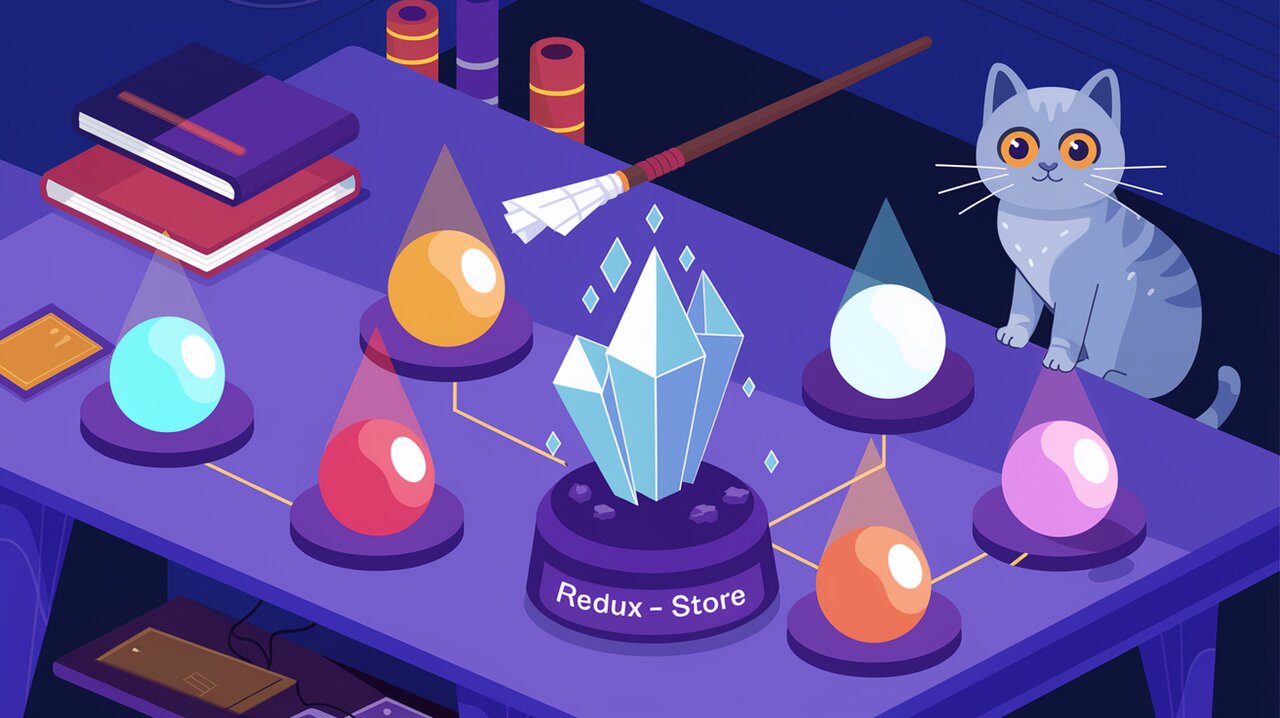
Redux-Mount: The Magical Wand for View-Specific State Management
In the enchanting realm of React and Redux, managing view-specific state can often feel like juggling flaming torches while riding a unicycle. Enter redux-mount, a magical wand that simplifies this complex act, allowing you to mount, unmount, and manipulate view-specific data with the grace of a seasoned sorcerer. Let’s embark on a journey to master this powerful tool and revolutionize your state management practices.
Unveiling the Magic of Redux-Mount
Redux-mount is a library that addresses a common challenge in single-page applications: handling view-specific state that may need to persist while navigating through the app. It provides an opinionated reducer, actions, and a helper for state selection, eliminating the need to create these elements yourself for every view.
Enchanting Features
- Path-based Mounting: Mount view-specific data on a specified path in your Redux store.
- Dynamic State Manipulation: Change mounted values on the fly without complex reducer logic.
- Persistent State: Keep view-specific state intact while navigating through your app.
- Simplified Selectors: Easily retrieve mounted data with a provided selector creator.
Summoning Redux-Mount
To begin your journey with redux-mount, you’ll need to summon it into your project. Open your magical terminal and chant one of the following incantations:
npm install redux-mount
Or if you prefer the yarn spell:
yarn add redux-mount
Casting Your First Spell
Let’s dive into the basic usage of redux-mount and see how it can simplify your state management rituals.
The Mounting Ceremony
First, we need to prepare our Redux store to work with redux-mount:
import { combineReducers } from 'redux';
import { reducer as mountReducer, createSelector } from 'redux-mount';
const MOUNT_STATE_KEY = '_mount';
const rootReducer = combineReducers({
[MOUNT_STATE_KEY]: mountReducer,
// Your other reducers...
});
// Create your Redux store with rootReducer
Now that our store is ready, let’s perform our first mounting spell:
import { actions } from 'redux-mount';
// Mount on a specific path
dispatch(actions.mount('user/profile'));
// Mount with initial data
dispatch(actions.mount('user/profile', { name: 'Merlin', role: 'Wizard' }));
In this incantation, we’re mounting view-specific data on the ‘user/profile’ path. The second example shows how to mount with initial data, perfect for when you have default values for your view.
Manipulating Mounted State
Once you’ve mounted your state, you can easily change it using the set
action:
dispatch(actions.set('role', 'Archmage'));
This spell will update the ‘role’ property of the currently mounted state. It’s that simple!
Retrieving Mounted State
To peek into your mounted state, use the selector created by createSelector
:
const mountSelector = createSelector(MOUNT_STATE_KEY);
const mountedData = mountSelector(state);
console.log(mountedData); // { name: 'Merlin', role: 'Archmage' }
This magical function allows you to easily retrieve the currently mounted data from your Redux state.
Advanced Sorcery
Now that we’ve mastered the basics, let’s delve into some more advanced redux-mount techniques.
The Unmounting Ritual
When you’re done with a particular view-specific state, you can unmount it:
dispatch(actions.unmount());
This spell will remove the current mount point, but it won’t erase the data. If you mount the same path again, your previous state will still be there!
Clearing the Crystal Ball
If you need to reset your mounted state to a blank slate, use the clear
action:
dispatch(actions.clear());
This will set the currently mounted state to an empty object, giving you a fresh start.
Multiple Mount Points
Redux-mount allows you to have multiple mount points active at once. Simply use different paths when mounting:
dispatch(actions.mount('user/profile', { name: 'Merlin' }));
dispatch(actions.mount('settings/theme', { dark: true }));
Each mount point will have its own isolated state, allowing you to manage different view-specific data simultaneously.
Conditional Mounting
You can create more complex mounting logic by combining redux-mount with your own custom actions:
const mountUserProfile = (userId) => (dispatch, getState) => {
const currentMount = mountSelector(getState());
if (currentMount.userId !== userId) {
dispatch(actions.mount(`user/${userId}`));
dispatch(fetchUserData(userId));
}
};
This spell will only mount a new user profile if it’s different from the currently mounted one, preventing unnecessary re-mounts and data fetches.
Conclusion
Redux-mount is a powerful wand in the React-Redux mage’s arsenal. By simplifying the management of view-specific state, it allows you to focus on crafting beautiful and functional user interfaces without getting tangled in the complexities of state management. Whether you’re building a small charm or a grand enchantment, redux-mount can help you manage your application’s state with the finesse of a true sorcerer. So go forth, and may your states be ever mounted and your views ever magical!