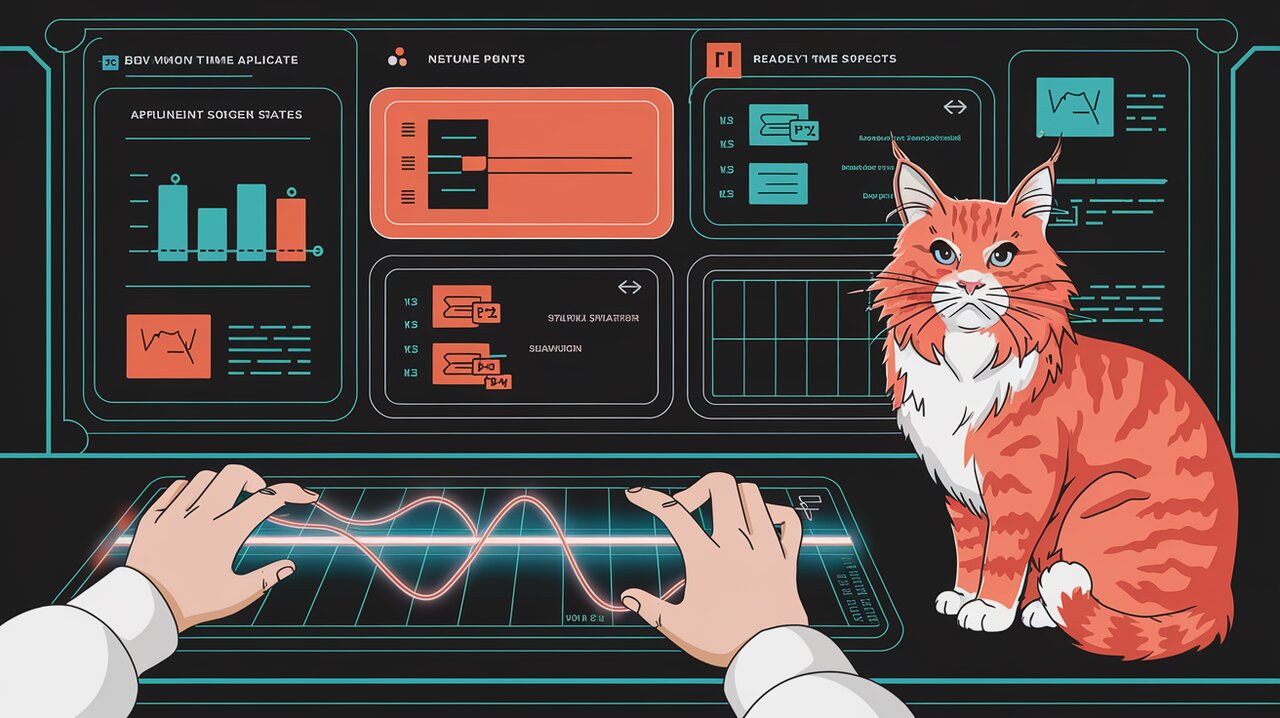
Redux DevTools Inspector: Orchestrating Time Travel in Your Redux Symphony
Redux DevTools Inspector is a powerful addition to the Redux ecosystem, offering developers an intuitive way to visualize and debug state changes in their React applications. This tool transforms the often complex task of state management into a symphonic experience, allowing developers to orchestrate their application’s behavior with precision and ease.
Tuning Your Redux Orchestra
At its core, Redux DevTools Inspector provides a suite of features designed to enhance the debugging process:
State Visualization: Easily inspect your Redux store’s state at any point in time. Action Replay: Step through dispatched actions to see how they affect the state. Time Travel Debugging: Jump to any previous state, making it simple to isolate and fix issues. Performance Monitoring: Track how long each action takes to process, helping identify bottlenecks.
These features work in harmony to provide a comprehensive view of your application’s state changes, much like a conductor’s score provides a complete view of an orchestra’s performance.
Setting the Stage
To begin using Redux DevTools Inspector, you’ll need to install it in your project. You can do this using npm or yarn:
npm install --save-dev @redux-devtools/extension
Or if you prefer yarn:
yarn add --dev @redux-devtools/extension
Composing Your Redux Symphony
Once installed, you’ll need to integrate Redux DevTools Inspector into your Redux store. Here’s how you can do that:
import { createStore, applyMiddleware, compose } from 'redux';
import { composeWithDevTools } from '@redux-devtools/extension';
import rootReducer from './reducers';
const store = createStore(
rootReducer,
composeWithDevTools(
applyMiddleware(...yourMiddleware)
// other store enhancers if any
)
);
This setup allows Redux DevTools Inspector to hook into your store, enabling it to track all state changes and actions dispatched in your application.
Conducting Time Travel
One of the most powerful features of Redux DevTools Inspector is its ability to perform time travel debugging. This allows you to move back and forth through your application’s state history, inspecting how each action affects the overall state.
Stepping Through Actions
In the Redux DevTools Inspector interface, you’ll see a list of all dispatched actions. You can click on any action to jump to that point in time, instantly updating your application’s state to reflect that moment.
// Example of an action you might see in the DevTools
const incrementAction = {
type: 'counter/increment',
payload: 1
};
By clicking on this action in the DevTools, you can see exactly how it affected your application’s state, making it easier to debug complex state changes.
Rewinding and Fast-Forwarding
Redux DevTools Inspector provides controls to step backwards and forwards through your action history. This is particularly useful when trying to isolate the cause of a bug:
- Use the “Step Backward” button to move to the previous action.
- Use the “Step Forward” button to move to the next action.
- Use the “Skip” button to jump multiple actions at once.
These controls give you fine-grained control over your application’s state timeline, much like a conductor controlling the tempo and progression of a musical piece.
Inspecting the State Score
As you navigate through your application’s history, Redux DevTools Inspector provides a detailed view of your state at each point in time.
State Diff View
One particularly useful feature is the “Diff” view, which shows you exactly what changed in your state as a result of each action:
{
todos: {
1: { id: 1, text: "Buy milk", completed: false },
2: { id: 2, text: "Walk the dog", completed: true }
}
}
After an action to complete a todo:
{
todos: {
1: { id: 1, text: "Buy milk", completed: true }, // Changed
2: { id: 2, text: "Walk the dog", completed: true }
}
}
The Diff view would highlight the change to the completed
field of the first todo, making it easy to see exactly what changed as a result of the action.
Monitoring Performance
Redux DevTools Inspector also includes tools to help you monitor the performance of your Redux operations. It tracks how long each action takes to process, helping you identify potential bottlenecks in your application.
// Example performance log
Action 'todos/addTodo' took 2.3ms
Action 'todos/toggleTodo' took 0.5ms
Action 'filter/setVisibilityFilter' took 0.1ms
This information can be invaluable when optimizing your application, allowing you to focus your efforts on the most time-consuming operations.
Advanced Techniques
Custom Action Creators
Redux DevTools Inspector works seamlessly with custom action creators, allowing you to see not just the raw actions, but also the parameters passed to your action creators:
const addTodo = (text) => ({
type: 'todos/addTodo',
payload: { text, completed: false, id: nextTodoId++ }
});
// In your component
dispatch(addTodo('Learn Redux'));
In the DevTools, you’ll be able to see both the resulting action and the original text
parameter passed to addTodo
.
Tracing Action Origins
Redux DevTools Inspector includes a “Trace” tab that shows you where each action was dispatched from in your codebase. This can be incredibly helpful when trying to understand the flow of your application:
// Example trace output
Action 'todos/addTodo' was dispatched from:
AddTodo.js:24
TodoList.js:57
App.js:12
This trace allows you to quickly navigate to the relevant parts of your code, speeding up the debugging process.
Harmonizing with Other Tools
Redux DevTools Inspector plays well with other Redux ecosystem tools. For instance, it can be used alongside libraries like Redux Toolkit to provide an even more powerful development experience.
You might find it helpful to explore how Redux DevTools Inspector complements other state management solutions. For example, our article on Orchestrating React State with Blue-Chip discusses another approach to state management that could be used in conjunction with Redux and Redux DevTools Inspector.
Additionally, for those interested in other Redux-related tools, our article on Buzzing with Redux-Bees explores a library for handling API calls in Redux applications, which could be debugged effectively using Redux DevTools Inspector.
The Final Movement
Redux DevTools Inspector is a powerful ally in the development of React applications using Redux. By providing intuitive visualization of state changes, powerful time-travel debugging capabilities, and performance insights, it allows developers to orchestrate complex state management with the precision of a master conductor.
As you continue to explore and use Redux DevTools Inspector, you’ll likely discover even more ways it can enhance your development workflow. Whether you’re debugging a complex state issue, optimizing performance, or simply trying to understand the flow of your application better, Redux DevTools Inspector provides the tools you need to create a harmonious Redux symphony.
Remember, effective state management is key to building robust and maintainable React applications. With Redux DevTools Inspector in your toolkit, you’re well-equipped to tackle even the most complex state management challenges, ensuring your Redux orchestra plays in perfect harmony.