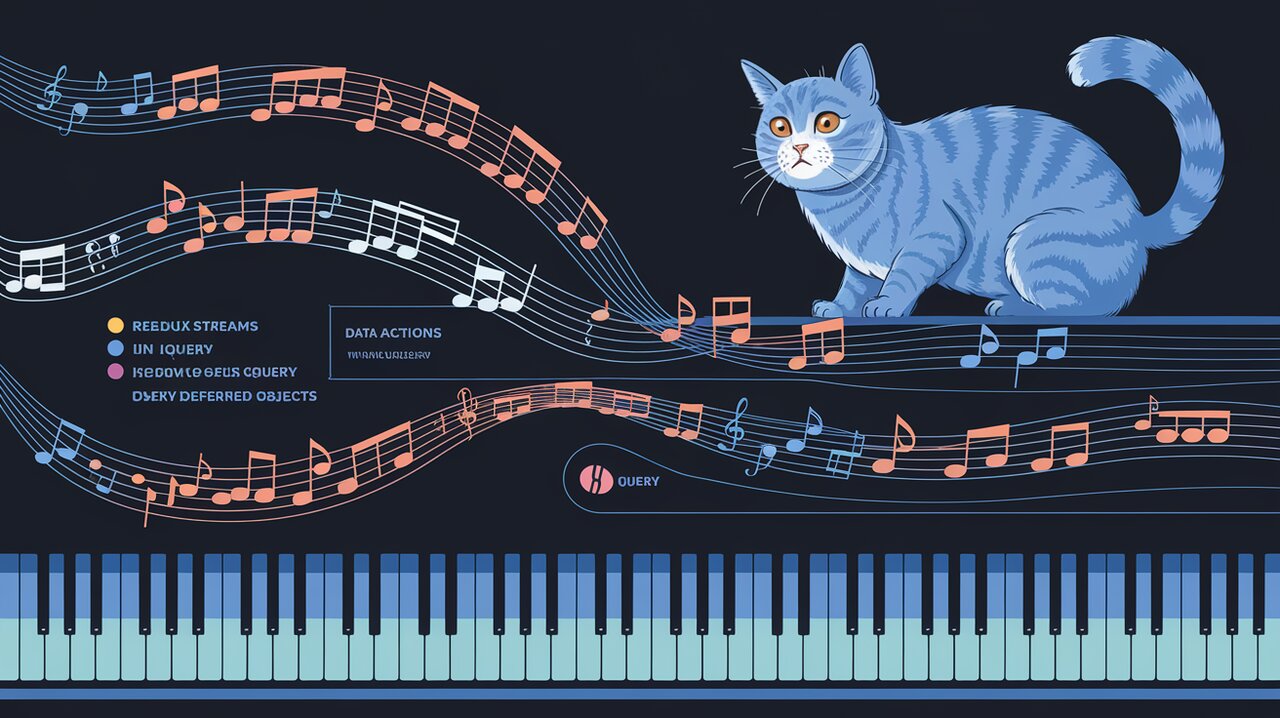
Redux Deferred: Orchestrating Asynchronous Actions with jQuery Harmony
Redux Deferred is a powerful middleware that brings the elegance of jQuery Deferred objects into the Redux ecosystem. This library simplifies the handling of asynchronous actions in React applications, providing a seamless bridge between Redux and jQuery’s promise-like Deferred objects. In this article, we’ll explore how redux-deferred can orchestrate your async workflows with finesse.
Understanding Redux Deferred
Redux Deferred is designed to work with jQuery’s Deferred objects, which are similar to Promises but with some unique characteristics. This middleware allows you to dispatch actions that contain Deferred objects, automatically handling their resolution or rejection and updating your Redux store accordingly.
Installation
To get started with redux-deferred, you’ll need to install it in your project. You can do this using npm or yarn:
npm install redux-deferred
or
yarn add redux-deferred
Basic Usage
Once installed, you can integrate redux-deferred into your Redux setup. Here’s how to apply the middleware:
import { createStore, applyMiddleware } from 'redux';
import DeferredMiddleware from 'redux-deferred';
const rootReducer = // your root reducer
const store = createStore(rootReducer, applyMiddleware(DeferredMiddleware));
With the middleware in place, you can now dispatch actions that contain Deferred objects.
Dispatching Deferred Actions
To use redux-deferred, you need to wrap your jQuery Deferred object in the payload of an action. Here’s an example:
import $ from 'jquery';
const loadData = () => ({
type: 'LOAD_DATA',
payload: $.get('https://api.example.com/data')
});
store.dispatch(loadData());
In this example, $.get()
returns a jQuery Deferred object. Redux Deferred will handle this action specially, waiting for the Deferred to resolve or reject before dispatching the final action.
Handling Results in Reducers
Your reducers can now handle both the successful and failed states of the asynchronous action:
const dataReducer = (state = { data: null, error: null }, action) => {
switch (action.type) {
case 'LOAD_DATA':
if (action.error) {
return { ...state, error: action.payload };
}
return { ...state, data: action.payload };
default:
return state;
}
};
When the Deferred object resolves, the action will be dispatched with the resolved value in the payload
. If it rejects, the error
property will be set to true
, and the payload
will contain the error details.
Advanced Usage
Redux Deferred shines in complex scenarios where you need to chain multiple asynchronous operations. You can leverage jQuery’s Deferred methods like then()
, done()
, and fail()
to create sophisticated async workflows:
const complexAction = () => ({
type: 'COMPLEX_ACTION',
payload: $.get('https://api.example.com/data')
.then(result => $.post('https://api.example.com/process', { data: result }))
.then(processedResult => ({ finalData: processedResult }))
});
store.dispatch(complexAction());
This action will perform a GET request, then a POST request with the result, and finally resolve with an object containing the processed data.
Error Handling
Redux Deferred makes error handling straightforward. When a Deferred object is rejected, the dispatched action will have an error
property set to true
:
const errorHandlingReducer = (state = { error: null }, action) => {
if (action.error) {
return { ...state, error: action.payload };
}
// Handle successful actions
return state;
};
This pattern allows you to centralize error handling in your reducers, making it easy to update your UI or perform error-specific logic.
Conclusion
Redux Deferred offers a elegant solution for managing asynchronous actions in Redux applications, especially those already using jQuery. By seamlessly integrating Deferred objects into the Redux flow, it simplifies complex async workflows and error handling.
While Redux Deferred is a powerful tool, it’s worth noting that modern JavaScript has evolved to favor native Promises and async/await syntax. For new projects, you might want to consider alternatives like redux-thunk or redux-saga that work with native Promises.
Redux Deferred remains a valuable tool for projects deeply integrated with jQuery, offering a bridge between older and newer asynchronous patterns in the Redux ecosystem.
By mastering Redux Deferred, you can create more responsive and robust React applications, handling complex asynchronous flows with ease and elegance.