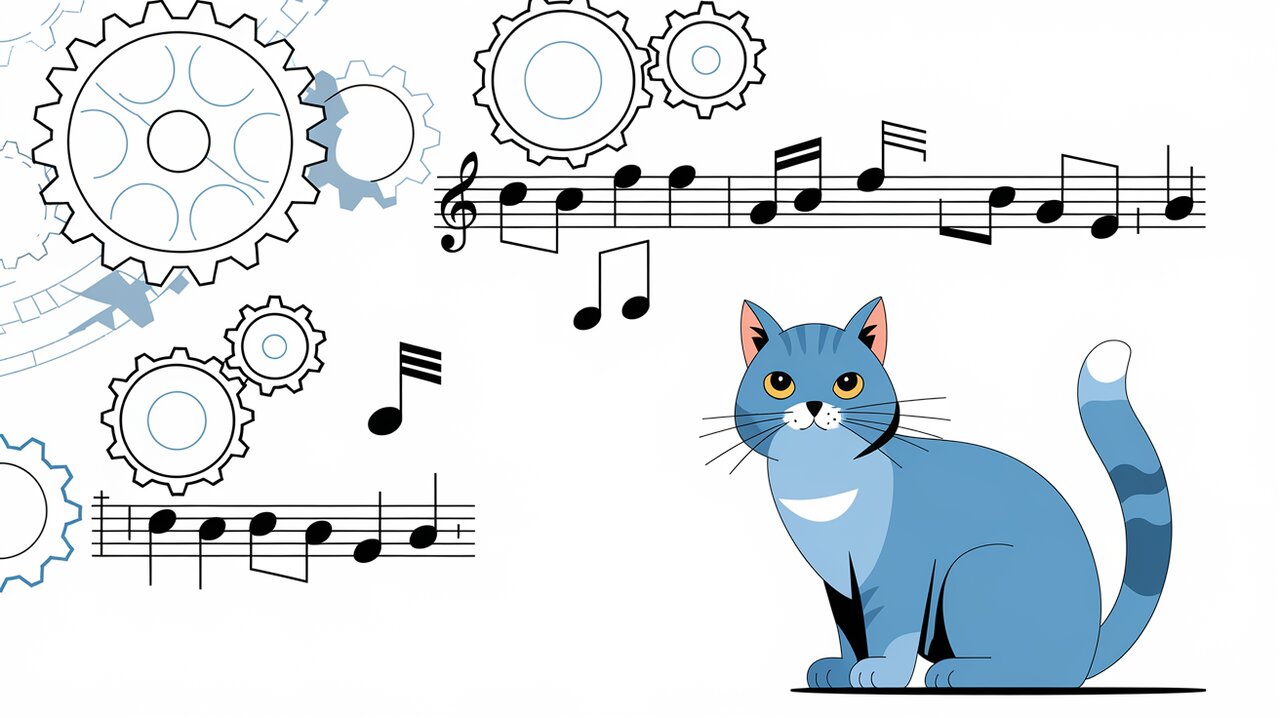
Redux-IO: Orchestrating Data Management in React Applications
Redux-IO is a powerful library designed to simplify data management in React applications using Redux. It provides a comprehensive set of tools for handling network data, making it easier to manage API interactions, normalize data in your Redux store, and optimize your application’s state management. By leveraging redux-io, developers can create more efficient and maintainable React applications with robust data handling capabilities.
Key Features of Redux-IO
Redux-IO comes packed with a variety of features that streamline data management:
- JSON-API support for standardized API interactions
- Data normalization in Redux store for efficient state management
- Asynchronous CRUD operations using Redux actions
- Optimistic updates for improved user experience
- Data denormalization for easier consumption in React components
- Built-in data caching mechanism with
reselect
compatibility - Flexible network resource configuration
- Comprehensive data status tracking and error handling
Setting Up Redux-IO
To get started with redux-io, you’ll need to install it in your project. You can do this using npm or yarn:
npm install @shoutem/redux-io
or
yarn add @shoutem/redux-io
Configuring Your Redux Store
Once installed, you’ll need to set up your Redux store to use redux-io. Here’s how you can configure your store:
import { createStore, applyMiddleware, combineReducers } from 'redux';
import { apiMiddleware } from 'redux-api-middleware';
import { apiStateMiddleware, storage, collection } from '@shoutem/redux-io';
import thunk from 'redux-thunk';
const rootReducer = combineReducers({
items: storage('acme.items'),
popularItems: collection('acme.items', 'popularItems'),
});
const store = createStore(
rootReducer,
applyMiddleware(thunk, apiMiddleware, apiStateMiddleware)
);
In this setup, we’re using the storage
and collection
reducers provided by redux-io. The storage
reducer is used to store normalized data, while the collection
reducer manages lists of item IDs.
Fetching Data with Redux-IO
Basic Data Fetching
Let’s look at how to fetch data using redux-io:
import { find } from '@shoutem/redux-io';
const fetchPopularItems = () => {
const config = {
endpoint: 'http://api.example.com/items?sort=popularity',
headers: {
'Content-Type': 'application/vnd.api+json',
},
};
return find(config, 'acme.items', 'popularItems');
};
// In your component
dispatch(fetchPopularItems());
This find
action will fetch popular items from the API and store them in your Redux state. The 'acme.items'
parameter specifies the schema, while 'popularItems'
is the tag for the collection.
Handling the Fetched Data
Redux-IO automatically handles the API response, normalizing the data and updating your Redux store. You can then access this data in your components:
import { isValid, isBusy, hasNext, next } from '@shoutem/redux-io';
import { getCollection } from '@shoutem/redux-io/reducers';
const mapStateToProps = (state) => ({
popularItems: getCollection(state.popularItems, state.items),
isLoading: isBusy(state.popularItems),
hasMoreItems: hasNext(state.popularItems),
});
// In your component
const { popularItems, isLoading, hasMoreItems } = props;
if (isLoading) {
return <LoadingSpinner />;
}
return (
<div>
{popularItems.map(item => <ItemComponent key={item.id} item={item} />)}
{hasMoreItems && <LoadMoreButton onClick={() => dispatch(next(state.popularItems))} />}
</div>
);
Advanced Usage
Optimistic Updates
Redux-IO supports optimistic updates, allowing you to update the UI immediately before the server responds:
import { create } from '@shoutem/redux-io';
const createItem = (itemData) => {
const config = {
endpoint: 'http://api.example.com/items',
headers: {
'Content-Type': 'application/vnd.api+json',
},
};
return create(config, 'acme.items', itemData);
};
// In your component
dispatch(createItem(newItemData));
The UI will update immediately with the new item, and redux-io will handle syncing with the server in the background.
Custom Network Configurations
You can customize how redux-io interacts with your API:
import { setStatus, updateStatus } from '@shoutem/redux-io';
const customApiCall = () => (dispatch) => {
dispatch(setStatus('acme.items', 'customOperation', { busy: true }));
return fetch('http://api.example.com/custom-endpoint')
.then(response => response.json())
.then(data => {
dispatch(updateStatus('acme.items', 'customOperation', { busy: false, error: false }));
// Process the data
})
.catch(error => {
dispatch(updateStatus('acme.items', 'customOperation', { busy: false, error }));
});
};
This allows you to integrate redux-io with custom API endpoints or operations while still benefiting from its status tracking and error handling capabilities.
Conclusion
Redux-IO offers a robust solution for managing network data in React applications. By providing tools for data normalization, asynchronous operations, and optimistic updates, it simplifies the often complex task of state management in large-scale applications. Whether you’re building a small project or a complex enterprise application, redux-io can help you create more efficient, maintainable, and performant React applications.
By leveraging redux-io’s features, you can focus more on building great user experiences and less on the intricacies of data management. As you continue to explore redux-io, you’ll discover even more ways to optimize your React and Redux workflow, making your development process smoother and more enjoyable.