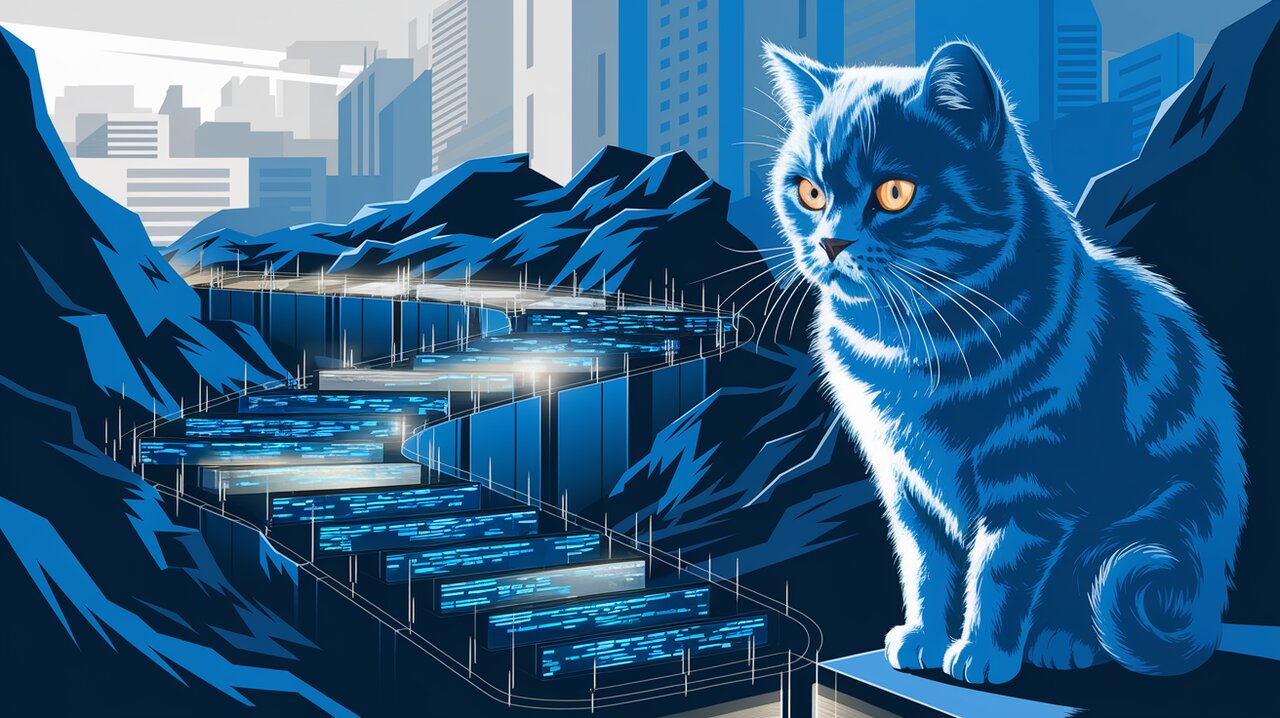
Redux-Most: Orchestrating Asynchronous Actions with Monadic Streams
Redux-Most is a powerful middleware for Redux that brings the elegance of monadic streams to the world of state management. By leveraging the capabilities of Most.js, Redux-Most enables developers to handle asynchronous actions with grace and precision, all while maintaining the simplicity and predictability that Redux is known for.
Unleashing the Power of Monadic Streams
At its core, Redux-Most is designed to work with the concept of “epics” - a pattern borrowed from redux-observable but tailored specifically for Most.js streams. This approach allows developers to treat async actions as streams of events, opening up a world of possibilities for complex state management scenarios.
Key Features
- Most.js Integration: Seamlessly works with Most.js, one of the fastest and most lightweight reactive programming libraries available.
- Epic Pattern: Utilizes the epic pattern for handling async actions, providing a clean and declarative way to manage side effects.
- Functional Approach: Encourages functional programming techniques like currying and partial application.
- Simplified API: Offers both a redux-observable-like API and a more declarative API for maximum flexibility.
Getting Started with Redux-Most
To begin your journey with Redux-Most, you’ll first need to install the library along with its peer dependencies:
npm install redux-most redux most
# or
yarn add redux-most redux most
Once installed, you can set up the middleware in your Redux store:
import { createStore, applyMiddleware } from 'redux';
import { createEpicMiddleware } from 'redux-most';
import rootEpic from './epics';
import rootReducer from './reducers';
const epicMiddleware = createEpicMiddleware(rootEpic);
const store = createStore(
rootReducer,
applyMiddleware(epicMiddleware)
);
Crafting Your First Epic
Epics are the heart of Redux-Most. They allow you to respond to actions and create streams of new actions. Here’s a simple example of an epic that responds to a user search action:
import { select, Epic } from 'redux-most';
import { from } from 'most';
import { SEARCH_USERS, searchUsersSuccess, searchUsersError } from './actions';
import { ajax } from './utils';
const searchUsers: Epic = (action$, store) =>
action$.thru(select(SEARCH_USERS))
.map(action => action.payload.query)
.chain(query =>
from(ajax.getJSON(`/api/users?q=${query}`))
.map(searchUsersSuccess)
.recoverWith(error => of(searchUsersError(error)))
);
export default searchUsers;
This epic listens for SEARCH_USERS
actions, extracts the query, makes an API call, and dispatches either a success or error action based on the result.
Advanced Usage: State Streams
Redux-Most offers an alternative API that provides epics with a stream of state changes instead of the store
object. This can be particularly useful for more declarative state management:
import { createEpicMiddleware, createStateStreamEnhancer } from 'redux-most';
import { withState } from 'redux-most';
const epicMiddleware = createEpicMiddleware(rootEpic);
const storeEnhancer = createStateStreamEnhancer(epicMiddleware);
const store = createStore(rootReducer, storeEnhancer);
// In your epic
const stateAwareEpic: Epic = (action$, state$) =>
action$.thru(select(SOME_ACTION))
.chain(action =>
state$.thru(withState(action))
.map(([state, action]) => {
// Use both state and action here
return someNewAction(state, action);
})
);
This approach allows you to react to both actions and state changes in a unified, stream-based manner.
Combining Epics
As your application grows, you’ll likely have multiple epics that need to work together. Redux-Most provides a combineEpics
utility to help manage this:
import { combineEpics } from 'redux-most';
import searchUsers from './searchUsers';
import updateProfile from './updateProfile';
import fetchNotifications from './fetchNotifications';
const rootEpic = combineEpics([
searchUsers,
updateProfile,
fetchNotifications
]);
export default rootEpic;
Performance Considerations
One of the key advantages of using Redux-Most is its performance. Most.js is known for its speed and efficiency, making it an excellent choice for applications that need to handle a high volume of asynchronous actions.
Conclusion
Redux-Most offers a powerful and elegant solution for managing asynchronous actions in Redux applications. By leveraging the strengths of Most.js and the epic pattern, it provides developers with a robust toolkit for building reactive, scalable applications.
Whether you’re building a small React app or a large-scale enterprise application, Redux-Most can help you manage complex state and side effects with ease. Its functional approach and stream-based paradigm make it a natural fit for modern JavaScript development practices.
As you continue your journey with Redux-Most, you might find it helpful to explore related concepts in the React ecosystem. For instance, you could look into how Redux-Saga orchestrates side effects or how Jotai simplifies state management for a different perspective on handling application state.
By mastering Redux-Most, you’ll be well-equipped to handle even the most complex asynchronous scenarios in your React applications, all while maintaining clean, readable, and performant code.