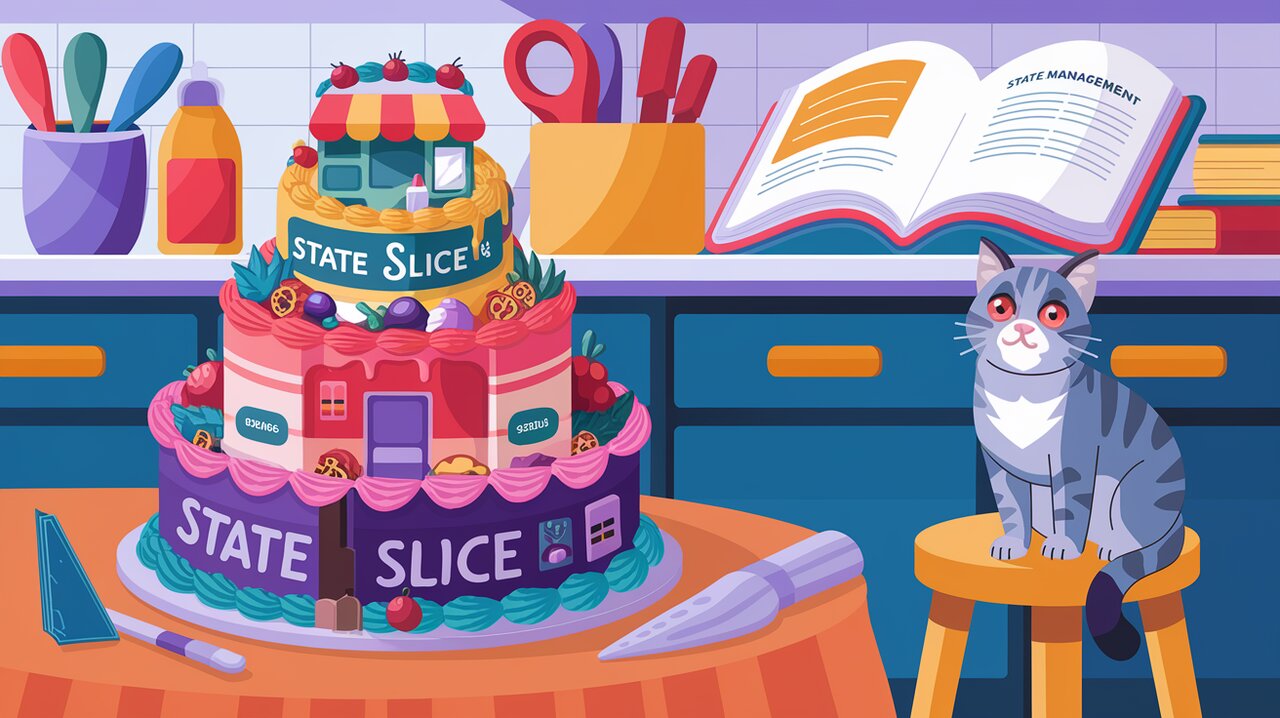
Redux Decorators: Sprinkling Syntactic Sugar on Your Redux Cake
Redux Decorators is a powerful library that brings a delightful syntax to Redux usage in ES7 and TypeScript environments. While primarily designed for Angular 2 applications, it offers a unique approach to state management that simplifies the Redux workflow. By leveraging decorators, this library allows developers to create more readable and maintainable code when working with Redux.
Sweet Features of Redux Decorators
Redux Decorators comes packed with a set of features that make state management a breeze:
- Slice Management: Easily define and manage specific slices of your state tree.
- Action Reducers: Simplify the creation and management of action reducers.
- Store Components: Effortlessly create components that observe store changes.
- Initial State Setup: Conveniently set up the initial state of your application.
- TypeScript Support: Full TypeScript support for type-safe Redux development.
Serving Up Redux Decorators
To start using Redux Decorators in your project, you can install it using npm or yarn:
npm install redux-decorators
Or if you prefer yarn:
yarn add redux-decorators
Baking the Basics
Let’s dive into some basic usage examples to get a taste of how Redux Decorators works.
Whipping Up a Reducer
First, let’s create a simple reducer using the @Slice
and @Reducer
decorators:
import { Slice, Reducer } from 'redux-decorators';
@Slice('count', 0)
@Reducer('increment', 'decrement')
export class CounterReducer {
increment(count: number) {
return count + 1;
}
decrement(count: number) {
return count - 1;
}
}
In this example, we’ve created a CounterReducer
class that operates on the ‘count’ slice of our state tree. The @Slice
decorator specifies the slice name and its initial value. The @Reducer
decorator registers the increment
and decrement
methods as action reducers.
Cooking Up a Store Component
Now, let’s create a component that interacts with our store:
import { Component } from '@angular/core';
import { Store } from 'redux-decorators';
@Component({
selector: 'app-counter',
template: `
<div>Count: {{count}}</div>
<button (click)="dispatch('increment')">Increment</button>
<button (click)="dispatch('decrement')">Decrement</button>
`
})
@Store('count')
export class CounterComponent {}
Here, we’ve used the @Store
decorator to register our CounterComponent
as a store observer. The ‘count’ property is automatically synced with the store’s ‘count’ slice.
Advanced Recipes
Let’s explore some more advanced usage of Redux Decorators.
Multiple Slices in One Reducer
While it’s generally recommended to keep reducers focused on a single slice, Redux Decorators allows for more complex scenarios:
import { Slice, Reducer } from 'redux-decorators';
@Slice('counter', { count: 0, lastUpdated: null })
@Reducer('increment', 'decrement', 'updateTimestamp')
export class AdvancedCounterReducer {
increment(state) {
return { ...state, count: state.count + 1 };
}
decrement(state) {
return { ...state, count: state.count - 1 };
}
@Slice('timestamp')
updateTimestamp(timestamp) {
return new Date().toISOString();
}
}
In this example, we’re managing a more complex state with a nested structure for the counter and a separate slice for the timestamp.
Custom Root Reducer
For more control over the reduction process, you can create a custom root reducer:
import { Reducer } from 'redux-decorators';
@Reducer()
class CustomRootReducer {
reducer(state = initialState, action) {
switch (action.type) {
case 'CUSTOM_ACTION':
return { ...state, customProperty: action.payload };
default:
return state;
}
}
}
This custom root reducer will override the default behavior, giving you full control over state updates.
The Icing on the Cake
Redux Decorators offers a unique and elegant approach to Redux integration in Angular applications. By leveraging the power of decorators, it simplifies many aspects of state management, from defining reducers to creating store-connected components. While it’s currently tailored for Angular 2+, the concepts and syntax improvements it brings to Redux are valuable for any developer looking to enhance their state management workflow.
Remember, while decorators provide a clean and intuitive API, they’re still an experimental feature in JavaScript. Always keep an eye on the latest ECMAScript specifications and consider the long-term maintainability of your codebase when adopting such patterns.
With Redux Decorators, you can enjoy a more streamlined Redux experience, allowing you to focus on building great features rather than wrestling with boilerplate code. Happy coding, and may your state always be predictable!