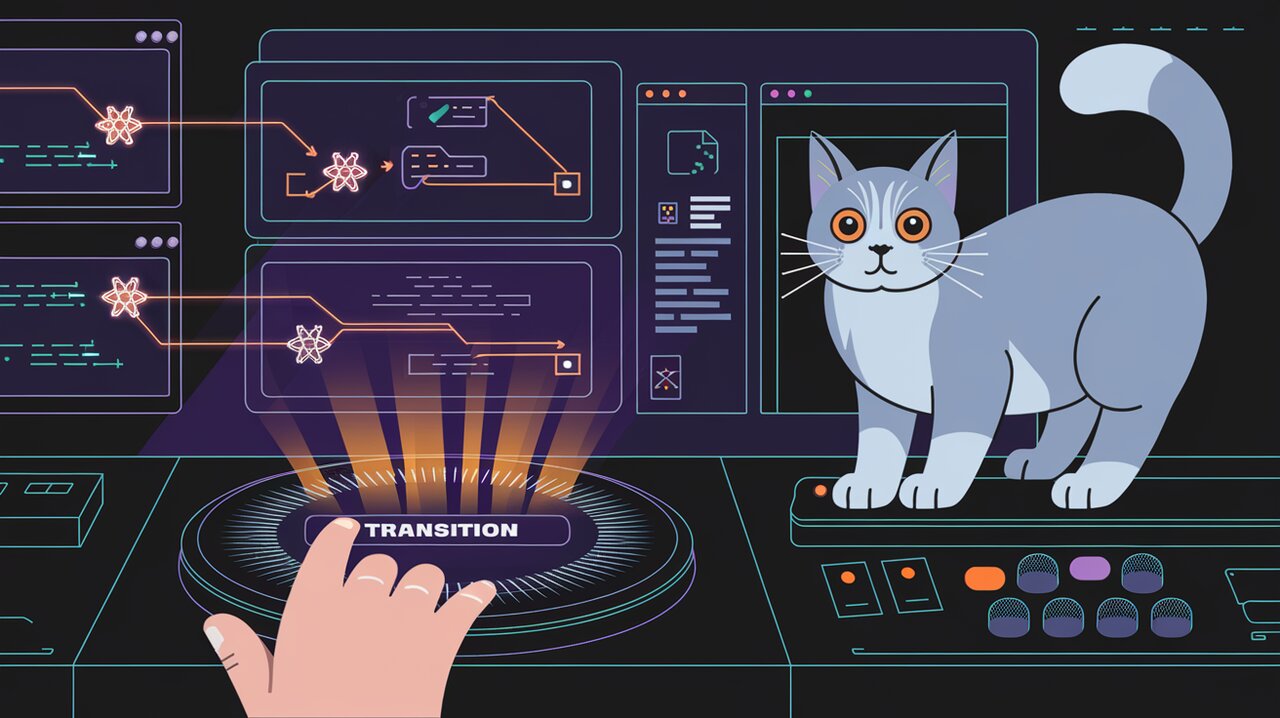
Time-Traveling with redux-history-transitions: Seamless Route Changes in React Apps
In the ever-evolving landscape of React applications, managing state and navigation can often feel like juggling while riding a unicycle. Enter redux-history-transitions, a powerful Redux store enhancer that promises to bring harmony to the chaos of route transitions and state management. This nifty library allows developers to co-locate transition logic with their actions, creating a more intuitive and maintainable codebase.
Navigating the Features
redux-history-transitions comes packed with features that make it a standout choice for developers looking to streamline their routing logic:
- Action-Driven Transitions: Trigger route changes directly from your Redux actions.
- Flexible History Support: Works with any routing system that uses the
history
library. - State-Aware Navigation: Access both previous and next state in transition handlers.
- Async-Friendly: Support for delayed transitions using Promises.
- Conditional Transitions: Easily implement success/failure routing logic for async actions.
Setting Sail with Installation
Before we dive into the deep end, let’s get redux-history-transitions installed in your project. Open your terminal and run one of the following commands:
npm install redux-history-transitions
# or if you're a yarn enthusiast
yarn add redux-history-transitions
Charting the Course: Basic Usage
Let’s start by enhancing our Redux store with the power of redux-history-transitions.
Creating an Enhanced Store
First, we’ll import the necessary ingredients and whip up an enhanced store:
import { createStore, compose } from 'redux';
import handleTransitions from 'redux-history-transitions';
import { createBrowserHistory } from 'history';
const history = createBrowserHistory();
const enhancer = handleTransitions(history);
const store = createStore(rootReducer, initialState, enhancer);
This setup allows our store to intercept actions with transition metadata and execute the appropriate navigation.
Crafting Transition-Enabled Actions
Now, let’s create an action that includes transition logic:
import { ActionCreator } from 'redux';
interface LoginAction {
type: 'LOGIN';
payload: {
userId: number;
};
meta: {
transition: (prevState: any, nextState: any, action: LoginAction) => {
pathname: string;
search?: string;
state?: any;
};
};
}
const login: ActionCreator<LoginAction> = (userId: number) => ({
type: 'LOGIN',
payload: { userId },
meta: {
transition: (prevState, nextState, action) => ({
pathname: `/dashboard/${action.payload.userId}`,
search: '?welcome=true',
state: { from: 'login' },
}),
},
});
In this example, when the login
action is dispatched, it will automatically trigger a navigation to the user’s dashboard after the state has been updated.
Advanced Maneuvers
Conditional Transitions
Sometimes you want different transitions based on the success or failure of an action. redux-history-transitions has got you covered:
const registerUser: ActionCreator<RegisterUserAction> = (username: string) => ({
type: 'REGISTER_USER',
payload: { username },
meta: {
done: true,
transition: {
success: (prevState, nextState, action) => ({
pathname: `/welcome/${action.payload.username}`,
}),
failure: (prevState, nextState, action) => ({
pathname: '/register',
search: '?error=true',
}),
},
},
});
This action will navigate to a welcome page on successful registration or back to the registration page with an error flag if it fails.
Delayed Transitions
For those times when you need to pause before transitioning, you can return a Promise:
import { Promise } from 'bluebird';
const delayedRedirect: ActionCreator<DelayedRedirectAction> = () => ({
type: 'DELAYED_REDIRECT',
meta: {
transition: (prevState, nextState, action) =>
Promise.delay(3000).then(() => ({
pathname: '/delayed-destination',
state: { message: 'You made it!' },
})),
},
});
This action will wait for 3 seconds before navigating, giving you time to show a loading indicator or animation.
Wrapping Up Our Journey
redux-history-transitions offers a elegant solution to the age-old problem of coordinating state changes with navigation in React applications. By allowing developers to define transitions alongside their actions, it promotes a more declarative and maintainable approach to routing logic.
Whether you’re building a simple app or a complex single-page application, this library can help you keep your codebase clean and your transitions smooth. It’s like having a skilled navigator aboard your React ship, ensuring you always reach your destination with style.
As you continue to explore the vast seas of React development, consider how redux-history-transitions might fit into your workflow. It could be the missing piece that turns your app from a rickety raft into a sleek yacht, gliding effortlessly between states and routes.
For those looking to dive deeper into Redux and routing, you might also want to check out our articles on navigating Redux seas with React Router and Redux observable cosmic symphony. These companion pieces can help you build a more comprehensive understanding of state management and routing in the React ecosystem.
Happy coding, and may your transitions always be smooth!