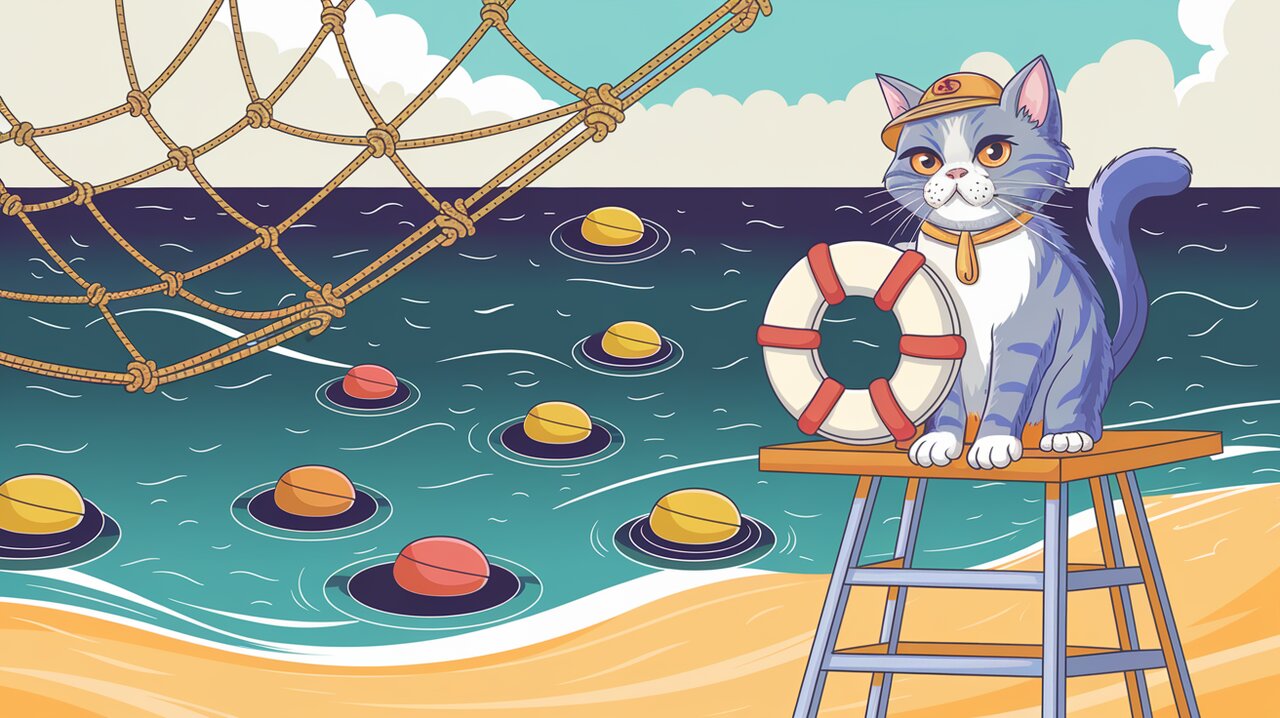
Catch the Redux Wave with redux-catch: Your Error Surfing Companion
In the world of Redux state management, handling errors gracefully can be as crucial as managing the state itself. redux-catch steps in as your reliable error-catching middleware, ensuring that your Redux application doesn’t wipe out when unexpected errors crash into your reducers or sync middlewares. Let’s dive into how this nifty tool can help you keep your application afloat and your debugging process streamlined.
Features That Make a Splash
- Error Interception: Catches errors in reducers and sync middlewares before they can sink your app.
- Custom Error Handling: Allows you to define your own error handling logic for maximum flexibility.
- State Snapshot: Provides access to the current state and last action at the time of the error.
- Dispatch Capability: Enables dispatching new actions in response to caught errors.
- Sentry Integration: Seamlessly works with Sentry for advanced error tracking and reporting.
Setting Sail with Installation
Before we can start catching errors, we need to get redux-catch
on board. You can install it using npm or yarn:
npm install redux-catch
# or
yarn add redux-catch
Basic Usage: Throwing Out the Life Preserver
Let’s start by implementing redux-catch
in its most basic form:
Applying the Middleware
import { createStore, applyMiddleware } from 'redux';
import reduxCatch from 'redux-catch';
import rootReducer from './reducers';
const errorHandler = (error: Error, getState: () => any, lastAction: any, dispatch: any) => {
console.error('Caught an exception!', error);
console.debug('Current state:', getState());
console.debug('Last action:', lastAction);
// Optionally dispatch a new action
};
const store = createStore(
rootReducer,
applyMiddleware(reduxCatch(errorHandler))
);
In this setup, we’re creating a simple error handler that logs the error, current state, and last action to the console. The reduxCatch
middleware is applied first in the middleware chain to ensure it can catch errors from all subsequent middlewares and reducers.
Understanding the Error Handler
The error handler function receives four parameters:
error
: The caught error object.getState
: A function to retrieve the current state.lastAction
: The action that was being processed when the error occurred.dispatch
: The store’s dispatch function, allowing you to dispatch new actions if needed.
This flexibility allows you to tailor your error handling to your specific needs, whether it’s logging, analytics, or triggering recovery actions.
Advanced Usage: Riding the Bigger Waves
Now that we’ve got our feet wet, let’s explore some more advanced uses of redux-catch
.
Integrating with Sentry
For production-grade error tracking, integrating with Sentry is a breeze:
import Raven from 'raven-js';
import { createStore, applyMiddleware } from 'redux';
import reduxCatch from 'redux-catch';
import rootReducer from './reducers';
// Configure Raven for Sentry
Raven.config('https://your-sentry-dsn@sentry.io/your-project').install();
const errorHandler = (error: Error, getState: () => any, lastAction: any) => {
Raven.context({
state: getState(),
action: lastAction,
});
Raven.captureException(error);
};
const store = createStore(
rootReducer,
applyMiddleware(reduxCatch(errorHandler))
);
This setup not only captures the error but also sends the current state and last action to Sentry, providing crucial context for debugging.
Custom Error Responses
You can use the dispatch
function to trigger specific actions in response to errors:
const errorHandler = (error: Error, getState: () => any, lastAction: any, dispatch: any) => {
console.error('An error occurred:', error);
if (error.message.includes('network')) {
dispatch({ type: 'SHOW_NETWORK_ERROR_NOTIFICATION' });
} else if (error.message.includes('validation')) {
dispatch({ type: 'SHOW_VALIDATION_ERROR', payload: error.message });
} else {
dispatch({ type: 'LOG_UNKNOWN_ERROR', payload: error });
}
};
This approach allows you to handle different types of errors in specific ways, enhancing your app’s error management capabilities.
Selective Error Catching
Sometimes, you might want to catch only specific types of errors:
const errorHandler = (error: Error, getState: () => any, lastAction: any, dispatch: any) => {
if (error instanceof CustomAppError) {
// Handle application-specific errors
console.error('Application error:', error.message);
dispatch({ type: 'HANDLE_APP_ERROR', payload: error });
} else if (error instanceof TypeError) {
// Handle type errors
console.error('Type error occurred:', error.message);
// Perform type error specific actions
} else {
// Handle other errors
console.error('Unexpected error:', error);
dispatch({ type: 'UNEXPECTED_ERROR', payload: error.message });
}
};
This pattern allows for more granular control over error handling, tailoring your responses to specific error types.
Conclusion: Smooth Sailing Ahead
redux-catch provides a robust safety net for your Redux applications, catching errors that might otherwise slip through the cracks. By implementing this middleware, you gain valuable insights into errors occurring in your reducers and sync middlewares, enabling quicker debugging and more resilient applications.
Whether you’re using it for simple console logging or integrating with advanced error tracking services like Sentry, redux-catch
offers the flexibility to handle errors in a way that best suits your application’s needs. So set sail with confidence, knowing that redux-catch
is there to keep your Redux waters calm and your application running smoothly.
For those looking to dive deeper into Redux error handling, you might also be interested in exploring how to handle async errors with Redux Thunk or how to manage complex state with Redux Toolkit. Happy coding, and may your Redux journeys be error-free!