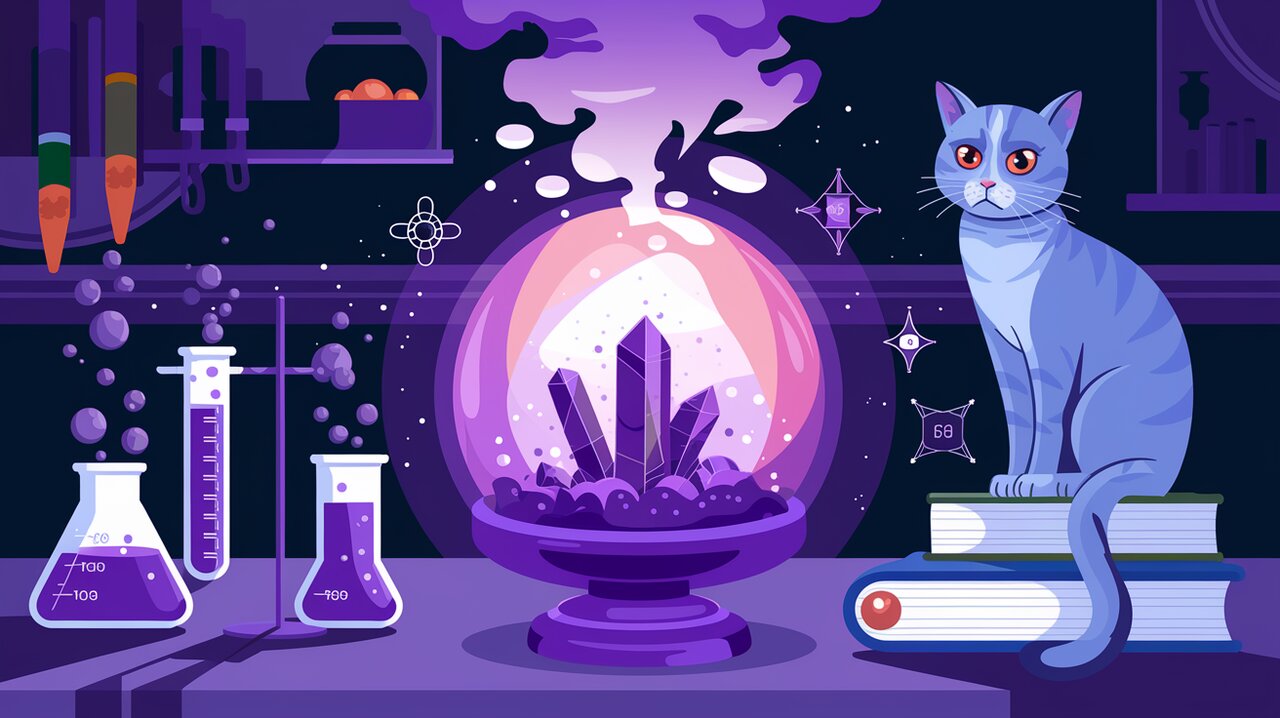
Redux Immutable Reducer: Conjuring Immutable State Magic
In the ever-evolving landscape of React and Redux applications, maintaining immutable state has become a crucial aspect of building robust and predictable user interfaces. Enter Redux Immutable Reducer, a powerful library that seamlessly integrates ImmutableJS with Redux, allowing developers to harness the full potential of immutable data structures without the hassle of constant conversions.
Unveiling the Magic of Immutable State
Redux Immutable Reducer solves a common pain point in Redux applications: the need for frequent .toJS()
and .fromJS()
calls when working with ImmutableJS and Redux together. By wrapping your reducers with this library, you can work directly with Immutable objects within your reducers while the rest of your application continues to interact with plain JavaScript objects.
Key Enchantments
- Seamless integration of ImmutableJS with Redux
- Automatic conversion between Immutable and plain JavaScript objects
- Cleaner and more readable state in Redux DevTools
- Simplified manipulation of complex state structures
Summoning the Library
To bring the power of Redux Immutable Reducer into your project, you’ll need to cast the following spells:
npm install --save redux-immutable-reducer
Or if you prefer the yarn incantation:
yarn add redux-immutable-reducer
Casting Your First Spell
Once installed, you can start using Redux Immutable Reducer in your project. Here’s how to summon its power:
import immutableReducer from 'redux-immutable-reducer';
const initialState = [1, 2, 3, 4];
function listReducer(state = initialState, action) {
switch (action.type) {
case 'ADD_ITEM':
return state.push(action.payload);
default:
return state;
}
}
export default immutableReducer(listReducer);
In this example, we’ve created a simple reducer that manages a list of numbers. By wrapping our listReducer
with immutableReducer
, we can use ImmutableJS methods like push
directly on the state, without worrying about mutating the original state.
Transforming Objects with Immutable Power
Redux Immutable Reducer isn’t limited to arrays. It’s equally potent when working with complex object structures:
import immutableReducer from 'redux-immutable-reducer';
const initialState = { user: { name: 'John', age: 30 } };
function userReducer(state = initialState, action) {
switch (action.type) {
case 'UPDATE_USER':
return state.mergeDeep({ user: action.payload });
default:
return state;
}
}
export default immutableReducer(userReducer);
Here, we’re using the mergeDeep
method to update nested properties of our state object. The immutableReducer
wrapper ensures that these operations are performed immutably, while still returning a plain JavaScript object to the rest of your application.
Advanced Incantations
Combining Multiple Immutable Reducers
When your application grows, you might want to combine multiple reducers, each managed by Redux Immutable Reducer. Here’s how you can achieve this:
import { combineReducers } from 'redux';
import immutableReducer from 'redux-immutable-reducer';
const listReducer = // ... as defined earlier
const userReducer = // ... as defined earlier
const rootReducer = combineReducers({
list: immutableReducer(listReducer),
user: immutableReducer(userReducer)
});
export default rootReducer;
This setup allows you to maintain immutable state for different parts of your application independently.
Custom Serialization
Sometimes, you might need to customize how your state is serialized. Redux Immutable Reducer allows you to pass custom serialization options:
import immutableReducer from 'redux-immutable-reducer';
import { Record } from 'immutable';
const UserRecord = Record({
name: '',
age: 0
});
function userReducer(state = UserRecord(), action) {
// ... reducer logic
}
export default immutableReducer(userReducer, {
serialize: (state) => state.toJS(),
deserialize: (state) => UserRecord(state)
});
This advanced usage allows you to work with Immutable Records while still maintaining compatibility with the rest of your Redux ecosystem.
Conclusion: Mastering the Art of Immutable State
Redux Immutable Reducer offers a powerful solution for developers looking to harness the benefits of immutable state in their Redux applications. By seamlessly integrating ImmutableJS with Redux, it eliminates the need for constant conversions and simplifies the process of working with complex state structures.
Whether you’re managing simple lists or deep, nested objects, this library provides the tools you need to maintain immutability throughout your reducers. As you continue to explore its capabilities, you’ll find that Redux Immutable Reducer not only simplifies your code but also enhances the overall predictability and maintainability of your React applications.
For those looking to dive deeper into the world of Redux and state management, you might also be interested in exploring related concepts such as Redux Saga for asynchronous flow or Zustand for simplified state management. These tools, combined with Redux Immutable Reducer, can form a powerful arsenal for tackling complex state management challenges in your React applications.