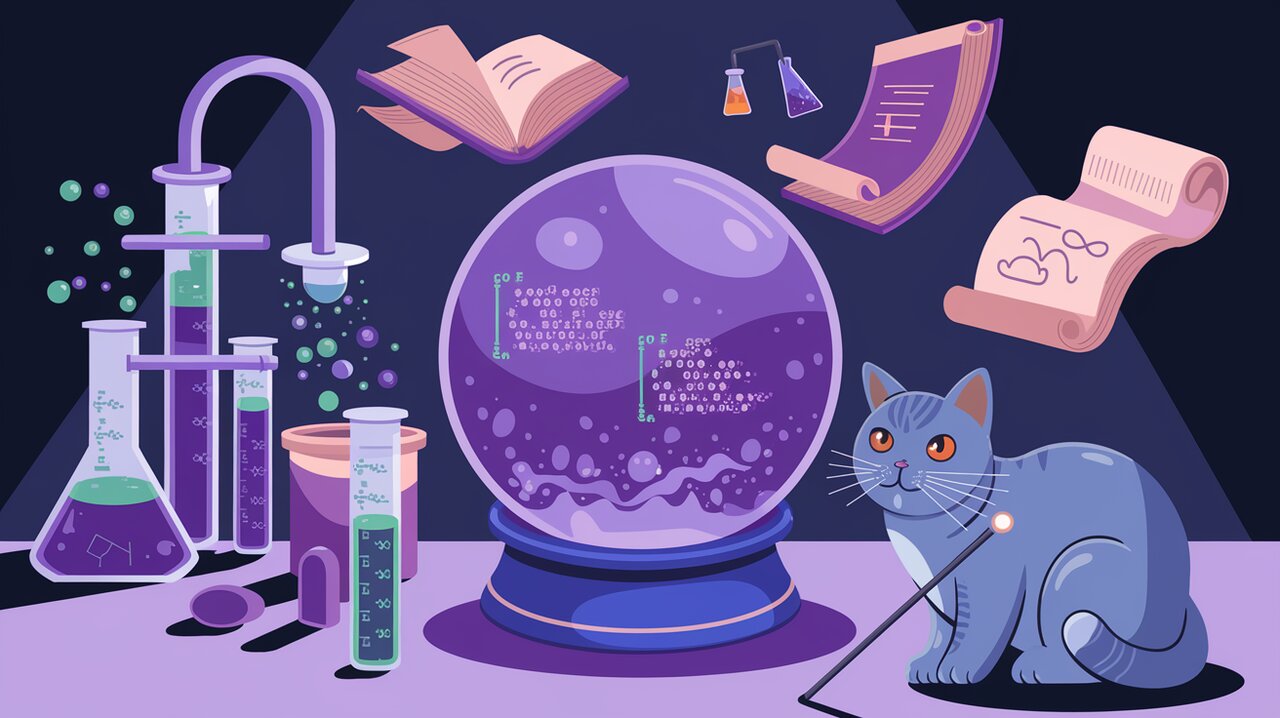
Redux DevTools GenTest Plugin: Conjuring Automated Tests from Thin Air
In the ever-evolving world of React and Redux development, ensuring the reliability and correctness of your application can be a daunting task. Enter Redux DevTools GenTest Plugin, a magical tool that transforms your Redux actions and state changes into comprehensive test suites with just a few clicks. This innovative plugin seamlessly integrates with Redux DevTools, allowing developers to generate tests automatically based on their application’s behavior.
Unveiling the Enchanted Features
Redux DevTools GenTest Plugin comes packed with a set of powerful features that will revolutionize your testing workflow:
- Automatic Test Generation: Create test cases based on your application’s actual state changes and actions.
- Seamless Integration: Works harmoniously with Redux DevTools, requiring minimal setup.
- Customizable Output: Tailor the generated tests to fit your project’s specific needs and testing framework.
- Real-time Preview: View and refine generated tests directly within the DevTools interface.
- Export Functionality: Easily export generated tests to your project’s test suite.
Summoning the Plugin
To bring the power of Redux DevTools GenTest Plugin into your project, you’ll need to perform a simple incantation. Open your terminal and cast the following spell:
npm install redux-devtools-gentest-plugin --save-dev
For those who prefer the mystical arts of Yarn, you can use this alternative chant:
yarn add redux-devtools-gentest-plugin --dev
Casting the Basic Spell
Once you’ve summoned the plugin, it’s time to weave it into your Redux DevTools setup. Here’s a basic incantation to get you started:
Conjuring the Test Monitor
First, import the magical components into your React application:
import { DevTools } from 'redux-devtools';
import { TestMonitor } from 'redux-devtools-gentest-plugin';
Next, incorporate the TestMonitor
into your DevTools configuration:
const App = () => (
<div>
<YourApp />
<DevTools store={store} monitor={TestMonitor} />
</div>
);
This enchantment will add a new panel to your Redux DevTools, allowing you to generate tests based on your application’s state changes.
Harvesting the Generated Tests
After interacting with your application and triggering various Redux actions, you’ll see a “Copy To Buffer” button appear in the TestMonitor panel. Clicking this button will copy the generated tests to your clipboard, ready to be pasted into your test suite.
Advanced Incantations
For those seeking to master the arcane arts of test generation, Redux DevTools GenTest Plugin offers more advanced techniques.
Customizing Test Output
You can tailor the generated tests to fit your project’s structure and testing framework. Here’s an example of how to customize the test output:
import { createStore } from 'redux';
import { DevTools, persistState } from 'redux-devtools';
import { TestMonitor, customizeTestGenerator } from 'redux-devtools-gentest-plugin';
const customGenerator = customizeTestGenerator({
importStatement: "import { rootReducer } from '../reducers';",
reducerName: 'rootReducer',
testFramework: 'jest'
});
const store = createStore(
rootReducer,
DevTools.instrument({ testGenerator: customGenerator })
);
This spell allows you to specify custom import statements, reducer names, and even choose your preferred testing framework.
Filtering Actions for Test Generation
Sometimes, you may want to focus your tests on specific actions. Here’s how you can apply a filter to the test generation process:
const actionFilter = (action) => action.type !== 'IGNORE_THIS_ACTION';
const store = createStore(
rootReducer,
DevTools.instrument({
testGenerator: customGenerator,
actionFilter
})
);
This incantation will exclude any actions with the type ‘IGNORE_THIS_ACTION’ from the generated tests.
Conclusion: Embracing the Magic of Automated Testing
Redux DevTools GenTest Plugin is a powerful ally in the quest for robust and reliable Redux applications. By automatically generating tests based on your application’s behavior, it not only saves valuable development time but also ensures comprehensive test coverage.
As you incorporate this magical tool into your workflow, you’ll find yourself spending less time writing boilerplate tests and more time crafting exceptional user experiences. The plugin’s ability to capture real-world interactions and transform them into test cases is nothing short of revolutionary.
Remember, while Redux DevTools GenTest Plugin provides an excellent starting point for your test suite, it’s still important to review and refine the generated tests to ensure they meet your specific requirements. Use this tool as a foundation upon which to build a robust and comprehensive testing strategy for your Redux applications.
Embrace the magic of automated test generation, and watch as your development process transforms into a more efficient and reliable journey. With Redux DevTools GenTest Plugin by your side, you’re well-equipped to face the challenges of modern React and Redux development with confidence and ease.
For those interested in exploring more React testing tools, you might want to check out our articles on Taming Errors with React Error Boundary and Redux Test Reducer: Simplifying Redux Testing. These complementary techniques can further enhance your testing arsenal and help you create more robust React applications.