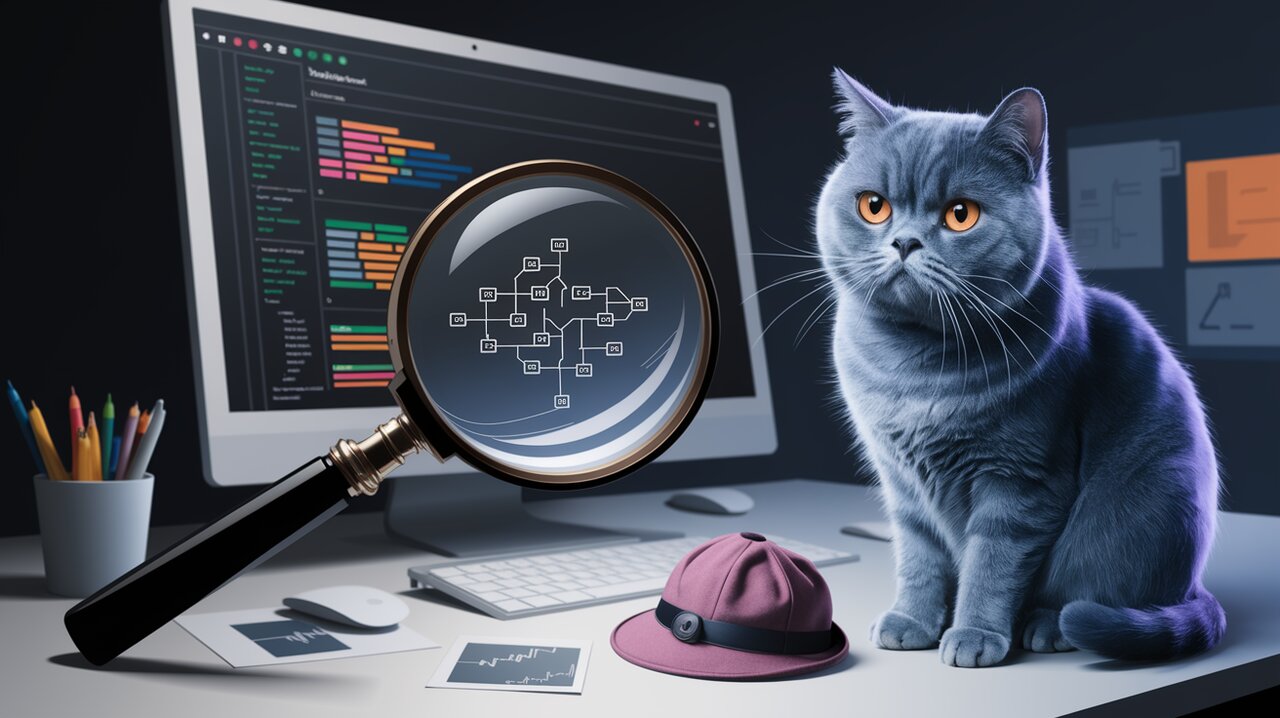
Redux-Debug: Your State's Private Investigator
In the intricate world of Redux state management, keeping track of state changes can sometimes feel like unraveling a complex mystery. That’s where Redux-Debug comes to the rescue, acting as your state’s personal detective. This powerful middleware brings the robust debugging capabilities of the popular debug()
function to your Redux applications, offering a seamless debugging experience that works flawlessly on both the server and the client.
Unveiling the Clues: Key Features of Redux-Debug
Redux-Debug comes packed with features that make it an indispensable tool for Redux developers:
-
Cross-environment Compatibility: Unlike some debugging tools that are limited to client-side use, Redux-Debug works seamlessly on both the server and in the browser.
-
Flexible Logging: While it’s designed to work with the
debug()
function, Redux-Debug can adapt to any sprintf-compatible function, giving you the flexibility to use your preferred logging method. -
Collapsible State Transitions: For those times when your state changes are numerous, Redux-Debug offers the option to collapse state transitions, keeping your logs clean and manageable.
-
Minimal Setup: With its straightforward API, Redux-Debug integrates into your Redux setup with just a few lines of code.
Setting Up Your Detective Kit: Installation
To start using Redux-Debug, you first need to add it to your project. You can do this using either npm or yarn:
npm install redux-debug
or
yarn add redux-debug
Basic Sleuthing: Getting Started with Redux-Debug
Once you’ve installed Redux-Debug, it’s time to integrate it into your Redux setup. Here’s a basic example of how to use it:
import { createStore, applyMiddleware, compose } from 'redux';
import Debug from 'redux-debug';
import debug from 'debug';
const reduxDebug = debug('redux');
const store = createStore(
rootReducer,
compose(
applyMiddleware(Debug(reduxDebug))
)
);
In this setup, we’re creating a debug instance with the namespace ‘redux’ and passing it to the Redux-Debug middleware. This middleware is then applied to our Redux store using applyMiddleware
.
Customizing Your Investigation
Redux-Debug allows you to customize its behavior through options. Here’s an example of how to use the collapsed
option to make your logs more compact:
const store = createStore(
rootReducer,
compose(
applyMiddleware(Debug(reduxDebug, { collapsed: true }))
)
);
This configuration will collapse the state transitions in your logs, which can be particularly useful when dealing with large or frequent state changes.
Advanced Detective Work: Leveraging Redux-Debug’s Full Potential
While the basic setup of Redux-Debug is powerful on its own, there are several advanced techniques you can use to enhance your debugging experience.
Using Custom Logging Functions
Although Redux-Debug is designed to work seamlessly with the debug()
function, you’re not limited to it. You can use any sprintf-compatible function as your logging method. Here’s an example using console.log
:
import { createStore, applyMiddleware, compose } from 'redux';
import Debug from 'redux-debug';
const store = createStore(
rootReducer,
compose(
applyMiddleware(Debug(console.log))
)
);
This setup will output your Redux state changes directly to the console, which can be useful in environments where the debug()
function isn’t available or when you prefer a simpler logging setup.
Selective Debugging with Namespaces
One of the powerful features of the debug()
function that Redux-Debug leverages is the ability to use namespaces. This allows you to selectively enable debugging for different parts of your application. Here’s how you might set this up:
import { createStore, applyMiddleware, compose } from 'redux';
import Debug from 'redux-debug';
import debug from 'debug';
const authDebug = debug('redux:auth');
const uiDebug = debug('redux:ui');
const store = createStore(
rootReducer,
compose(
applyMiddleware(
Debug(authDebug),
Debug(uiDebug)
)
)
);
With this setup, you can enable debugging for specific parts of your Redux store by setting the appropriate DEBUG environment variable or localStorage value. For example, to debug only authentication-related state changes, you would set DEBUG=redux:auth
.
Enhancing Readability with Custom Formatters
While Redux-Debug provides informative logs out of the box, you can enhance the readability of your logs by creating custom formatters. Here’s an example of how you might create a formatter that highlights certain types of actions:
import { createStore, applyMiddleware, compose } from 'redux';
import Debug from 'redux-debug';
import debug from 'debug';
const reduxDebug = debug('redux');
const customFormatter = (action: any, time: string, took: number) => {
const { type, ...payload } = action;
const formattedPayload = JSON.stringify(payload, null, 2);
return `Action: ${type.toUpperCase()}\nPayload: ${formattedPayload}\nTime: ${time}\nDuration: ${took}ms`;
};
const enhancedDebug = (message: string) => {
reduxDebug(customFormatter(...JSON.parse(message)));
};
const store = createStore(
rootReducer,
compose(
applyMiddleware(Debug(enhancedDebug))
)
);
This custom formatter separates the action type from the payload, uppercases the action type for emphasis, and pretty-prints the payload for better readability.
Wrapping Up the Investigation
Redux-Debug serves as an invaluable tool in your Redux development toolkit, offering a flexible and powerful way to gain insights into your application’s state changes. By leveraging its cross-environment compatibility, customizable logging, and integration with the robust debug()
function, you can significantly streamline your debugging process.
Whether you’re tracking down elusive bugs, optimizing performance, or simply trying to understand the flow of your application, Redux-Debug acts as your faithful detective, illuminating the inner workings of your Redux store. So why fumble in the dark when you can have a state-of-the-art investigative tool at your fingertips? Give Redux-Debug a try in your next project and experience the difference a good detective can make in solving your Redux mysteries.
For more insights into Redux and state management, you might want to check out our articles on Redux Rhapsody: Orchestrating React State and Redux DevTools: Time Travel Symphony.