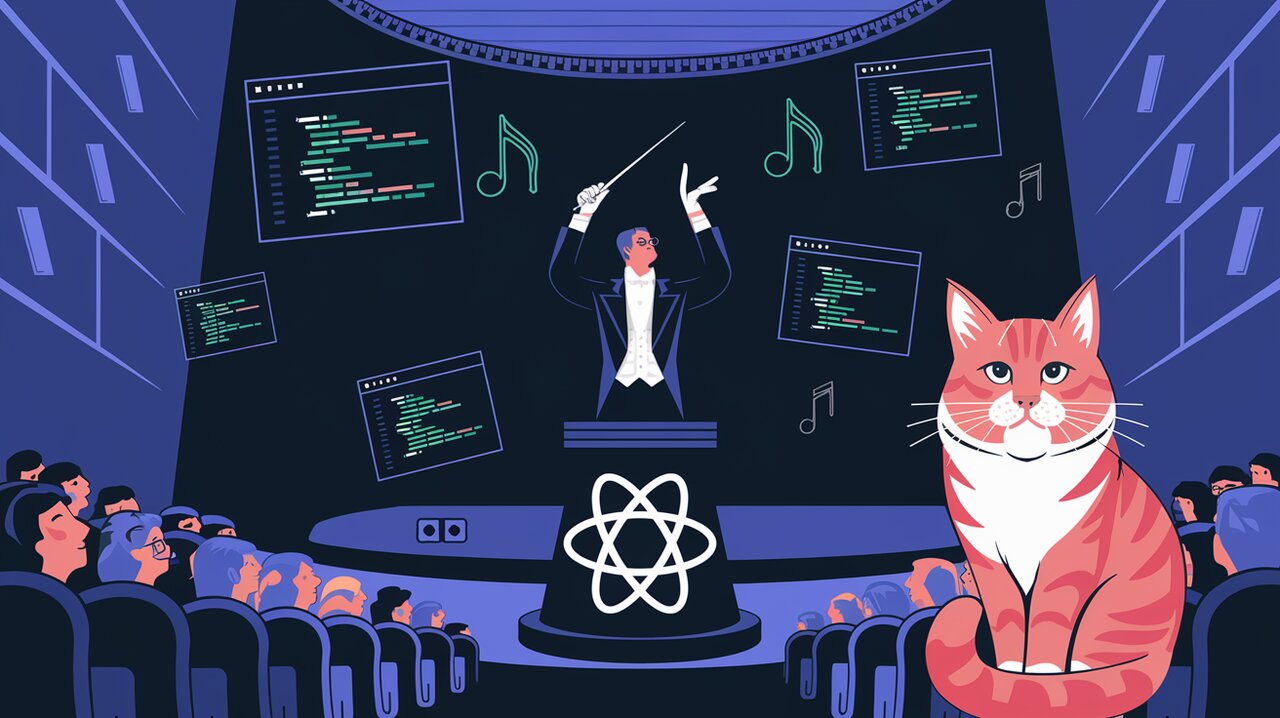
Orchestrating Redux Routes with redux-director: A Symphony of State and Navigation
Redux-director is a powerful library that brings together the robust state management of Redux with the flexible routing capabilities of Director. This combination allows developers to create React applications with seamless navigation and state control, all while maintaining a clean and organized codebase. Let’s dive into the world of redux-director and explore how it can elevate your React development experience.
Setting the Stage: Installation and Setup
Before we can start our redux-director symphony, we need to set up our environment. First, let’s install the necessary packages:
npm install director --save
npm install redux-director --save
With these libraries in place, we’re ready to begin integrating redux-director into our React application.
Connecting the Router to the Store
The first movement in our redux-director symphony is connecting the router to the Redux store. This crucial step allows the router to interact with your application’s state.
In your main application file, typically index.js
, add the following code:
import React from 'react';
import { render } from 'react-dom';
import { Provider } from 'react-redux';
import { Router } from 'redux-director';
import App from './containers/App';
import configureStore from './store/configureStore';
const store = configureStore();
Router.connect(store);
render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('app')
);
This code initializes your Redux store and connects the redux-director Router to it, ensuring that your routing and state management are in perfect harmony.
Composing Route Components
With our router connected, it’s time to create placeholders for our route components. These placeholders will be dynamically filled with the appropriate components based on the current route.
In your App.js
file, implement the following structure:
import React from 'react';
import { View } from 'redux-director';
import '../components/Routes';
const App: React.FC = () => {
return (
<div>
<View name="sidebar" />
<View name="main" />
</div>
);
};
export default App;
This setup creates two view placeholders: “sidebar” and “main”. These will be populated with components defined in your routes.
Orchestrating Routes
Now, let’s define our routes. Create a Routes.js
file to set up the routing configuration:
import React from 'react';
import { Router } from 'redux-director';
import Home from './Home';
import Contact from './Contact';
import MyPage from './MyPage';
import Sidebar from './Sidebar';
const sidebar = () => <Sidebar />;
const mymw = (ctx: any, next: () => void) => {
console.log("store state", ctx.state, ctx);
next();
};
const routes = {
"home": {
pattern: "",
components: {
main: () => <Home />,
sidebar: sidebar
}
},
"myvariableroute": {
pattern: "/route/:var",
components: {
main: (routerstate: any) => (
<MyPage var={routerstate.params.var} />
),
sidebar: sidebar
},
data: {
myfield: "myvalue"
}
},
"contact": {
pattern: "/contact",
middlewares: [mymw],
components: {
main: () => <Contact />,
sidebar: sidebar
}
}
};
Router.setRoutes(routes, 'home');
This configuration defines three routes: home, a variable route, and a contact route. Each route specifies which components should be rendered in the “main” and “sidebar” views.
Enhancing with Middleware
Redux-director allows you to add middleware to your routes, providing a powerful way to execute code before a route is rendered. In the example above, we’ve added a simple middleware function to the “contact” route that logs the store state.
Navigating Your Application
With our routes set up, navigation becomes straightforward. You can use simple <a>
tags with hash-based URLs:
import React from 'react';
const Sidebar: React.FC = () => (
<div>
Sidebar
<ul>
<li><a href="#/">home</a></li>
<li><a href="#/contact">contact</a></li>
<li><a href="#/route/Title">MyPage-Main</a></li>
</ul>
</div>
);
export { Sidebar };
Finale: Integrating with Redux
To complete our redux-director symphony, we need to add the router reducer to our Redux store. In your reducers/index.js
file:
import { combineReducers } from 'redux';
import { Reducer as routerReducer } from 'redux-director';
const rootReducer = combineReducers({
router: routerReducer,
// ... other reducers
});
export default rootReducer;
This integration ensures that your routing state is managed within your Redux store, allowing for a unified state management approach.
Encore: Advanced Features
Redux-director offers additional methods for more complex routing scenarios:
Router.redirect(url)
: Programmatically navigate to a new URL.Router.dispatch(url)
: Trigger a route change without updating the browser URL.
These methods provide fine-grained control over your application’s routing behavior.
Conclusion: A Harmonious React Application
Redux-director brings together the power of Redux state management and flexible routing, creating a symphony of seamless navigation and state control in your React applications. By following this guide, you’ve learned how to orchestrate routes, integrate with Redux, and leverage middleware for enhanced functionality.
As you continue to explore redux-director, you’ll discover even more ways to fine-tune your application’s routing and state management. For more insights into React development, check out our articles on React hooks and state management with Jotai. Happy coding, and may your React applications always be in perfect harmony!