
As you become more comfortable with Redux-Await, you can explore more advanced usage patterns:
Multiple Async Actions
You can include multiple async operations in a single action:
const syncUser = (userId) => ({
type: 'SYNC_USER',
AWAIT_MARKER,
payload: {
profile: api.getUserProfile(userId),
preferences: api.getUserPreferences(userId),
},
});
Chaining Async Actions
For more complex workflows, you can chain async actions using Redux-Thunk:
import { AWAIT_MARKER } from 'redux-await';
const complexOperation = () => async (dispatch) => {
await dispatch({
type: 'FIRST_OPERATION',
AWAIT_MARKER,
payload: {
result: api.firstOperation(),
},
});
dispatch({
type: 'SECOND_OPERATION',
AWAIT_MARKER,
payload: {
finalResult: api.secondOperation(),
},
});
};
The Finale: Best Practices and Considerations
As we conclude our exploration of Redux-Await, let’s consider some best practices:
-
Descriptive Payload Properties: Use clear, descriptive names for your payload properties. This helps in tracking statuses and errors more effectively.
-
Error Handling: Always account for potential failures in your components by checking the error states provided by Redux-Await.
-
Performance: For large applications, be mindful of the number of async actions you’re tracking. Consider cleaning up resolved actions when they’re no longer needed.
-
TypeScript Integration: While our examples use TypeScript, Redux-Await works seamlessly with both JavaScript and TypeScript projects.
Encore: Complementary Libraries
To further enhance your Redux experience, consider exploring these complementary libraries:
- Redux-Saga: For more complex async flow management.
- Redux-Observable: If you prefer a reactive programming approach with RxJS.
Redux-Await orchestrates a beautiful symphony of async state management in your React applications. By simplifying the handling of promises in Redux actions, it allows developers to focus on creating rich, responsive user experiences without getting bogged down in the complexities of async state tracking.
As you implement Redux-Await in your projects, you’ll find that managing asynchronous operations becomes less of a chore and more of a harmonious part of your development process. So go forth and conduct your async Redux symphony with confidence!