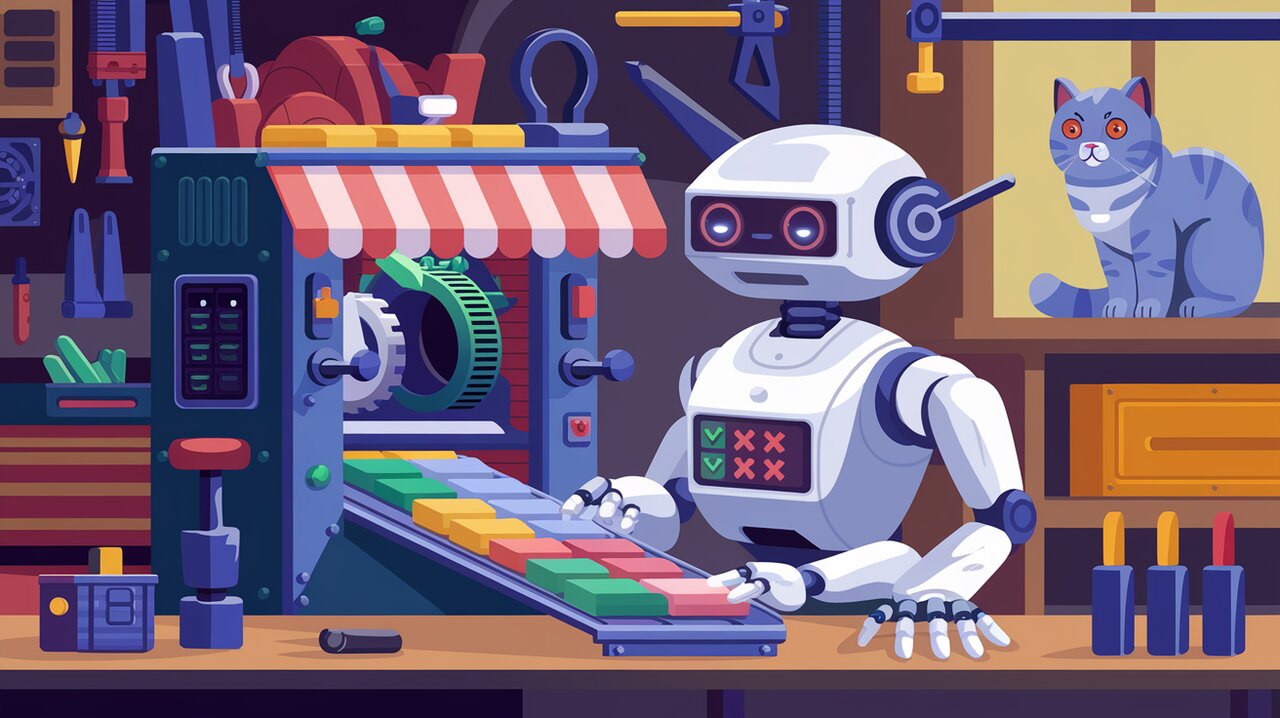
Redux FSA Guardian: Keeping Your Actions in Check
In the complex world of Redux state management, maintaining consistency in your action structures is paramount for a smooth and predictable application behavior. This is where Redux Ensure FSA comes to the rescue. The @meadow/redux-ensure-fsa
library serves as a vigilant guardian for your Redux actions, ensuring they adhere to the Flux Standard Action (FSA) protocol. Let’s dive into how this powerful middleware can enhance your development workflow and keep your Redux ecosystem clean and efficient.
What is Redux Ensure FSA?
Redux Ensure FSA is a middleware designed to validate actions in your Redux application. It checks whether each action dispatched through your Redux store complies with the Flux Standard Action protocol. This validation step is crucial for maintaining a consistent action structure throughout your application, which in turn leads to more predictable state changes and easier debugging.
Key Features
- FSA Compliance Checking: Automatically validates all actions against the FSA standard.
- Development-Only Usage: Designed to be used in development environments to catch non-compliant actions early.
- Customizable Ignore Function: Allows you to specify which actions should bypass the FSA check.
- Seamless Integration: Easily integrates with existing Redux middleware chains.
Setting Up Redux Ensure FSA
Installation
To get started with Redux Ensure FSA, you’ll need to install it in your project. You can do this using npm or yarn:
Using npm:
npm install @meadow/redux-ensure-fsa
Using yarn:
yarn add @meadow/redux-ensure-fsa
Basic Configuration
Once installed, you can integrate Redux Ensure FSA into your Redux middleware setup. Here’s a basic example of how to configure it:
import { createStore, applyMiddleware } from 'redux';
import ensureFSAMiddleware from '@meadow/redux-ensure-fsa';
import thunkMiddleware from 'redux-thunk';
import rootReducer from './reducers';
const middleware = [thunkMiddleware];
if (process.env.NODE_ENV !== 'production') {
const fsaMiddleware = ensureFSAMiddleware();
middleware.push(fsaMiddleware);
}
const store = createStore(
rootReducer,
applyMiddleware(...middleware)
);
In this setup, we’re adding the ensureFSAMiddleware
to our middleware array only in non-production environments. This ensures that our action validation doesn’t impact performance in production.
Understanding the Middleware’s Role
The ensureFSAMiddleware
acts as a gatekeeper for your actions. When an action is dispatched, it passes through this middleware, which checks if the action conforms to the FSA standard. If an action doesn’t comply, an error is thrown, alerting you to the issue immediately.
Advanced Usage
Customizing Action Validation
Sometimes, you might want to exclude certain actions from FSA validation. Redux Ensure FSA allows you to do this through a custom ignore function. Here’s how you can set it up:
import ensureFSAMiddleware from '@meadow/redux-ensure-fsa';
const customIgnoreFunction = (action: any) => {
// Ignore actions with a specific type
return action.type === 'SPECIAL_NON_FSA_ACTION';
};
const fsaMiddleware = ensureFSAMiddleware({
ignore: customIgnoreFunction
});
In this example, we’re creating a custom ignore function that excludes actions with the type ‘SPECIAL_NON_FSA_ACTION’ from FSA validation. This is particularly useful when working with third-party libraries or legacy code that might not follow the FSA standard.
Integrating with Other Middleware
Redux Ensure FSA plays well with other middleware. It’s typically best to place it at the end of your middleware chain to catch any non-FSA actions that might be produced by other middleware. Here’s an example of how you might set this up:
import { createStore, applyMiddleware } from 'redux';
import thunkMiddleware from 'redux-thunk';
import loggerMiddleware from 'redux-logger';
import ensureFSAMiddleware from '@meadow/redux-ensure-fsa';
import rootReducer from './reducers';
const middleware = [
thunkMiddleware,
loggerMiddleware,
];
if (process.env.NODE_ENV !== 'production') {
middleware.push(ensureFSAMiddleware());
}
const store = createStore(
rootReducer,
applyMiddleware(...middleware)
);
By placing ensureFSAMiddleware
at the end, we ensure that all actions, including those potentially modified by other middleware, are checked for FSA compliance.
Best Practices and Tips
Debugging with Redux Ensure FSA
When Redux Ensure FSA catches a non-compliant action, it throws an error. This can be incredibly helpful during development. Here’s a tip to make debugging even easier:
const fsaMiddleware = ensureFSAMiddleware({
ignore: (action) => false,
onError: (error, action) => {
console.error('Non-FSA Action detected:', action);
console.error('Error:', error.message);
// You could also log this to an error tracking service
}
});
By providing an onError
function, you can customize how non-FSA actions are handled, allowing for more detailed logging or integration with error tracking services.
Performance Considerations
While Redux Ensure FSA is a powerful tool for maintaining action consistency, it’s important to use it judiciously. Here are some performance tips:
- Development Only: Always ensure that the middleware is only active in development environments.
- Selective Checking: Use the ignore function to bypass checks for actions you’re certain are FSA-compliant.
- Order in Middleware Chain: Place it at the end of your middleware chain to minimize unnecessary checks.
Conclusion
Redux Ensure FSA is a valuable addition to any Redux developer’s toolkit. By enforcing Flux Standard Action compliance, it helps maintain a clean and predictable state management flow in your applications. From catching inconsistencies early in development to facilitating easier debugging, this middleware proves its worth in keeping your Redux ecosystem healthy and efficient.
By integrating @meadow/redux-ensure-fsa
into your project, you’re taking a proactive step towards more robust and maintainable Redux applications. Remember, consistent action structures lead to more predictable state changes, easier debugging, and ultimately, a smoother development experience. So, let Redux Ensure FSA be your guardian in the quest for Redux excellence!