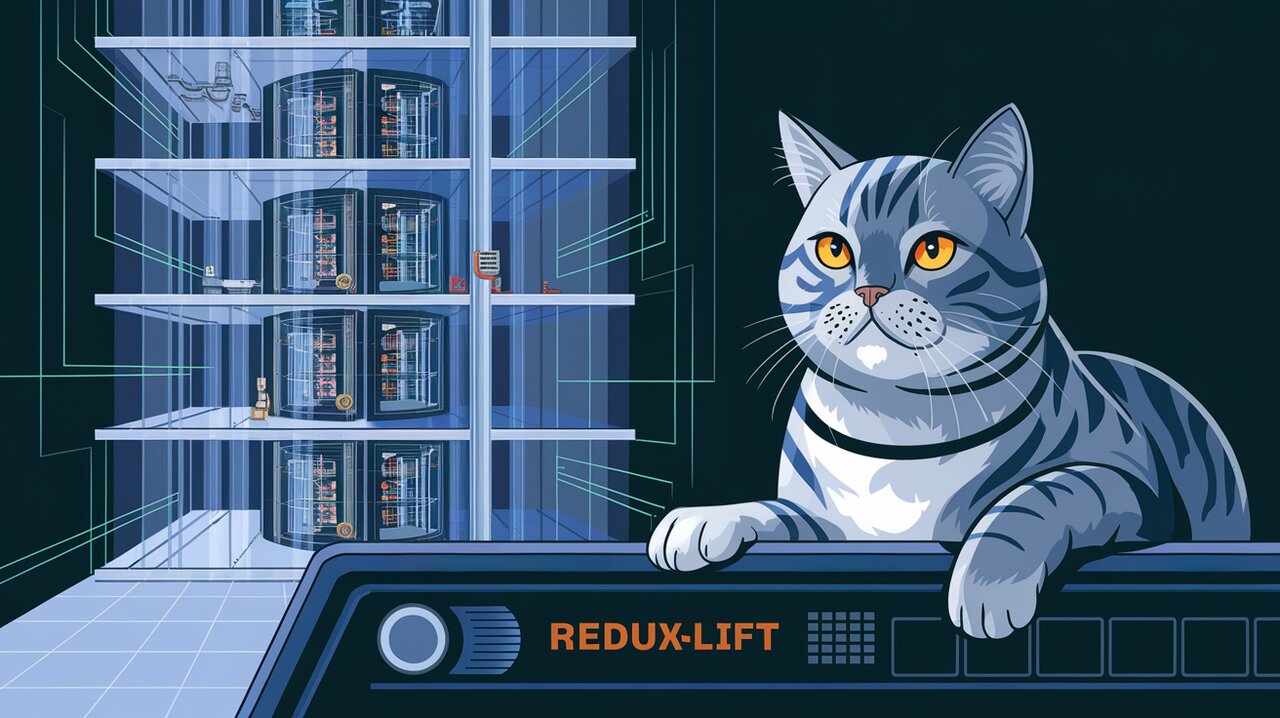
Redux-Lift: Elevating Your Redux State to New Heights
Redux-lift is a powerful extension to the Redux ecosystem that introduces a new concept called “lifting” to enhance state management in React applications. While Redux middleware is excellent for altering the behavior of the dispatch
function, it falls short when it comes to composing multiple parts of an application with different action or store needs. This is where redux-lift shines, offering a more flexible and powerful approach to store composition.
Elevating Your Redux Experience
At its core, redux-lift allows you to “lift” your state, reducers, and actions into another context. It’s a type of store enhancer that encompasses and extends the functionality of middleware. With redux-lift, you can manipulate the entire state tree, not just the dispatch function, opening up a world of possibilities for complex state management scenarios.
Key Features of Redux-Lift
- Comprehensive State Tree Manipulation: Unlike traditional middleware, redux-lift allows you to work with the entire state tree.
- Flexible Composition: Easily compose multiple parts of your application with different state and action requirements.
- Middleware Superset: Includes all the capabilities of middleware and more.
- Advanced Use Cases Support: Ideal for implementing time-travel debugging, server-side rendering state tracking, ephemeral UI state management, and multi-app Redux composition.
Installing Redux-Lift
To get started with redux-lift, you’ll need to install it in your project. You can do this using npm or yarn:
npm install redux-lift
or
yarn add redux-lift
Basic Usage: Lifting Your State
Let’s dive into how you can use redux-lift to enhance your Redux store. Here’s a basic example of how to create a lift:
import lift from 'redux-lift';
const myLift = lift({
liftState: (state) => ({ ...state, liftedProperty: 'I am lifted!' }),
liftReducer: (reducer) => (state, action) => {
const newState = reducer(state, action);
return { ...newState, reducerWasLifted: true };
},
liftDispatch: (dispatch) => (action) => {
console.log('Action dispatched:', action);
return dispatch(action);
}
});
const store = createStore(rootReducer, myLift);
In this example, we’re creating a lift that modifies the state, enhances the reducer, and logs actions when they’re dispatched. The liftState
function adds a new property to the state, liftReducer
modifies the behavior of the reducer, and liftDispatch
adds logging to the dispatch function.
Understanding the Lift Functions
Each lift function serves a specific purpose:
liftState
: Transforms the initial state of your store.liftReducer
: Enhances or modifies the behavior of your root reducer.liftDispatch
: Alters the dispatch function, similar to middleware.
Advanced Usage: Composing Multiple Lifts
One of the most powerful features of redux-lift is the ability to compose multiple lifts together. This allows you to create modular, reusable pieces of state management logic:
import lift from 'redux-lift';
const timeTravel = lift({
liftState: (state) => ({ ...state, history: [] }),
liftReducer: (reducer) => (state, action) => {
const newState = reducer(state, action);
return {
...newState,
history: [...state.history, { action, state: newState }]
};
}
});
const promiseTracker = lift({
liftState: (state) => ({ ...state, pendingPromises: [] }),
liftDispatch: (dispatch) => (action) => {
if (action.payload instanceof Promise) {
state.pendingPromises.push(action.payload);
action.payload.finally(() => {
state.pendingPromises = state.pendingPromises.filter(p => p !== action.payload);
});
}
return dispatch(action);
}
});
const composedLift = lift.compose(timeTravel, promiseTracker);
const store = createStore(rootReducer, composedLift);
In this advanced example, we’ve created two lifts: timeTravel
for implementing a simple time-travel debugging feature, and promiseTracker
for keeping track of pending promises in the state. We then compose these lifts together using lift.compose
to create a single, powerful lift that combines both functionalities.
Implementing Ephemeral UI State
Redux-lift can also be used to manage ephemeral UI state that doesn’t need to be persisted or included in time-travel debugging:
import lift from 'redux-lift';
import { connect } from 'react-redux';
const ephemeralLift = lift({
liftState: (state) => ({ ...state, ephemeral: {} }),
liftDispatch: (dispatch) => (action) => {
if (action.type === 'EPHEMERAL') {
return (state.ephemeral[action.key] = action.value);
}
return dispatch(action);
}
});
function ephemeral(key, initialValue) {
return connect(
(state) => ({ value: state.ephemeral[key] || initialValue }),
(dispatch) => ({
onChange: (value) => dispatch({ type: 'EPHEMERAL', key, value })
})
);
}
const EphemeralTextField = ephemeral('myTextField', '')(
({ value, onChange }) => (
<input
value={value}
onChange={(e) => onChange(e.target.value)}
type="text"
/>
)
);
This example demonstrates how to use redux-lift to manage ephemeral UI state, such as form inputs that don’t need to be part of the main Redux state.
Conclusion: Elevating Your Redux Architecture
Redux-lift provides a powerful toolset for enhancing and extending your Redux architecture. By allowing you to manipulate the entire state tree, compose multiple state management strategies, and handle advanced use cases like time-travel debugging and ephemeral state, redux-lift elevates your ability to manage complex application states.
Whether you’re building a large-scale application with multiple interconnected parts or simply looking to add more flexibility to your Redux setup, redux-lift offers a robust solution. Its ability to seamlessly integrate with existing Redux code while providing enhanced capabilities makes it an invaluable tool in any React developer’s arsenal.
As you explore the possibilities of redux-lift, you’ll find that it opens up new avenues for structuring your application’s state management, leading to more maintainable, modular, and powerful React applications.
For more insights into Redux and state management in React, you might want to check out our articles on Redux Rhapsody: Orchestrating React State and Mastering Jotai React State. These complementary pieces will give you a well-rounded understanding of state management techniques in the React ecosystem.